How to Format Specifiers for Floating-Point Numbers in Java
- Format Specifier and Its Importance in Java
- Format Specifiers for Floating-Point Numbers in Java
- Format Floating-Point With/Without Precision in Java
-
Use the
format()
Method ofFormatter
Class to Format Floating-Point Numbers in Java -
Use the
format()
Method ofDecimalFormat
Class to Format Floating-Point Numbers in Java
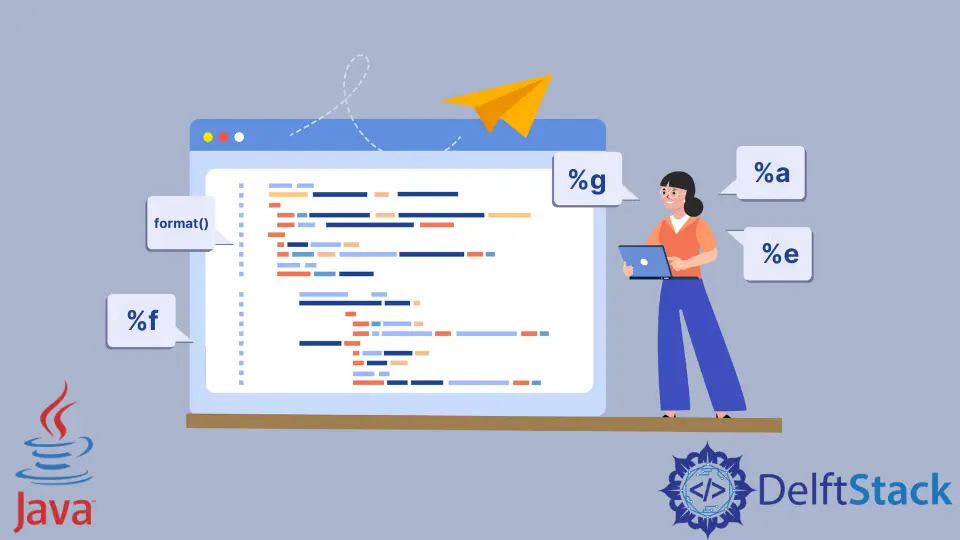
This tutorial tells about the format specifiers for floating-point numbers in Java. It also explains the format specifiers and how we can take assistance from them to format the floating-point numbers and display the output on the screen according to our expectations.
Format Specifier and Its Importance in Java
The format specifiers speak about which data type will be printed on the computer screen, helps to format the output and display the results we want.
Different format specifiers are used in formatting various data types’ values. For instance, the conversion character s
formats the strings, d
formats the decimal integers, f
can format the floating-point numbers and more.
We can also use them to take input from the user. Every format specifier begins with the percentage (%
) symbol; a type character follows it.
For example, %d
for int
, %f
for float
, etc.
Format Specifiers for Floating-Point Numbers in Java
The format specifier for floating-point numbers deals with a number’s fractional and integral parts. We can format the values of double
, Double
, float
, Float
, and BigDecimal
data types using floating-point number format specifiers.
Some of the conversion characters are listed below that we can use to format the floating-point numbers.
-
The
f
formats an argument in the locale-specific decimal format. Here, the precision means the number of digits after the decimal separator and the value is also rounded according to the precision. -
The
a
orA
is used to format in the form of hexadecimal exponential and can’t be used with theBigDecimal
type. -
The
e
orE
is used to format in a locale-specific scientific notation. After formatting, the output contains one digit followed by a decimal separator and exponent part.The precision decides how many digits will be printed after a decimal separator. We cannot use the
','
flag with this type of conversion. -
G
org
can format the value in the locale-specific general scientific notation. The formatting can act likef
ore
conversions here.How? See the following two rules.
4.1 The value will be formatted using
f
conversion, if the value after rounding meets the condition 10-4 =< value after rounding < 10precision.
4.2 On the other hand, it usese
conversion if the value after rounding satisfies the condition 10precision =< value after rounding < 10 -4.
The precision denotes the digits’ number after a decimal separator using e
or f
conversion character.
We can’t use precision while using the a
conversion character. For g
, the precision represents the digit’s total number in the resulting magnitude that we get after rounding.
Format Floating-Point With/Without Precision in Java
Example Code (using default precision):
public class Main {
public static void main(String[] args) {
System.out.printf("%e %n", 11.1);
System.out.printf("%f %n", 11.1);
System.out.printf("%g %n", 11.1);
System.out.printf("%e %n", 0.000005678);
System.out.printf("%f %n", 0.000005678);
System.out.printf("%g %n", 0.000005678);
System.out.printf("%a %n", 0.000005678);
}
}
Output:
1.110000e+01
11.100000
11.1000
5.678000e-06
0.000006
5.67800e-06
0x1.7d0b4c170d6b5p-18
Example Code (using precision and width):
// precision is 2
System.out.printf("%.2e %n", 987654.321);
System.out.printf("%.2f %n", 987654.321);
System.out.printf("%.2g %n", 987654.321);
// width is 8 and precision is 2
System.out.printf("'%8.2e' %n", 987654.321);
System.out.printf("'%8.2f' %n", 987654.321);
System.out.printf("'%8.2g' %n", 987654.321);
Output:
9.88e+05
987654.32
9.9e+05
'9.88e+05'
'987654.32'
' 9.9e+05'
Example Code (if floating-point is NaN
or infinity
):
System.out.printf("%.2e %n", Double.NaN);
System.out.printf("%.2f %n", Double.POSITIVE_INFINITY);
System.out.printf("%.2g %n", Double.NEGATIVE_INFINITY);
// the ( flag encloses the -ve number inside the () parenthsis
System.out.printf("%(f %n", Double.POSITIVE_INFINITY);
System.out.printf("%(f %n", Double.NEGATIVE_INFINITY);
Output:
NaN
Infinity
-Infinity
Infinity
(Infinity)
Use the format()
Method of Formatter
Class to Format Floating-Point Numbers in Java
import java.util.Date;
import java.util.Formatter;
import java.util.Locale;
public class FormatFloatingPointNumb {
public static void main(String args[]) {
Formatter formatter = new Formatter();
Date date = new Date();
// print the day
System.out.println(formatter.format(Locale.US, "In the US: %tA %n", date));
}
}
Output:
In the US: Sunday
We use the Java Formatter
, a utility class that helps us work with formatting stream output using Java programming. Here, format()
method has same syntax as printf()
.
The reason to use the format()
method for the Formatter
class is that we can do locale-specific formatting whenever required (see the code example above).
Use the format()
Method of DecimalFormat
Class to Format Floating-Point Numbers in Java
import java.text.DecimalFormat;
import java.util.Formatter;
public class FormatFloatingPointNumb {
public static void main(String args[]) {
DecimalFormat df = new DecimalFormat("#.##");
String formatted = df.format(2.4876);
System.out.println(formatted);
}
}
Output:
2.49
Another way to code the above example is below.
import java.text.DecimalFormat;
import java.util.Formatter;
public class FormatFloatingPointNumb {
public static void main(String args[]) {
DecimalFormat df = new DecimalFormat();
df.setMaximumFractionDigits(2);
System.out.println(df.format(2.4876));
}
}
Output:
2.49
The DecimalFormat
is the NumberFormat
’s concrete sub-class. It allows us to format the integers (234), scientific notation (1.65E5), currency ($456), percentage (25%), and fixed-point numbers (12.3) in any locale.
If we pass #.##
to the constructor of DecimalFormat
, it decides the digit’s number after decimal separator depending on how many #
we have after the decimal separator.
The other way is to create an object of DecimalFormat
. Then, call setMaximumFractionDigits()
by passing a number to tell the number of digits you want after the decimal separator.