Java 中的 Lambda 比較器
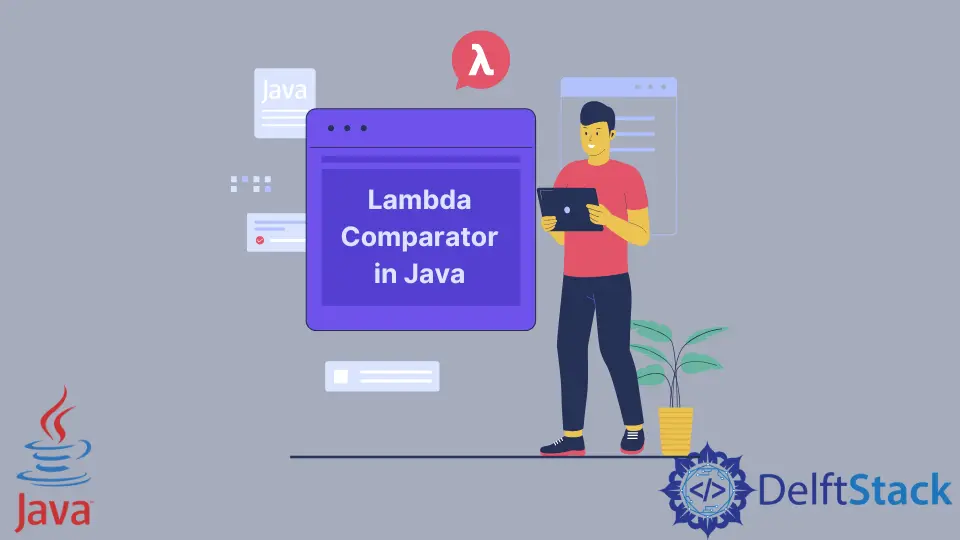
本指南將處理 Java 程式語言中的 lambda 比較器。
我們需要了解 Lambda 的基本理解才能理解比較器函式。讓我們深入瞭解本指南。
Java 中的 Lambda 比較器
lambda 表示式是一個接受引數並返回值的程式碼塊。
Lambda 表示式類似於方法。但是,它們不需要像方法這樣的名稱,它們可以直接在方法體中實現。
Lambda 表示式是朝著函數語言程式設計邁出的一步。Lambda 表示式類似於 JavaScript 中的箭頭函式。以下是 lambda 函式的語法。
(parameter) -> { /*statements Here*/
}
// Lambda Expressions With Single Parameter......
(parameter1, parameter2) -> { /*statements Here*/
}
// Lambda Expressions With two Parameter......
讓我們看一下 lambda 的第一個示例。
List<Integer> numbers = new ArrayList<Integer>(); // Example Array list
numbers.add(5);
numbers.add(9);
numbers.add(8);
numbers.add(1);
// e.g Lambda Expressions With No Parameter......
Consumer<Integer> cons = (index) -> {
System.out.println(index);
};
numbers.forEach(cons);
// Lambda Expression End Here............
上面的程式碼顯示了陣列列表。現在你已經瞭解了 lambda 語法,讓我們來看看 lambda 比較器。
介面 Comparator<T>
是一個函式式(lambda 表示式)介面,可用作 lambda 表示式或方法引用的賦值目標。比較函式用於對物件的 collections(arraylist)
進行排序。
比較器可以傳遞給排序方法(Collections.sort
或 Arrays.sort
)。我們將使用這些方法對定義的整個資料集進行排序。
看看下面的程式碼。
List<Employee> totalEmployees = new ArrayList<Employee>();
totalEmployees.add(new Employee(24, "RavigHambole", 5000));
totalEmployees.add(new Employee(26, "Bill Gates", 400));
totalEmployees.add(new Employee(34, "Mark Zukerberg", 40000));
totalEmployees.add(new Employee(56, "Sundar Picahi", 300));
totalEmployees.add(new Employee(34, "Potus", 1220));
totalEmployees.add(new Employee(23, "Obama", 5032));
totalEmployees.add(new Employee(15, "Halary Clinton", 5030));
totalEmployees.add(new Employee(23, "David", 500320));
// Now Compartor Method To Sort According to Age......
System.out.println("Before Sort");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary :" + temp.Salary);
}
// simply defined and displayed the array list
我們在程式碼示例中定義了一個包含姓名、年齡和薪水的資料集。我們可以按年齡或按薪水對這些資料進行排序。
讓我們看看 lambda 表示式在這方面如何幫助我們。
Comparator<Employee> ee = new Comparator<Employee>() {
// Overriding Comparator method to sort according to age
@Override
public int compare(Employee o1, Employee o2) {
return o2.age - o1.age;
}
};
// Now Sorting the Method.... According to age
totalEmployees.sort((Employee o1, Employee o2) -> o1.age - o2.age);
System.out.println("After Sort by age..........");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary" + temp.Salary);
}
為比較器定義了一個內建功能。我們通過部署比較年齡和按年齡對資料集進行排序的邏輯來覆蓋它。
稍後,我們只是將員工作為引數傳遞給 lambda。我們通過比較兩個引數(員工)的年齡來定義功能。
以上所有程式碼都是下面完整程式碼的一部分。你需要新增庫和所有類來執行它們。
因此,執行以下程式碼。
完整程式碼
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.function.Consumer;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<Integer>();
numbers.add(5);
numbers.add(9);
numbers.add(8);
numbers.add(1);
Consumer<Integer> cons = (index) -> {
System.out.println(index);
};
numbers.forEach(cons);
List<Employee> totalEmployees = new ArrayList<Employee>();
totalEmployees.add(new Employee(24, "RavigHambole", 5000));
totalEmployees.add(new Employee(26, "Bill Gates", 400));
totalEmployees.add(new Employee(34, "Mark Zukerberg", 40000));
totalEmployees.add(new Employee(56, "Sundar Picahi", 300));
totalEmployees.add(new Employee(34, "Potus", 1220));
totalEmployees.add(new Employee(23, "Obama", 5032));
totalEmployees.add(new Employee(15, "Halary Clinton", 5030));
totalEmployees.add(new Employee(23, "David", 500320));
// Now Compartor Method To Sort According to Age......
System.out.println("Before Sort");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary :" + temp.Salary);
}
Comparator<Employee> ee = new Comparator<Employee>() {
// Overirding Compartor method to sort according to
// age
@Override
public int compare(Employee o1, Employee o2) {
return o2.age - o1.age;
}
};
totalEmployees.sort(ee);
// Now Sorting the Method.... According to age
totalEmployees.sort((Employee o1, Employee o2) -> o1.age - o2.age);
System.out.println("After Sort by age..........");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary" + temp.Salary);
}
}
}
class Employee { // Employee Class..
public int age;
public String Name;
public int Salary;
Employee(int a, String Na, int Sa) {
this.age = a;
this.Name = Na;
this.Salary = Sa;
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn