在 Java 中从 URL 获取 Json
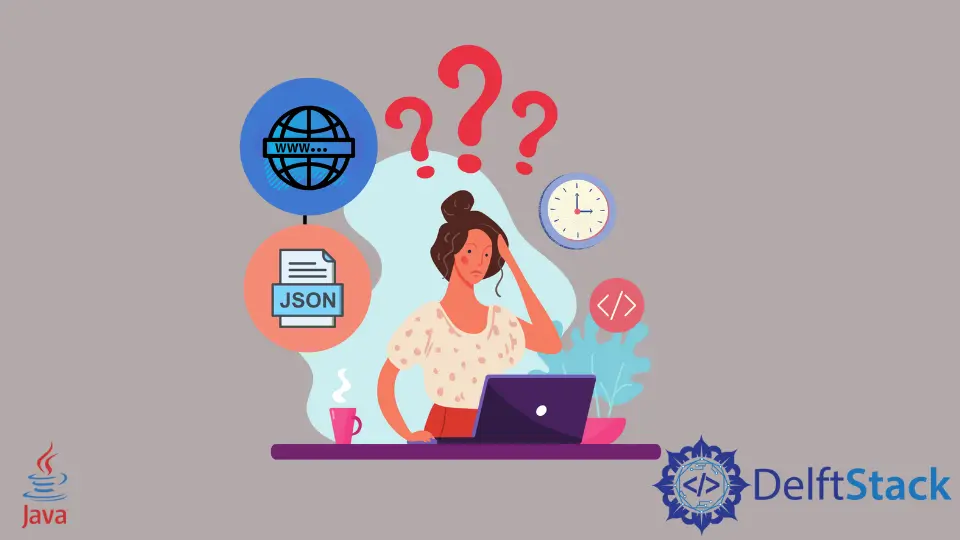
在本指南中,我们将学习如何在 Java 中从 URL 获取 JSON。URL 是包含数据的 API,你可以将这些数据转换为 JSON 以供进一步使用。我们假设你已经熟悉 Java 中 JSON 的基本概念。
在 Java 中从 URL 获取 JSON
网上有无数的 JSON URL 示例。阅读本指南后,你还可以访问此处并测试任何 JSON URL。我们将读取存储在此类 API 中的数据,并将它们以 JSON 格式呈现在我们的输出中。例如,如果我们打开这个示例 URL (http://ip.jsontest.com/),它会打开一个网页,其中包含以下 JSON 格式的输出。
同样,如果我们使用这个示例 URL (http://headers.jsontest.com/),输出将如下所示。
现在,让我们看看如何从 URL 中获取相同的 JSON 格式。我们将采用与上面相同的两个示例。
代码示例:
import java.io.*;
import java.net.*;
import java.nio.charset.*;
import org.json.*;
public static void main(String[] args) throws IOException, JSONException {
String url = "http://ip.jsontest.com/"; // example url which return json data
ReadJson reader = new ReadJson(); // To ReadJson in order to read from url.
JSONObject json = reader.readJsonFromUrl(url); // calling method in order to read.
System.out.println(json.toString()); // simple for printing.
}
在上面的代码示例中,在 main 函数中,我们将 URL 存储在字符串中。为了从 URL 读取数据,我们创建了一个对象读取器。我们调用了 readJsonFromUrl 方法并将其与对象读取器集成。让我们看看魔法发生在哪里。
在 readJsonFromUrl
里面
public JSONObject readJsonFromUrl(String link) throws IOException, JSONException {
InputStream input = new URL(link).openStream();
// Input Stream Object To Start Streaming.
try { // try catch for checked exception
BufferedReader re = new BufferedReader(new InputStreamReader(input, Charset.forName("UTF-8")));
// Buffer Reading In UTF-8
String Text = Read(re); // Handy Method To Read Data From BufferReader
JSONObject json = new JSONObject(Text); // Creating A JSON
return json; // Returning JSON
} catch (Exception e) {
return null;
} finally {
input.close();
}
}
在上面的函数中,链接被分配给将启动流处理的输入。为了从字符输入流中读取文本,我们需要缓冲字符以进行高效读取。了解有关缓冲区的更多信息此处。上面的示例将以 UTF-8 格式进行缓冲。为了从 BufferReader 读取数据,我们创建了另一个公共函数 Read。
函数内部 Read
public String Read(Reader re) throws IOException { // class Declaration
StringBuilder str = new StringBuilder(); // To Store Url Data In String.
int temp;
do {
temp = re.read(); // reading Charcter By Chracter.
str.append((char) temp);
} while (temp != -1);
// re.read() return -1 when there is end of buffer , data or end of file.
return str.toString();
}
在 Read 中,我们只是使用 do...while
循环将 URL 数据存储在字符串中。我们正在逐个读取字符并将它们存储在 temp 中。使用类型转换,我们将所有数据都放在 str
中的字符中。str
作为存储在 Text
函数 readJsonFromUrl
中的字符串被返回。我们使用 JSONObject
创建一个 JSON 并返回它。
要运行上述程序,我们需要使用以下命令。
javac -cp 'org.json.jar' ReadJson.java
java -cp 'org.json.jar' ReadJson.java
示例 URL (http://ip.jsontest.com/) 的输出如下。
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn