Java getActionCommand() メソッド
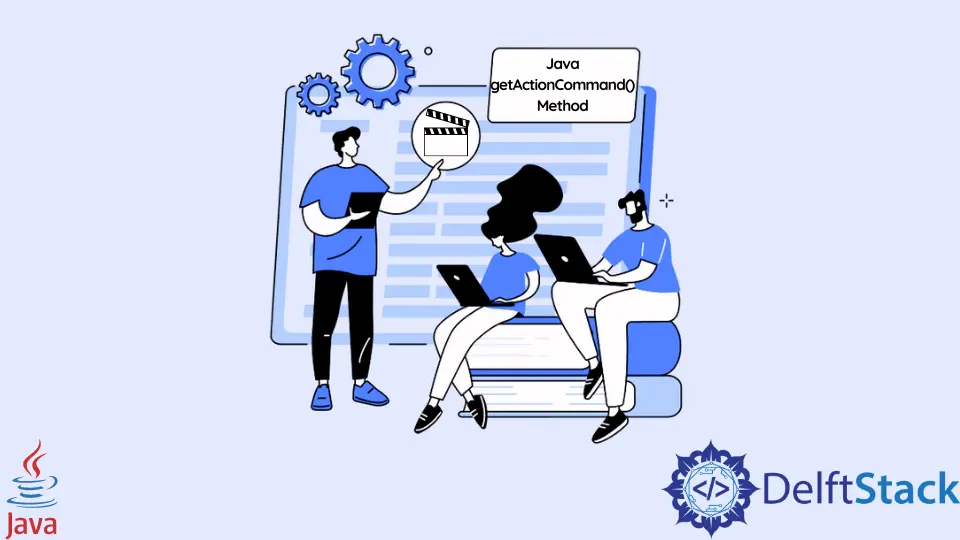
このチュートリアルでは、Java で getActionCommand()
メソッドを使用する方法を示します。
Java で getActionCommand()
メソッドを使用する
ActionListener
クラスの getActionCommand()
メソッドは、ボタンを識別します。 複数のボタンがある場合、getActionCommand()
を使用すると、どのボタンが押されたかを簡単に知ることができます。
getActionCommand()
メソッドは、アクション コマンドの文字列表現を提供します。 値はコンポーネント固有で、setActionCommmand()
メソッドを使用して設定できます。
getActionCommand
メソッドを使用して例を作成しましょう。 まず、JFrame を拡張して ActionListener
を実装するクラスを作成します。
次に、プライベート ボタンを初期化し、コンストラクター メソッドを使用してタイトル、サイズ、setButtons()
、setActions()
メソッドなどを設定します。setButtons()
メソッドを使用してボタンに名前を割り当て、それらを追加して使用します。 setActions()
メソッドは、getActionCommand
が呼び出されたときに呼び出されるアクション コマンドを設定します。
ここで、actionPerformed
を使用して、上記のコマンドにアクションを割り当てます。 これらのコマンドは、getActionCommand()
を使用して呼び出されます。
最後に、main メソッドを使用して別のクラスを作成し、上記のクラスのインスタンスを作成してアプリケーションを実行します。
完全なソース コード:
package delftstack;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
class DemoFrame extends JFrame implements ActionListener {
private JButton J_Button1, J_Button2, J_Button3;
DemoFrame() {
setTitle("getActionCommand");
setLayout(new FlowLayout());
setButtons();
setAction();
setSize(700, 200);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private void setButtons() {
J_Button1 = new JButton("JButton One");
J_Button2 = new JButton("JButton Two");
J_Button3 = new JButton("JButton Three");
add(J_Button1);
add(J_Button2);
add(J_Button3);
}
private void setAction() {
J_Button1.addActionListener(this);
J_Button1.setActionCommand("J_Button1");
J_Button2.addActionListener(this);
J_Button2.setActionCommand("J_Button2");
J_Button3.addActionListener(this);
J_Button3.setActionCommand("J_Button3");
}
public void actionPerformed(ActionEvent event) {
if (event.getActionCommand() == "J_Button1")
JOptionPane.showMessageDialog(rootPane, "The JButton One is Pressed");
else if (event.getActionCommand() == "J_Button2")
JOptionPane.showMessageDialog(rootPane, "The JButton Two is Pressed");
else if (event.getActionCommand() == "J_Button3")
JOptionPane.showMessageDialog(rootPane, "The JButton Three is Pressed");
}
}
public class Example {
public static void main(String[] args) {
DemoFrame Demo_Frame = new DemoFrame();
}
}
上記のコードは、3つのボタンを作成し、それらのアクション コマンドを設定します。 ボタンを押すと、getActionCommand()
が呼び出され、メソッド showMessageDialog()
が対応するメッセージを表示します。
出力:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook