How to Format Specifiers for Integral Numbers in Java
- What Is a Format Specifier in Java
- Format Specifiers for Integral Numbers in Java
-
the Use of
%d
in Java -
the Use of
%o
and%x
in Java
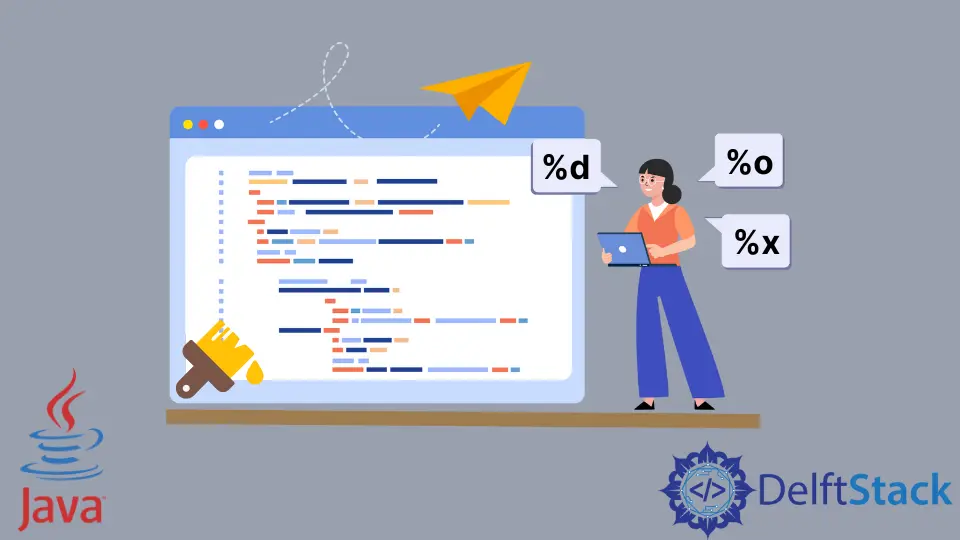
This tutorial speaks about the format specifiers for integral numbers in Java. We’ll see the format specifiers and use them for integral numbers to display output on the screen.
What Is a Format Specifier in Java
The format specifiers tell which type of data would be printed on the screen, also used for taking input from the user. These format specifiers start with a percentage symbol (%
) followed by a type character indicating the data’s type, for instance, int
, string
, float
etc.
Format Specifiers for Integral Numbers in Java
We can format the values of short
, Short
, byte
, Byte
, BigInteger
, long
, and Long
. We can format the whole number using an integral number’s format specifiers; we also have various flags that we can use with them.
-
d
- used to format an argument as the decimal integer; we can’t use the#
flag with this. -
o
- used to format an argument as the base-8 integer, we can’t use'(', '+', ' ', ','
flags with this. The output will start with zero (0
) if we use the#
flag. -
x
orX
- used to format an argument as the base-16 integer. The output starts with0x
if we usex
with the#
flag.By using
X
with the#
flag, the output would be0X
. We can’t use'(', '+', ' ', ','
flags if the argument’s data type isint
/Integer
,long
/Long
,short
/Short
, orbyte
/Byte
.If the argument’s data type is
BigInteger
, we cannot use the,
flag.
Remember, the precision
is not applicable in a format specifier for integral numbers.
the Use of %d
in Java
Example Code:
System.out.printf("'%d' %n", 5678);
Output:
'5678'
Here, the printf()
statement prints the number and formats it according to the format specifier as specified. The %d
represents an integer number, and %n
is used for printing a new line.
Example Code:
System.out.printf("'%6d' %n", 5678);
System.out.printf("'%6d' %n", 25695678);
Output:
' 5678'
'25695678'
The %6d
represents the minimum number of characters that need to be printed on the screen. If the number is less than the 6-digit, it would be padded with white spaces.
See the output above to get the number as ' 5678'
because it is not a 6-digit number and is padded with white spaces. The output will not be truncated if it is even larger than 6-digits (see the second printf()
statement and its respective output in the above code).
Example Code:
System.out.printf("'%-6d' %n", 5678);
System.out.printf("'%-6d' %n", 25695678);
Output:
'5678 '
'25695678'
The %-6d
is the same concept as we learned for %6d
in the previous example, but the number will be padded with white spaces on the right (if it is less than 6-digits). The result will not be truncated here if it is a larger number.
Are you looking for a solution to add zeros instead of white spaces? See the following example.
Example Code:
System.out.printf("'%06d' %n", 5678);
Output:
'005678'
The result is padded with zeros instead of white spaces using %06d
. Remember, we can only add zeros on the left by using the 0
flag.
Example Code:
System.out.printf("'%(d' %n", 5678);
System.out.printf("'%(d' %n", -5678);
Output:
'5678'
'(5678)'
Here, we are using the (
flag with %d
as %(d
, which encloses the number within ()
if it is a negative number, otherwise, the result will not be affected. See the above code example where we use the (
flag with positive and negative numbers.
Example Code:
System.out.printf("'% d' %n", 5678);
System.out.printf("'% d' %n", -5678);
Output:
' 5678'
'-5678'
Using the % d
will add a leading space to the result but only for positive numbers. See the output given above.
Are you looking for a solution to print the positive numbers with the +
and negative numbers with the -
sign? See the following code.
Example Code:
System.out.printf("'%+d' %n", 5678);
System.out.printf("'%+d' %n", -5678);
Output:
'+5678'
'-5678'
Here, %+d
helps us add the +
sign if the number is positive and -
if the number is negative.
the Use of %o
and %x
in Java
If we use x
and o
with the negative number whose data type is int
/Integer
, short
/Short
or Long
/long
, then the value (argument value) will be converted to the unsigned number first by adding a number 2N
to it (N
shows the bits’ number).
Remember, the BigInteger
arguments are not affected by these conversions. See the instance given below.
Example Code:
byte b1 = 1;
byte b2 = -3;
System.out.printf("%o\n", b1);
System.out.printf("%o", b2);
Output:
1
375
Take a look at another example code given below.
System.out.printf("%#o %n", 5789);
System.out.printf("%#x %n", 5789);
System.out.printf("%#X %n", 5789);
Output:
013235
0x169d
0X169D
Don’t worry about this output; let’s learn all printf()
statements sequentially. Here, %o
is for base-8 integer (also called octal value), and %x
or %X
is for base-16 integer (hexadecimal value).
The output starts with 0
, 0x
, and 0X
if the #
flag is used with %o
, %x
and %X
, respectively. Check the following screenshot explaining how the output is transformed.