Lambda Comparator in Java
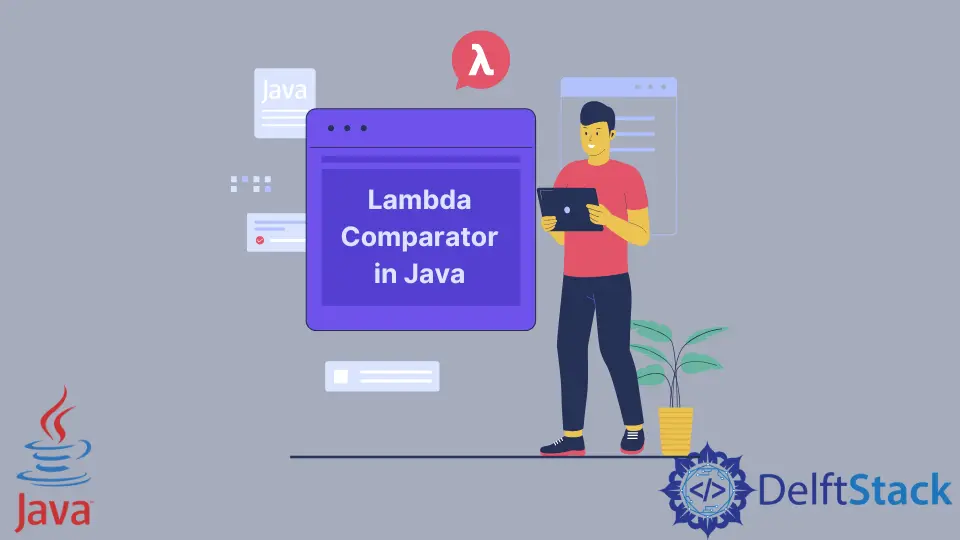
This guide will tackle the lambda comparators in the Java programming language.
We need to understand Lambda’s basic understanding to understand the comparator function. Let’s dive into this guide.
Lambda Comparator in Java
A lambda expression is a code block accepting parameters and returns a value.
Lambda expressions are similar to methods. However, they do not need a name like methods, and they can be implemented right in the body of a method.
Lambda expression is a step towards functional programming. Lambda expressions are approximately like an arrow function in JavaScript. Following are the syntax of the lambda functions.
(parameter) -> { /*statements Here*/
}
// Lambda Expressions With Single Parameter......
(parameter1, parameter2) -> { /*statements Here*/
}
// Lambda Expressions With two Parameter......
Let’s see the first example of lambda.
List<Integer> numbers = new ArrayList<Integer>(); // Example Array list
numbers.add(5);
numbers.add(9);
numbers.add(8);
numbers.add(1);
// e.g Lambda Expressions With No Parameter......
Consumer<Integer> cons = (index) -> {
System.out.println(index);
};
numbers.forEach(cons);
// Lambda Expression End Here............
The above code displays the array list. Now that you have seen the lambda syntax, let’s look at the lambda comparator.
Interface Comparator<T>
is a functional (lambda expression) interface and can be used as the assignment target for a lambda expression or method reference. A comparison function is used for ordering collections(arraylist)
of objects.
Comparators can be passed to a sort method (Collections.sort
or Arrays.sort
). We’ll use these methods to sort the whole data set defined.
Take a look at the following code.
List<Employee> totalEmployees = new ArrayList<Employee>();
totalEmployees.add(new Employee(24, "RavigHambole", 5000));
totalEmployees.add(new Employee(26, "Bill Gates", 400));
totalEmployees.add(new Employee(34, "Mark Zukerberg", 40000));
totalEmployees.add(new Employee(56, "Sundar Picahi", 300));
totalEmployees.add(new Employee(34, "Potus", 1220));
totalEmployees.add(new Employee(23, "Obama", 5032));
totalEmployees.add(new Employee(15, "Halary Clinton", 5030));
totalEmployees.add(new Employee(23, "David", 500320));
// Now Compartor Method To Sort According to Age......
System.out.println("Before Sort");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary :" + temp.Salary);
}
// simply defined and displayed the array list
We have defined a data set featuring a name, age, and salary in the code example. We can either sort this data by age or by salary.
Let’s see how the lambda expression helps us in this regard.
Comparator<Employee> ee = new Comparator<Employee>() {
// Overriding Comparator method to sort according to age
@Override
public int compare(Employee o1, Employee o2) {
return o2.age - o1.age;
}
};
// Now Sorting the Method.... According to age
totalEmployees.sort((Employee o1, Employee o2) -> o1.age - o2.age);
System.out.println("After Sort by age..........");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary" + temp.Salary);
}
There’s a built-in functionality defined for the comparator. We override that by deploying our logic of comparing the ages and sorting the data set by age.
Later, we are simply passing the employees as parameters in lambda. We defined the functionality by comparing the ages of both parameters (employees).
All the above codes are pieces of the complete code down below. You need to add libraries and all classes to run them.
So, run the following code.
the Complete Code
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.function.Consumer;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<Integer>();
numbers.add(5);
numbers.add(9);
numbers.add(8);
numbers.add(1);
Consumer<Integer> cons = (index) -> {
System.out.println(index);
};
numbers.forEach(cons);
List<Employee> totalEmployees = new ArrayList<Employee>();
totalEmployees.add(new Employee(24, "RavigHambole", 5000));
totalEmployees.add(new Employee(26, "Bill Gates", 400));
totalEmployees.add(new Employee(34, "Mark Zukerberg", 40000));
totalEmployees.add(new Employee(56, "Sundar Picahi", 300));
totalEmployees.add(new Employee(34, "Potus", 1220));
totalEmployees.add(new Employee(23, "Obama", 5032));
totalEmployees.add(new Employee(15, "Halary Clinton", 5030));
totalEmployees.add(new Employee(23, "David", 500320));
// Now Compartor Method To Sort According to Age......
System.out.println("Before Sort");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary :" + temp.Salary);
}
Comparator<Employee> ee = new Comparator<Employee>() {
// Overirding Compartor method to sort according to
// age
@Override
public int compare(Employee o1, Employee o2) {
return o2.age - o1.age;
}
};
totalEmployees.sort(ee);
// Now Sorting the Method.... According to age
totalEmployees.sort((Employee o1, Employee o2) -> o1.age - o2.age);
System.out.println("After Sort by age..........");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary" + temp.Salary);
}
}
}
class Employee { // Employee Class..
public int age;
public String Name;
public int Salary;
Employee(int a, String Na, int Sa) {
this.age = a;
this.Name = Na;
this.Salary = Sa;
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn