Java의 URL에서 Json 가져오기
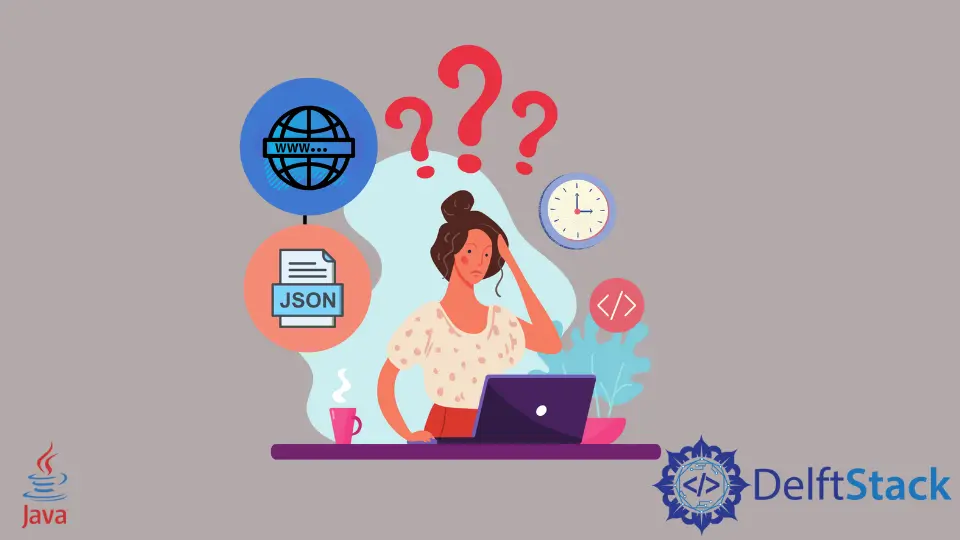
이 가이드에서는 Java의 URL에서 JSON을 가져오는 방법을 배웁니다. URL은 추가 사용을 위해 JSON으로 변환할 수 있는 데이터가 포함된 API입니다. Java에서 JSON의 기본 개념에 이미 익숙하다고 가정합니다.
Java의 URL에서 JSON 가져오기
온라인에는 수많은 JSON URL 샘플이 있습니다. 이 가이드를 읽은 후 여기를 방문하여 JSON URL을 테스트할 수도 있습니다. 이러한 API에 저장된 데이터를 읽고 JSON 형식으로 출력에 표시합니다. 예를 들어 이 예제 URL(http://ip.jsontest.com/)을 열면 JSON 형식의 다음 출력이 포함된 웹 페이지가 열립니다.
마찬가지로 이 예제 URL(http://headers.jsontest.com/)을 사용하면 출력은 다음과 같습니다.
이제 URL에서 동일한 JSON 형식을 가져오는 방법을 살펴보겠습니다. 위와 같은 두 가지 예를 들어보겠습니다.
코드 예:
import java.io.*;
import java.net.*;
import java.nio.charset.*;
import org.json.*;
public static void main(String[] args) throws IOException, JSONException {
String url = "http://ip.jsontest.com/"; // example url which return json data
ReadJson reader = new ReadJson(); // To ReadJson in order to read from url.
JSONObject json = reader.readJsonFromUrl(url); // calling method in order to read.
System.out.println(json.toString()); // simple for printing.
}
위의 코드 예제에서 main 함수 내에서 문자열 안에 URL을 저장합니다. URL에서 데이터를 읽기 위해 객체 판독기를 만들었습니다. readJsonFromUrl 메서드를 호출하고 이를 개체 판독기와 통합했습니다. 마법이 일어나는 곳을 봅시다.
readJsonFromUrl
내부
public JSONObject readJsonFromUrl(String link) throws IOException, JSONException {
InputStream input = new URL(link).openStream();
// Input Stream Object To Start Streaming.
try { // try catch for checked exception
BufferedReader re = new BufferedReader(new InputStreamReader(input, Charset.forName("UTF-8")));
// Buffer Reading In UTF-8
String Text = Read(re); // Handy Method To Read Data From BufferReader
JSONObject json = new JSONObject(Text); // Creating A JSON
return json; // Returning JSON
} catch (Exception e) {
return null;
} finally {
input.close();
}
}
위의 기능에서 스트리밍 프로세스를 시작할 입력에 링크가 할당됩니다. 문자 입력 스트림에서 텍스트를 읽으려면 효율적인 읽기를 위해 문자를 버퍼링해야 합니다. 여기 버퍼에 대해 자세히 알아보세요. 위의 예는 UTF-8 형식으로 버퍼링됩니다. BufferReader에서 데이터를 읽기 위해 또 다른 공용 함수인 Read
를 만들었습니다.
Read
기능 내부
public String Read(Reader re) throws IOException { // class Declaration
StringBuilder str = new StringBuilder(); // To Store Url Data In String.
int temp;
do {
temp = re.read(); // reading Charcter By Chracter.
str.append((char) temp);
} while (temp != -1);
// re.read() return -1 when there is end of buffer , data or end of file.
return str.toString();
}
Read 내부에서 do...while
루프를 사용하여 문자열 내부에 URL 데이터를 저장합니다. 우리는 문자를 문자로 읽고 각각을 temp에 저장합니다. 우리는 타이페캐스팅을 사용하여 str
안에 문자의 모든 데이터가 있습니다. str
은 readJsonFromUrl
함수의 Text
안에 저장된 문자열로 반환됩니다. JSONObject
를 사용하여 JSON을 생성하고 반환합니다.
위의 프로그램을 실행하려면 다음 명령을 사용해야 합니다.
javac -cp 'org.json.jar' ReadJson.java
java -cp 'org.json.jar' ReadJson.java
예시 URL(http://ip.jsontest.com/)의 출력은 다음과 같습니다.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn