How to Get JSON From URL in Java
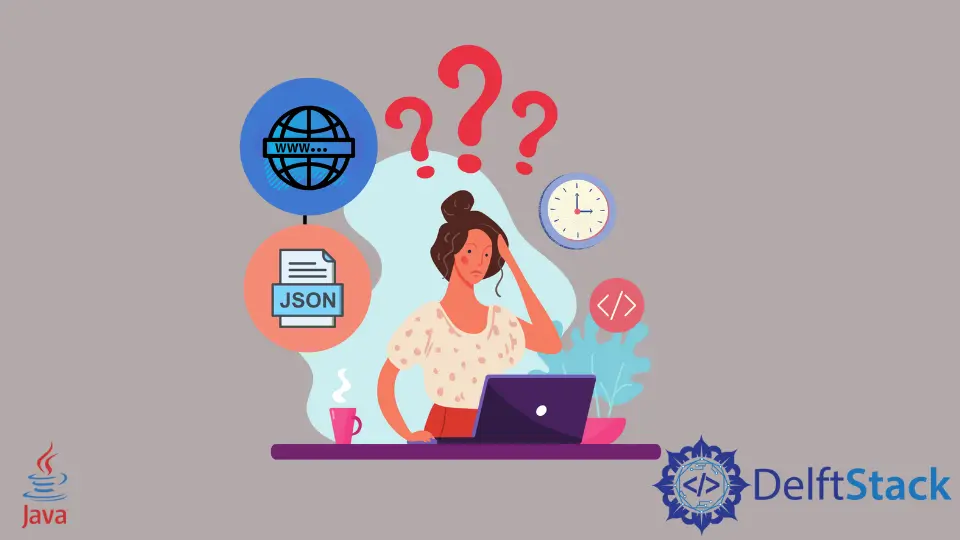
In this guide, we will learn how to get JSON from URL in Java. The URLs are APIs containing data that you can convert to JSON for further use. We are assuming you are already familiar with the basic concepts of JSON in Java.
Get JSON From URL in Java
There are countless JSON URL samples available online. After reading this guide, you can also visit here and test any JSON URL. We will read the data stored in such APIs and present them in our output in JSON format. For instance, if we open this example URL (http://ip.jsontest.com/), it will open a webpage with the following output in JSON format.
Similarly, if we take this example URL (http://headers.jsontest.com/), the output would be as follows.
Now, let’s see how we can get the same JSON format from the URL. We’ll take the same two examples as above.
Code example:
import java.io.*;
import java.net.*;
import java.nio.charset.*;
import org.json.*;
public static void main(String[] args) throws IOException, JSONException {
String url = "http://ip.jsontest.com/"; // example url which return json data
ReadJson reader = new ReadJson(); // To ReadJson in order to read from url.
JSONObject json = reader.readJsonFromUrl(url); // calling method in order to read.
System.out.println(json.toString()); // simple for printing.
}
In the code example above, inside the main function, we store a URL inside a string. To read data from the URL, we created an object reader. We called the method readJsonFromUrl and integrated it with the object reader. Let’s see where the magic happens.
Inside readJsonFromUrl
public JSONObject readJsonFromUrl(String link) throws IOException, JSONException {
InputStream input = new URL(link).openStream();
// Input Stream Object To Start Streaming.
try { // try catch for checked exception
BufferedReader re = new BufferedReader(new InputStreamReader(input, Charset.forName("UTF-8")));
// Buffer Reading In UTF-8
String Text = Read(re); // Handy Method To Read Data From BufferReader
JSONObject json = new JSONObject(Text); // Creating A JSON
return json; // Returning JSON
} catch (Exception e) {
return null;
} finally {
input.close();
}
}
In the above function, the link is assigned to input which will start the streaming process. To read the text from the character-input stream, we need to buffer the characters for efficient reading. Learn more about Buffers here. The above example will buffer in UTF-8 format. To read data from BufferReader, we created another public function, Read
.
Inside the Function Read
public String Read(Reader re) throws IOException { // class Declaration
StringBuilder str = new StringBuilder(); // To Store Url Data In String.
int temp;
do {
temp = re.read(); // reading Charcter By Chracter.
str.append((char) temp);
} while (temp != -1);
// re.read() return -1 when there is end of buffer , data or end of file.
return str.toString();
}
Inside Read, we are simply storing URL data inside a string using the do...while
loop. We are reading character by character and storing each of them inside temp. Using typecasting, we have all the data in characters inside str
. str
as a string stored inside Text
in function readJsonFromUrl
is returned. We create a JSON using JSONObject
and return it.
To run the above program, we need to use the following command.
javac -cp 'org.json.jar' ReadJson.java
java -cp 'org.json.jar' ReadJson.java
The output for the example URL (http://ip.jsontest.com/) is as follows.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn