How to Fix Java Error java.lang.AbstractMethodError
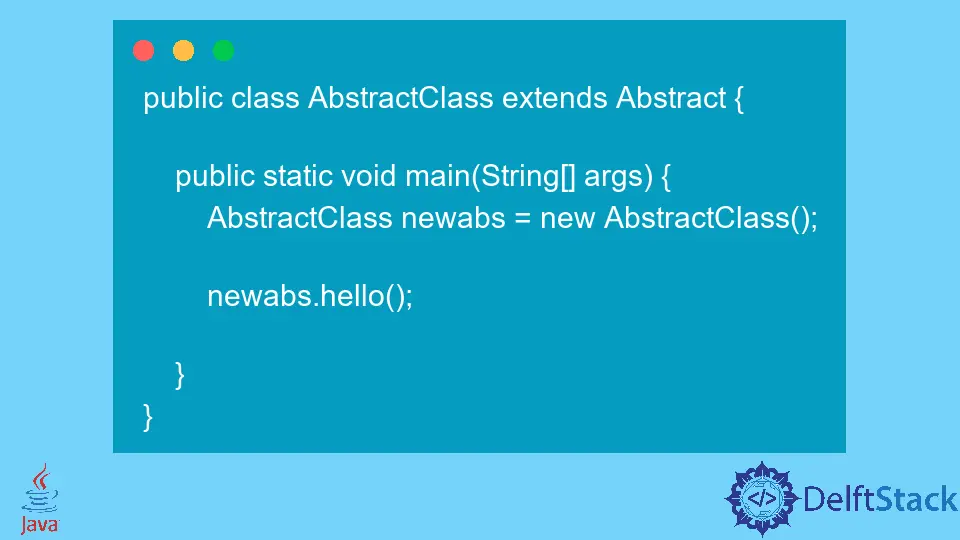
This article will help you understand the java.lang.AbstractMethodError
and how to solve them in Java.
Fix the java.lang.AbstractMethodError
Error in Java
Sometimes, our program may experience AbstractMethodError
at runtime. Finding the root of the issue could take some time if we are unfamiliar with this mistake.
In this lesson, we’ll examine AbstractMethodError
in more detail. We’ll learn what an AbstractMethodError
is and when one could occur.
When a program tries to make up an abstract method that hasn’t been implemented, the error AbstractMethodError
is raised. We know that the compiler will raise an error if any abstract processes are not implemented; the application won’t be created as a result.
We’ll construct an AbstractMethodError
example using the command-line javac compiler. This error is generated when the classes are independently compiled, which most IDEs forbid.
Make a Java class named Abstract
from the source code below:
Code (Abstract.java
):
public class Abstract {
public void hello() {
System.out.println("Hello! I belong to abstract class");
}
}
Follow these instructions on the command line to compile this class:
javac Abstract.java
Create the second class, named AbstractClass
, using the following source code once the first class has been compiled:
Code (AbstractClass.java
):
public class AbstractClass extends Abstract {
public static void main(String[] args) {
AbstractClass newabs = new AbstractClass();
newabs.hello();
}
}
This would result from assembling and executing as follows:
javac AbstractClass.java
java AbstractClass
Output:
Hello! I belong to abstract class
Even though everything is OK, what would happen if we changed the hello()
function to be abstract and then recompiled Abstract
without modifying AbstractClass
? Try it by changing the Abstract
to something like this:
public abstract class Abstract {
public abstract void hello();
}
We can now easily recompile this class; however, when we execute the AbstractClass
, we get the following:
Exception in thread "main" java.lang.AbstractMethodError: AbstractClass.hello()V
at AbstractClass.main(AbstractClass.java:6)
Suppose a base class method is changed to be abstract, modifying the base class as a result, and the necessary modifications are not made in the child class. In that case, AbstractMethodError
will occur (i.e., overriding the abstract methods).
Thus, just like in the example above, the programmer uses an abstract, unimplemented method (without being aware of its abstractness). Since only the base class is altered and compiled, he is unaware of this problem.
Implementing the Abstract
method in the AbstractClass
is all required in this situation. The AbstractMethodError
issue would be resolved by doing this.
public class AbstractClass extends Abstract {
public static void main(String[] args) {
AbstractClass newabs = new AbstractClass();
newabs.hello();
}
@Override
public void hello() {
System.out.println("Hello! I belong to abstract class");
}
}
Output:
Hello! I belong to abstract class
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack