Java getActionCommand() Method
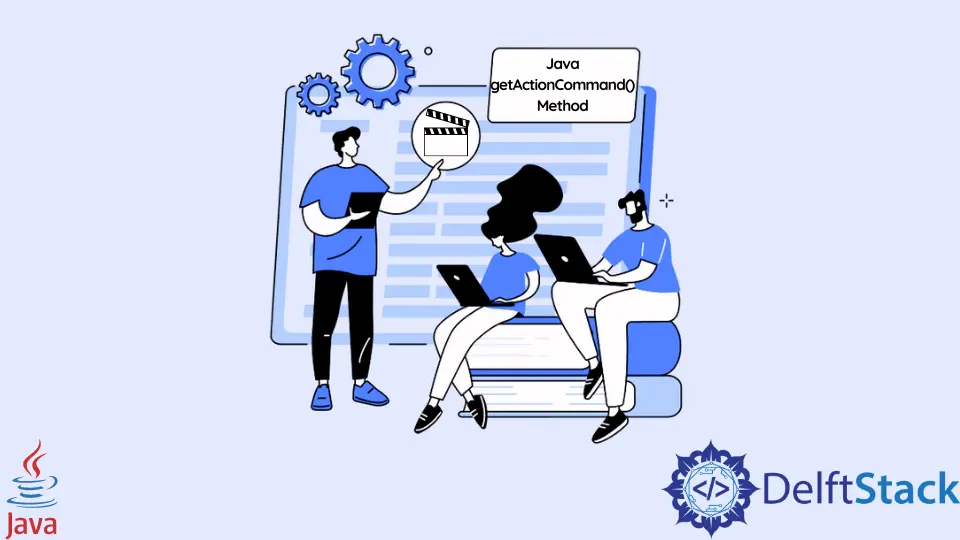
This tutorial demonstrates how to use getActionCommand()
method in Java.
Use the getActionCommand()
Method in Java
The getActionCommand()
method in Java is important for event handling in graphical user interfaces (GUIs). It is commonly used with components like buttons, checkboxes, and radio buttons.
This method allows developers to associate a unique command string with each GUI component, and when an action, such as a button click, occurs, getActionCommand()
retrieves this command string.
The significance lies in the ability to distinguish between different actions triggered by various components.
Instead of relying solely on the type of the component, the associated command string provides a more flexible and descriptive way to identify the action. This is particularly useful when multiple buttons or similar components exist in a GUI, enabling developers to handle each action uniquely based on the associated command.
In essence, getActionCommand()
enhances the precision of event handling, contributing to cleaner and more maintainable code in Java applications with graphical interfaces.
The getActionCommand()
method is commonly used with components in Java Swing and AWT for handling events. Here are some of the classes where getActionCommand()
method is typically used:
Class | Description |
---|---|
AbstractButton |
This includes buttons such as JButton , JCheckBox , JRadioButton , etc. The getActionCommand() method can be used to retrieve the action command associated with these buttons. |
ActionEvent |
In event handling, getActionCommand() is used to retrieve the command string from the ActionEvent object generated by a user action. |
When these components trigger events, developers often need a way to identify the specific action performed. The getActionCommand()
method becomes invaluable in such scenarios, allowing for the retrieval of an associated action command string.
This article will explore how to effectively use getActionCommand()
with JButton
, JCheckBox
, JRadioButton
, and ActionEvent
.
Let’s delve into a comprehensive example that illustrates the usage of getActionCommand()
:
import java.awt.event.*;
import javax.swing.*;
public class ActionCommandExample implements ActionListener {
JButton jButton;
JCheckBox jCheckBox;
JRadioButton jRadioButton;
public ActionCommandExample() {
// Creating a JFrame
JFrame frame = new JFrame("Action Command Example");
// Creating a JButton
jButton = new JButton("Click me");
jButton.setActionCommand("buttonCommand");
jButton.addActionListener(this);
// Creating a JCheckBox
jCheckBox = new JCheckBox("Check me");
jCheckBox.setActionCommand("checkboxCommand");
jCheckBox.addActionListener(this);
// Creating a JRadioButton
jRadioButton = new JRadioButton("Select me");
jRadioButton.setActionCommand("radioButtonCommand");
jRadioButton.addActionListener(this);
// Adding components to the frame
frame.setLayout(new BoxLayout(frame.getContentPane(), BoxLayout.Y_AXIS));
frame.add(jButton);
frame.add(jCheckBox);
frame.add(jRadioButton);
// Setting up the frame
frame.pack();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
// ActionListener implementation
public void actionPerformed(ActionEvent e) {
String command = e.getActionCommand();
// Handling button command
if (command.equals("buttonCommand")) {
System.out.println("Button clicked with command: " + command);
}
// Handling checkbox command
else if (command.equals("checkboxCommand")) {
System.out.println("Checkbox clicked with command: " + command);
}
// Handling radio button command
else if (command.equals("radioButtonCommand")) {
System.out.println("Radio button clicked with command: " + command);
}
}
public static void main(String[] args) {
new ActionCommandExample();
}
}
The code example follows a structured approach to demonstrate the usage of getActionCommand()
in Java.
It begins by importing the necessary classes from the javax.swing
and java.awt.event
packages. Following that, the ActionCommandExample
class is declared, highlighting its implementation of the ActionListener
interface.
Component initialization is the next step, where instances of JButton
, JCheckBox
, and JRadioButton
are created. Their action commands are set using the setActionCommand()
method, establishing a link between the components and their respective commands.
The frame setup involves creating a JFrame
and configuring its layout to be a BoxLayout
. This layout is chosen to arrange components vertically within the frame, providing a visually organized structure.
The actionPerformed
method is then implemented as part of the ActionListener
interface. Within this method, the getActionCommand()
method is employed to retrieve the action command associated with the event triggered by a user action.
Command handling is a crucial aspect of the example, where the action command is examined to identify which component initiated the action. Corresponding messages are then printed, providing clarity on the specific user interaction.
Finally, the main
method is introduced to instantiate an object of the ActionCommandExample
class, serving as the entry point for initiating the graphical user interface (GUI). This systematic and detailed explanation ensures a clear understanding of how getActionCommand()
is integrated into a Java program to enhance event handling within a user interface.
Output:
Upon running this program, a graphical user interface with a button, checkbox, and radio button is displayed. Clicking each component will produce output messages indicating the component and its associated action command.
Button clicked with command: buttonCommand
Button clicked with command: buttonCommand
Checkbox clicked with command: checkboxCommand
Radio button clicked with command: radioButtonCommand
This comprehensive example showcases how getActionCommand()
can be used with various Swing components to handle user actions effectively, making it a crucial tool for Java GUI development. Understanding and implementing this method enhances the ability to manage events in a user-friendly manner within Java applications.
Conclusion
The getActionCommand()
in Java is a crucial method for precise event handling in graphical user interfaces. By associating unique command strings with components like buttons, it enables developers to identify and respond to specific actions, enhancing code clarity and maintainability.
This method streamlines the process of handling diverse user interactions, contributing to the efficiency and flexibility of Java applications with graphical interfaces.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook