Iterator and Iterable Interfaces in Java
- The Difference Between Iterator and Iterable in Java
- Implementation of Iterator in Java
- Custom Iterable and Iterator in Java
- Iterator and Iterable Solved in Java
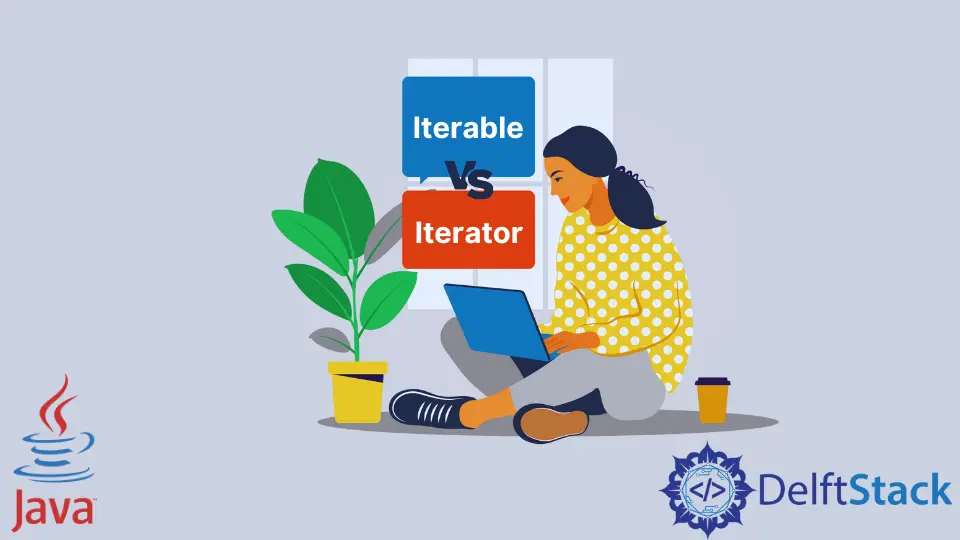
An iterable is like a collection of goods in a warehouse, whereas an iterator is the keeper of that warehouse.
One might not know how many products are there? And thus, one might ask the iterator, do you have this item or not?
The Difference Between Iterator and Iterable in Java
Iterator and iterable are collections of objects. These are not any different from the arrays and wrappers.
Though, both methods override them to allow you to inherit the objects in a way that best suits your requirement.
An object can target an extended for
statement in the iterable interface, popularly known as a for each
loop. Its type parameter is <T>
, specifies the type of elements returned by the iterator<>
.
Syntax:
public class IteratorAndIterable<T> implements Iterable<T> {}
An iterator<>
returns an instance of the iterator <T>
elements.
It is also an object, a collector, or simply an interface. The following parameter will further deal with the returned instance.
Parameters:
hasNext();
: It returns true, if the iteration has additional elements.next();
: This method returns the next element in the iteration.remove();
: Removes the final element returned from the underlying collection by this iterator.
Syntax:
// Custom Iterator
public class myCustomiterator<T> implements Iterator<T> {
// hasNext()
public boolean hasNext() {
// your code goes here
return false;
}
// next()
public E next() {
// your code goes here
return false;
}
}
Implementation of Iterator in Java
We will dynamically generate an iterator<>
from the array list to show you how to traverse each element.
Code:
import java.util.*;
// Main class
public class ExplainIterator {
public static void main(String[] args) {
ArrayList<String> demolist = new ArrayList<String>();
demolist.add("Here");
demolist.add("Is");
demolist.add("Iterator");
demolist.add("Demo");
/*
* Now, we are going to dynamically generate Iterator from the Arrays List for
* traversing each element
*/
/*
* Iterator method has two parameters: hasNext() and next(). The hasNext()
* returns boolean (true or false). While the next() method returns next
* complete token (it hasNext() gave it one).
*/
Iterator<String> demo = demolist.iterator();
while (demo.hasNext()) {
System.out.println(demo.next());
}
// Iterator method
}
// main function
}
// main class
In this example, the iterator<>
method discussed two parameters, hasNext()
and next()
, worked perfectly fine.
The hasNext()
returned true. While the next()
method returned complete token because hasNext()
gave it one.
Finally, we looped the index out of the iterator<>
.
Output:
Here
Is
Iterator
Demo
Custom Iterable and Iterator in Java
Do you remember the real-world example of these concepts? If yes, then now is the time to implement these methods as they work.
In Java, you have to use an iterator with the iterable. Your iterable<>
collection must return you a specific object with the help of its iterator.
You will precisely learn what it is all about in the following code block.
IteratorAndIterable.java
:
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
// You will inherit it
// Define Custom Iterable
public class IteratorAndIterable<T> implements Iterable<T> {
// An array list (setL: setlist)
private List<T> setL = new ArrayList<>();
// Get value from the List
public void add(T getback) {
setL.add(getback);
}
// Define Iterator to get your iterable objects
public Iterator<T> iterator() {
return new myCi<T>(setL);
}
// Your custom iterator overrides Iterator
public class myCi<E> implements Iterator<E> {
// For a condition to avoid exception later
int SP = 0;
// Custom Array List
List<E> CAL;
public myCi(List<E> CAL) {
this.CAL = CAL;
}
// Check the iterator values
public boolean hasNext() {
if (CAL.size() >= SP + 1) {
// Remember SP = 0? To avoid exception
// If hasNext() has value, it returns true
return true;
}
return false;
}
// Use next() to get the token that hasNext() just passed
// No need to use default values, make use of the your custom <E>
public E next() {
// Get token and store it into getback
E getback = CAL.get(SP);
// You can do anything with this loop now
SP++;
// return getback instead of deafault value (Now check line number 122) It will be clear
return getback;
}
public void remove() {}
}
}
Iterator and Iterable Solved in Java
This code explained each line of code step by step. Please check the comments. We extended an Iterable<>
and created our own.
Later, we created an iterator<>
inside the iterable<>
and overrode exactly how we wanted it to behave.
The iterator’s hasNext()
passed via next()
, and our custom list was ready to pick it.
Now we will create a simple class to define an array list. Then we will show you how you can get the object of iterable from this code block and print it on the next.
next()
passed by the hasNext()
, iterated from the custom iterable with a custom iterator.ExecuteIterable.java
:
// Get object from the iterable
public abstract class ExecuteIterable {
public static void main(String[] args) {
// Your former class of custom Iterable and Iterator
IteratorAndIterable<String> Lst = new IteratorAndIterable<>();
// Valuse
Lst.add("EAST");
Lst.add("WEST");
Lst.add("NORTH");
Lst.add("SOUTH");
// For each loop to extract values from the iterator
for (String indx : Lst) {
// Print
System.out.println(indx);
}
}
}
Output:
EAST
WEST
NORTH
SOUTH
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn