Java の Lambda コンパレータ
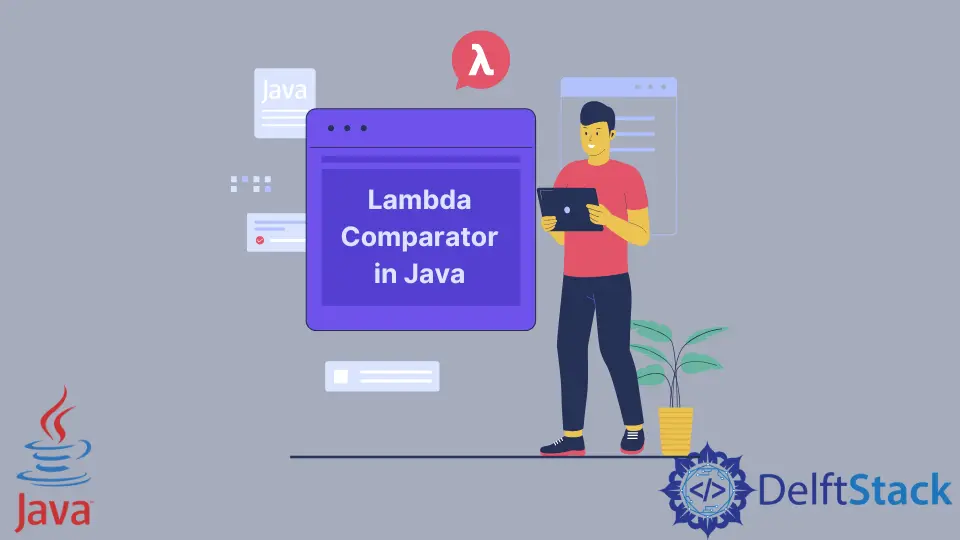
このガイドでは、Java プログラミング言語のラムダコンパレータに取り組みます。
コンパレータ関数を理解するには、ラムダの基本的な理解を理解する必要があります。このガイドを詳しく見ていきましょう。
Java の Lambda コンパレータ
ラムダ式は、パラメーターを受け入れるコードブロックであり、値を返します。
ラムダ式はメソッドに似ています。ただし、メソッドのような名前は必要なく、メソッドの本体に直接実装できます。
ラムダ式は関数型プログラミングへの第一歩です。ラムダ式は、JavaScript の矢印関数にほぼ似ています。ラムダ関数の構文は次のとおりです。
(parameter) -> { /*statements Here*/
}
// Lambda Expressions With Single Parameter......
(parameter1, parameter2) -> { /*statements Here*/
}
// Lambda Expressions With two Parameter......
ラムダの最初の例を見てみましょう。
List<Integer> numbers = new ArrayList<Integer>(); // Example Array list
numbers.add(5);
numbers.add(9);
numbers.add(8);
numbers.add(1);
// e.g Lambda Expressions With No Parameter......
Consumer<Integer> cons = (index) -> {
System.out.println(index);
};
numbers.forEach(cons);
// Lambda Expression End Here............
上記のコードは配列リストを表示します。ラムダ構文を確認したので、ラムダコンパレータを見てみましょう。
インターフェイス Comparator<T>
は機能(ラムダ式)インターフェイスであり、ラムダ式またはメソッド参照の割り当てターゲットとして使用できます。比較関数は、オブジェクトの collections(arraylist)
を並べ替えるために使用されます。
コンパレータは、ソートメソッド(Collections.sort
または Arrays.sort
)に渡すことができます。これらのメソッドを使用して、定義されたデータセット全体を並べ替えます。
次のコードを見てください。
List<Employee> totalEmployees = new ArrayList<Employee>();
totalEmployees.add(new Employee(24, "RavigHambole", 5000));
totalEmployees.add(new Employee(26, "Bill Gates", 400));
totalEmployees.add(new Employee(34, "Mark Zukerberg", 40000));
totalEmployees.add(new Employee(56, "Sundar Picahi", 300));
totalEmployees.add(new Employee(34, "Potus", 1220));
totalEmployees.add(new Employee(23, "Obama", 5032));
totalEmployees.add(new Employee(15, "Halary Clinton", 5030));
totalEmployees.add(new Employee(23, "David", 500320));
// Now Compartor Method To Sort According to Age......
System.out.println("Before Sort");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary :" + temp.Salary);
}
// simply defined and displayed the array list
コード例では、名前、年齢、給与を特徴とするデータセットを定義しました。このデータは、年齢または給与で並べ替えることができます。
ラムダ式がこの点でどのように役立つかを見てみましょう。
Comparator<Employee> ee = new Comparator<Employee>() {
// Overriding Comparator method to sort according to age
@Override
public int compare(Employee o1, Employee o2) {
return o2.age - o1.age;
}
};
// Now Sorting the Method.... According to age
totalEmployees.sort((Employee o1, Employee o2) -> o1.age - o2.age);
System.out.println("After Sort by age..........");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary" + temp.Salary);
}
コンパレータ用に定義された組み込み機能があります。年齢を比較し、データセットを年齢で並べ替えるロジックを展開することで、これをオーバーライドします。
後で、ラムダのパラメーターとして従業員を渡すだけです。両方のパラメーター(従業員)の年齢を比較することにより、機能を定義しました。
上記のコードはすべて、以下の完全なコードの一部です。それらを実行するには、ライブラリとすべてのクラスを追加する必要があります。
したがって、次のコードを実行します。
完全なコード
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.function.Consumer;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<Integer>();
numbers.add(5);
numbers.add(9);
numbers.add(8);
numbers.add(1);
Consumer<Integer> cons = (index) -> {
System.out.println(index);
};
numbers.forEach(cons);
List<Employee> totalEmployees = new ArrayList<Employee>();
totalEmployees.add(new Employee(24, "RavigHambole", 5000));
totalEmployees.add(new Employee(26, "Bill Gates", 400));
totalEmployees.add(new Employee(34, "Mark Zukerberg", 40000));
totalEmployees.add(new Employee(56, "Sundar Picahi", 300));
totalEmployees.add(new Employee(34, "Potus", 1220));
totalEmployees.add(new Employee(23, "Obama", 5032));
totalEmployees.add(new Employee(15, "Halary Clinton", 5030));
totalEmployees.add(new Employee(23, "David", 500320));
// Now Compartor Method To Sort According to Age......
System.out.println("Before Sort");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary :" + temp.Salary);
}
Comparator<Employee> ee = new Comparator<Employee>() {
// Overirding Compartor method to sort according to
// age
@Override
public int compare(Employee o1, Employee o2) {
return o2.age - o1.age;
}
};
totalEmployees.sort(ee);
// Now Sorting the Method.... According to age
totalEmployees.sort((Employee o1, Employee o2) -> o1.age - o2.age);
System.out.println("After Sort by age..........");
for (Employee temp : totalEmployees) {
System.out.println("Name :" + temp.Name);
System.out.println("Age :" + temp.age);
System.out.println("Salary" + temp.Salary);
}
}
}
class Employee { // Employee Class..
public int age;
public String Name;
public int Salary;
Employee(int a, String Na, int Sa) {
this.age = a;
this.Name = Na;
this.Salary = Sa;
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn