Método Java getActionCommand()
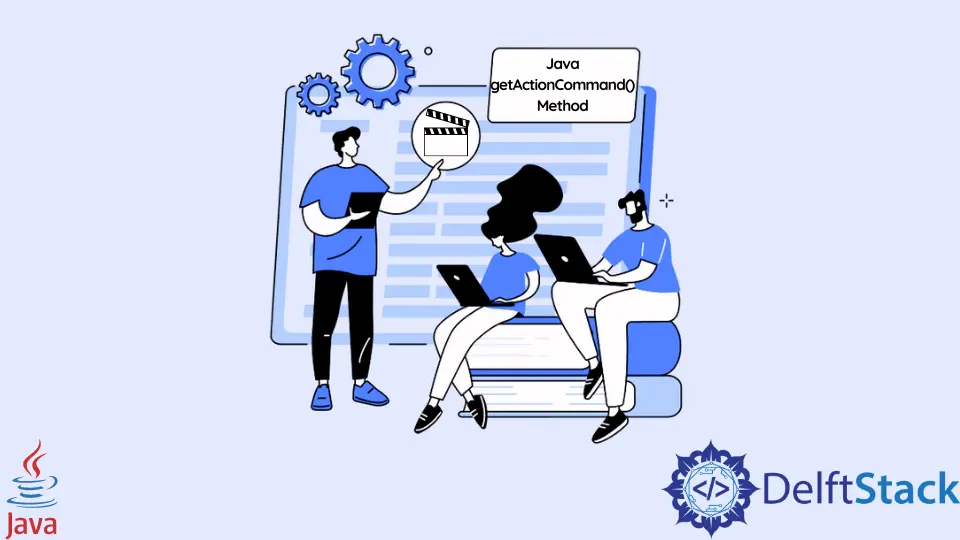
Este tutorial demuestra cómo usar el método getActionCommand()
en Java.
Utilice el método getActionCommand()
en Java
El método getActionCommand()
de la clase ActionListener
identifica un botón. Cuando hay varios botones, el getActionCommand()
nos brinda una manera fácil de saber qué botón se presionó.
El método getActionCommand()
nos dará una representación de cadena en el comando de acción. El valor será específico del componente que podemos establecer usando el método setActionCommmand()
.
Vamos a crear un ejemplo usando el método getActionCommand
. Primero, cree una clase que amplíe JFrame e implemente ActionListener
.
Luego, inicialice los botones privados y use el método constructor para establecer el título, el tamaño, los métodos setButtons()
, setActions()
, etc. Use el método setButtons()
para asignar nombres a los botones y agregarlos y usar el método setActions()
para establecer los comandos de acciones que se invocarán cuando se invoque getActionCommand
.
Ahora, use actionPerformed
para asignar acciones a los comandos anteriores. Estos comandos serán invocados usando el getActionCommand()
.
Por último, cree otra clase con el método principal y cree una instancia de la clase anterior para ejecutar la aplicación.
Código fuente completo:
package delftstack;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
class DemoFrame extends JFrame implements ActionListener {
private JButton J_Button1, J_Button2, J_Button3;
DemoFrame() {
setTitle("getActionCommand");
setLayout(new FlowLayout());
setButtons();
setAction();
setSize(700, 200);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private void setButtons() {
J_Button1 = new JButton("JButton One");
J_Button2 = new JButton("JButton Two");
J_Button3 = new JButton("JButton Three");
add(J_Button1);
add(J_Button2);
add(J_Button3);
}
private void setAction() {
J_Button1.addActionListener(this);
J_Button1.setActionCommand("J_Button1");
J_Button2.addActionListener(this);
J_Button2.setActionCommand("J_Button2");
J_Button3.addActionListener(this);
J_Button3.setActionCommand("J_Button3");
}
public void actionPerformed(ActionEvent event) {
if (event.getActionCommand() == "J_Button1")
JOptionPane.showMessageDialog(rootPane, "The JButton One is Pressed");
else if (event.getActionCommand() == "J_Button2")
JOptionPane.showMessageDialog(rootPane, "The JButton Two is Pressed");
else if (event.getActionCommand() == "J_Button3")
JOptionPane.showMessageDialog(rootPane, "The JButton Three is Pressed");
}
}
public class Example {
public static void main(String[] args) {
DemoFrame Demo_Frame = new DemoFrame();
}
}
El código anterior creará tres botones y establecerá comandos de acción para ellos. Cuando pulsamos un botón, se invocará el getActionCommand()
, y el método showMessageDialog()
mostrará el mensaje correspondiente.
Producción:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook