Java 中浮点数的格式说明符
- 在 Java 中格式说明符及其的重要性
- Java 中浮点数的格式说明符
- 在 Java 中格式化带/不带精度的浮点数
-
在 Java 中使用
Formatter
类的format()
方法格式化浮点数 -
在 Java 中使用
DecimalFormat
类的format()
方法格式化浮点数
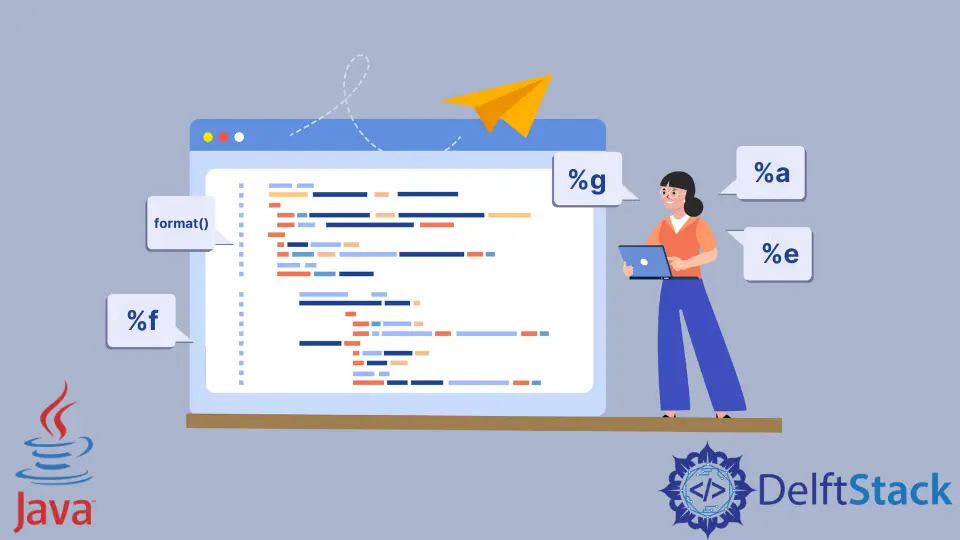
本教程介绍 Java 中浮点数的格式说明符。它还解释了格式说明符以及我们如何从它们那里获得帮助来格式化浮点数并根据我们的期望在屏幕上显示输出。
在 Java 中格式说明符及其的重要性
格式说明符说明将在计算机屏幕上打印哪种数据类型,有助于格式化输出并显示我们想要的结果。
不同的格式说明符用于格式化各种数据类型的值。例如,转换字符 s
格式化字符串,d
格式化十进制整数,f
可以格式化浮点数等等。
我们还可以使用它们来获取用户的输入。每个格式说明符都以百分比 (%
) 符号开头;一个类型字符跟随它。
例如,%d
表示 int
,%f
表示 float
,等等。
Java 中浮点数的格式说明符
浮点数的格式说明符处理数字的小数部分和整数部分。我们可以使用浮点数格式说明符格式化 double
、Double
、float
、Float
和 BigDecimal
数据类型的值。
下面列出了一些转换字符,我们可以使用它们来格式化浮点数。
-
f
以特定于语言环境的十进制格式格式化参数。这里的精度是指小数点分隔符后的位数,该值也根据精度四舍五入。 -
a
或A
用于格式化十六进制指数形式,不能与BigDecimal
类型一起使用。 -
e
或E
用于格式化特定于语言环境的科学记数法。格式化后,输出包含一位数字,后跟小数分隔符和指数部分。精度决定在小数点分隔符后打印多少位。我们不能在这种类型的转换中使用
','
标志。 -
G
或g
可以用特定于语言环境的通用科学记数法格式化值。格式在这里可以像f
或e
转换。怎么做?请看下面两条规则。
4.1 如果舍入后的值满足条件 10-4 =< 舍入后的值< 10precision,则使用
f
转换对值进行格式化。
4.2 另一方面,如果舍入后的值满足条件 10precision =< 舍入后的值 < 10-4,则使用e
转换。
精度表示使用 e
或 f
转换字符的小数分隔符后的位数。
使用 a
转换字符时不能使用精度。对于 g
,精度表示我们在舍入后得到的结果幅度中的数字总数。
在 Java 中格式化带/不带精度的浮点数
示例代码(使用默认精度):
public class Main {
public static void main(String[] args) {
System.out.printf("%e %n", 11.1);
System.out.printf("%f %n", 11.1);
System.out.printf("%g %n", 11.1);
System.out.printf("%e %n", 0.000005678);
System.out.printf("%f %n", 0.000005678);
System.out.printf("%g %n", 0.000005678);
System.out.printf("%a %n", 0.000005678);
}
}
输出:
1.110000e+01
11.100000
11.1000
5.678000e-06
0.000006
5.67800e-06
0x1.7d0b4c170d6b5p-18
示例代码(使用精度和宽度):
// precision is 2
System.out.printf("%.2e %n", 987654.321);
System.out.printf("%.2f %n", 987654.321);
System.out.printf("%.2g %n", 987654.321);
// width is 8 and precision is 2
System.out.printf("'%8.2e' %n", 987654.321);
System.out.printf("'%8.2f' %n", 987654.321);
System.out.printf("'%8.2g' %n", 987654.321);
输出:
9.88e+05
987654.32
9.9e+05
'9.88e+05'
'987654.32'
' 9.9e+05'
示例代码(如果浮点数是 NaN
或 infinity
):
System.out.printf("%.2e %n", Double.NaN);
System.out.printf("%.2f %n", Double.POSITIVE_INFINITY);
System.out.printf("%.2g %n", Double.NEGATIVE_INFINITY);
// the ( flag encloses the -ve number inside the () parenthsis
System.out.printf("%(f %n", Double.POSITIVE_INFINITY);
System.out.printf("%(f %n", Double.NEGATIVE_INFINITY);
输出:
NaN
Infinity
-Infinity
Infinity
(Infinity)
在 Java 中使用 Formatter
类的 format()
方法格式化浮点数
import java.util.Date;
import java.util.Formatter;
import java.util.Locale;
public class FormatFloatingPointNumb {
public static void main(String args[]) {
Formatter formatter = new Formatter();
Date date = new Date();
// print the day
System.out.println(formatter.format(Locale.US, "In the US: %tA %n", date));
}
}
输出:
In the US: Sunday
我们使用 Java Formatter
,这是一个实用程序类,可帮助我们使用 Java 编程来格式化流输出。这里,format()
方法的语法与 printf()
相同。
对 Formatter
类使用 format()
方法的原因是,我们可以在需要时进行特定于语言环境的格式化(参见上面的代码示例)。
在 Java 中使用 DecimalFormat
类的 format()
方法格式化浮点数
import java.text.DecimalFormat;
import java.util.Formatter;
public class FormatFloatingPointNumb {
public static void main(String args[]) {
DecimalFormat df = new DecimalFormat("#.##");
String formatted = df.format(2.4876);
System.out.println(formatted);
}
}
输出:
2.49
下面是对上述示例进行编码的另一种方法。
import java.text.DecimalFormat;
import java.util.Formatter;
public class FormatFloatingPointNumb {
public static void main(String args[]) {
DecimalFormat df = new DecimalFormat();
df.setMaximumFractionDigits(2);
System.out.println(df.format(2.4876));
}
}
输出:
2.49
DecimalFormat
是 NumberFormat
的具体子类。它允许我们在任何语言环境中格式化整数 (234)、科学记数法 (1.65E5)、货币 ($456)、百分比 (25%) 和定点数 (12.3)。
如果我们将 #.##
传递给 DecimalFormat
的构造函数,它会根据小数分隔符后有多少 #
来决定小数分隔符后的数字。
另一种方法是创建一个 DecimalFormat
的对象。然后,通过传递一个数字来调用 setMaximumFractionDigits()
,以告知你想要的小数分隔符后的位数。