Java Iterator remove() Method
-
Introduction to
Collection
’sremove()
Method -
Use the
remove()
Method ofCollection
-
Introduction to
Iterator
’sremove()
Method -
Use the
Iterator
’sremove()
Method
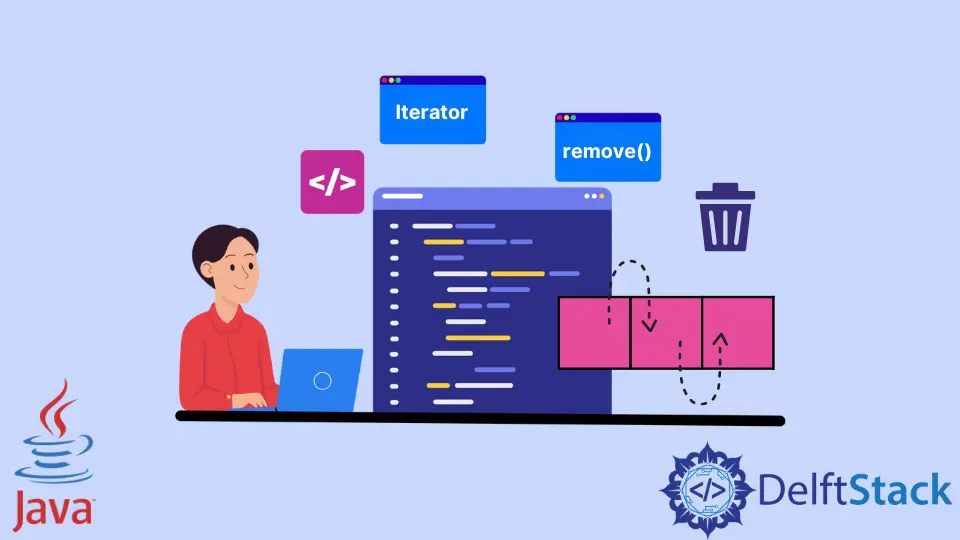
Java developers often need to remove the element or object from the ArrayList
while iterating.
Here, the problem arises when they try to remove the object from the original collection while looping through it as it throws the ConcurrentModificationException
. In this article, we will learn how the Iterator
’s remove()
method and the Collection
’s remove()
method works differently.
Introduction to Collection
’s remove()
Method
In Java, Collection
is an interface that contains different data types such as ArrayList
, Set
, etc. To remove an element from the Collection
, we can use the remove()
method by taking the collection as a reference.
Users can follow the syntax below to use the remove()
method with the collection.
collection.remove(Ind);
Ind
- The remove()
method removes elements from the Ind
index of referenced collection
.
Use the remove()
Method of Collection
In the example below, we have created the collection and removed the element from the 2nd and 4th index using the remove()
method of the collection
.
import java.util.ArrayList;
class Test {
public static void main(String[] args) {
// Create an ArrayList
ArrayList<String> testList = new ArrayList<String>();
// Add elements in the ArrayList
testList.add("Apple");
testList.add("Banana");
testList.add("Cat");
testList.add("Dog");
testList.add("Fox");
testList.add("Cow");
// Removing the element at index 2 and 4
System.out.println(testList.remove(2) + " removed\n");
System.out.println(testList.remove(4) + " removed");
}
}
Output:
Cat removed
Cow removed
In the above output, users can see that once we remove the cat
from the 2nd index, all elements in ArrayList
shift by one index.
When we try to remove the element from the 4th index after removing the element from the 2nd index, the remove()
method removes the element from the 5th index of the actual ArrayList
as all elements after the 2nd index shifted to the left by 1.
We have learned how the Collection
’s remove()
method works normally.
Introduction to Iterator
’s remove()
Method
Now, the question is, what is the difference between the Iterator
’s and Collection
’s remove()
methods, as both remove the element from the Collection
?
Let’s understand the problem using the below code.
import java.util.ArrayList;
class Test {
public static void main(String[] args) {
// Create an ArrayList
ArrayList<String> testList = new ArrayList<String>();
// Add elements in the ArrayList
testList.add("Apple");
testList.add("Banana");
testList.add("Cat");
testList.add("Dog");
testList.add("Fox");
testList.add("Cow");
for (String element : testList) {
if (element.equals("Cat")) {
testList.remove(element);
}
}
}
}
In the above example, we have created the ArrayList
of different strings. We are iterating through the ArrayList
using the for
loop and checking that if the element is equal to cat
, we try to remove it using the collection.remove()
method.
In the output, users can see that it throws the ConcurrentModificationException
exception as we modify the original list while iterating through it.
Output:
Exception in thread "main" java.util.ConcurrentModificationException
To solve our problem, we can use the iterator
to iterate through the ArrayList
and the remove()
method of the Iterator
to remove the element.
Use the Iterator
’s remove()
Method
In the example below, we have created the iterator name itr
for the ArrayList
of the string. We are iterating through the ArrayList
using the for
loop and itr
.
Also, we are removing the Fox
element from the ArrayList
while iterating through it.
import java.util.ArrayList;
import java.util.Iterator;
class Test {
public static void main(String[] args) {
// Create an ArrayList
ArrayList<String> testList = new ArrayList<String>();
// Add elements in the ArrayList
testList.add("Apple");
testList.add("Banana");
testList.add("Cat");
testList.add("Dog");
testList.add("Fox");
testList.add("Cow");
// Removing the "Fox" while iterating through the `testList`
Iterator<String> itr = testList.iterator();
while (itr.hasNext()) {
String element = itr.next();
if (element.equals("Fox")) {
testList.remove(element);
}
}
System.out.println(testList);
}
}
Output:
[Apple, Banana, Cat, Dog, Cow]
We can observe that Fox
is removed while iterating through the ArrayList
in the above output.
Understand the Code of Iterator
’s remove()
Method
We have taken the below pseudo code of the iterator
’s remove()
method from the official docs of Java. Users can see that it also uses the remove()
method of ArrayList
, but also it updates the expectedModCount
, which doesn’t throw the ConcurrentModificationException
exception.
It is always required to know the next element when we iterate through the ArrayList
. So, if we try to modify the original list using the remove()
method, it will not update the next index and throws the error, and that problem is overcome in the iterator
’s remove()
method.
try {
ArrayList.this.remove(lastRet);
cursor = lastRet;
lastRet = -1;
expectedModCount = modCount;
} catch (IndexOutOfBoundsException ex) {
throw new ConcurrentModificationException();
}
Also, users can clone the list and iterate through the new list. While iterating through the new list, they can remove the element from the original list.