Custom Iterator in Java
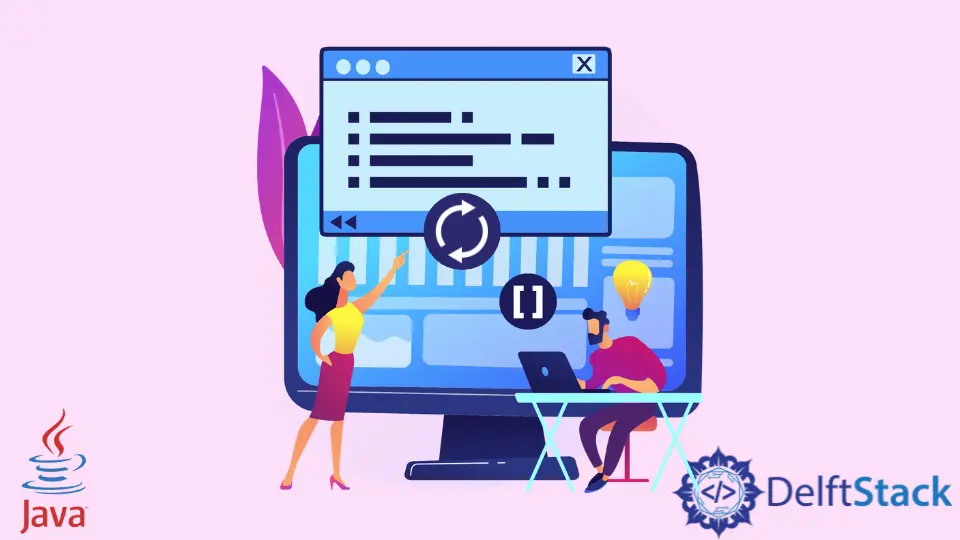
In this guide, we are going to learn how to make a custom iterator in Java.
An iterator in Java is a pretty useful tool. You can consider it as an alternative to the foreach
loop.
An iterator features some functions which assist the developers in updating a well-defined collection. Let’s see how we can make a custom iterator in Java.
Learn more about iterators in Java here.
Custom Iterator in Java
Custom iterators in Java are used to provide a tailored and application-specific mechanism for iterating over collections. While Java offers built-in iterators for standard data structures, there are scenarios where a more specialized iteration is required.
Custom iterators allow developers to define their own logic for traversing elements, accommodating unique data structures or specific iteration requirements. This customization enhances code readability and maintainability, as developers can encapsulate complex iteration logic within the iterator, promoting a clean separation of concerns.
Additionally, custom iterators facilitate seamless integration with Java’s enhanced for
loops and functional programming constructs, enabling a more expressive and efficient approach to handling collections in diverse application scenarios.
Ways to Create a Custom Iterator in Java
Custom Iterators With Iterator
Interface
The Iterator interface is crucial for custom iterators in Java as it provides a standardized, efficient, and consistent way to traverse collections. By adhering to this interface, custom iterators seamlessly integrate with Java’s enhanced for
loops and other collection-processing methods, promoting code readability and maintainability.
This adherence ensures compatibility across various data structures and facilitates a more cohesive and conversational approach to iteration in Java.
Example Code:
import java.util.Iterator;
import java.util.NoSuchElementException;
public class CustomIteratorExample implements Iterator<String> {
private String[] elements;
private int currentIndex;
public CustomIteratorExample(String[] elements) {
this.elements = elements;
this.currentIndex = 0;
}
@Override
public boolean hasNext() {
return currentIndex < elements.length;
}
@Override
public String next() {
if (hasNext()) {
return elements[currentIndex++];
} else {
throw new NoSuchElementException();
}
}
// Optional: Implement remove() if needed
// public void remove() { ... }
public static void main(String[] args) {
String[] data = {"one", "two", "three"};
CustomIteratorExample customIterator = new CustomIteratorExample(data);
while (customIterator.hasNext()) {
System.out.println(customIterator.next());
}
}
}
In this code example, we’ve crafted a CustomIteratorExample
class that implements the Iterator
interface. The class manages a String
array and a currentIndex
to track the current position during iteration.
The constructor initializes the array and sets the index to zero. The hasNext()
method checks for remaining elements, and next()
retrieves the current element, incrementing the index.
The optional remove()
method supports element removal. In the main
method, an instance is created, and a while
loop iterates over the elements, printing each to the console.
This structure ensures a clear, standardized, and efficient approach to custom iteration in Java.
Output:
This example demonstrates the power and flexibility of creating custom iterators in Java. By implementing the Iterator
interface and defining the hasNext
, next
, and optionally remove
methods, developers can tailor iterators to suit specific requirements, providing a more efficient and elegant solution for traversing collections.
Custom Iterators With Java 8 Streams
Java 8 introduced a paradigm shift in the way developers approach iteration and collection processing with the introduction of Streams. While Streams provide powerful abstractions for working with sequences of elements, there are scenarios where a more customized iteration is needed.
In this section, we’ll explore the creation of custom iterators using the hasNext
, next
, and remove
methods within Java 8 Streams. This enables developers to build tailored iteration mechanisms seamlessly integrated with the functional programming style of Java 8.
Example Code:
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.stream.Stream;
public class CustomIteratorWithStreamsExample {
public static void main(String[] args) {
List<String> dataList = new ArrayList<>();
dataList.add("apple");
dataList.add("banana");
dataList.add("orange");
Stream<String> customStream = createCustomIterator(dataList);
customStream.forEach(System.out::println);
}
private static <T> Stream<T> createCustomIterator(List<T> dataList) {
Iterator<T> customIterator = new Iterator<T>() {
private int currentIndex = 0;
@Override
public boolean hasNext() {
return currentIndex < dataList.size();
}
@Override
public T next() {
if (hasNext()) {
return dataList.get(currentIndex++);
} else {
throw new java.util.NoSuchElementException();
}
}
// Optional: Implement remove() if needed
// public void remove() { ... }
};
return Stream.generate(customIterator::next).limit(dataList.size());
}
}
In this Java 8 Streams example, our goal is to create a custom iterator for a list of strings (dataList
). The main
method initializes the list and calls the createCustomIterator
method to obtain a custom stream.
The forEach
terminal operation is then employed to print each element of the stream.
The createCustomIterator
method takes a list as a parameter, returning a custom stream. It utilizes an anonymous inner class implementing the Iterator
interface with overridden hasNext
and next
methods.
An optional remove
method is provided, and the stream is generated using the custom iterator’s next
method, limited to the original list’s size.
Output:
Combining the power of custom iterators and Java 8 Streams, developers can seamlessly integrate customized iteration logic into functional programming paradigms. This approach provides a versatile and expressive way to handle collections, making code more readable and maintainable while retaining the efficiency of stream processing.
Custom Iterators in Java Collections
Custom iterators in Java collections provide a crucial mechanism for tailoring iteration to specific application needs. Unlike standard iterators, custom iterators empower developers to implement specialized logic for traversing and manipulating collections efficiently.
This approach enhances code readability, promotes a clean separation of concerns, and ensures adaptability to unique data structures. Custom iterators offer a versatile solution, fostering a more expressive and idiomatic way to handle collections compared to generic or traditional methods.
Example Code:
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class CustomIteratorWithCollectionsExample {
public static void main(String[] args) {
List<String> dataList = new ArrayList<>();
dataList.add("apple");
dataList.add("banana");
dataList.add("orange");
CustomIterator<String> customIterator = new CustomIterator<>(dataList);
while (customIterator.hasNext()) {
System.out.println(customIterator.next());
}
}
static class CustomIterator<T> implements Iterator<T> {
private List<T> elements;
private int currentIndex;
public CustomIterator(List<T> elements) {
this.elements = elements;
this.currentIndex = 0;
}
@Override
public boolean hasNext() {
return currentIndex < elements.size();
}
@Override
public T next() {
if (hasNext()) {
return elements.get(currentIndex++);
} else {
throw new java.util.NoSuchElementException();
}
}
// Optional: Implement remove() if needed
// public void remove() { ... }
}
}
In this example, we traverse and print a list of fruits using a custom iterator. In the main
method, a list of strings (dataList
) is created, and a custom iterator (CustomIterator
) is instantiated for iteration.
The CustomIterator
class, implementing the Iterator
interface, maintains a list of elements and a currentIndex
. The hasNext()
method checks for remaining elements, and next()
retrieves the current element, incrementing the index.
If there are no more elements, a NoSuchElementException
is thrown.
The optional remove()
method supports element removal. This structured approach ensures a clear, standardized, and efficient custom iteration in Java.
Output:
In this exploration of custom iterators within Java collections, we witnessed the creation of a specialized iterator tailored to a list of fruits. By implementing the hasNext
, next
, and optionally remove
methods, developers can craft iterators that seamlessly integrate with their unique use cases.
The flexibility and control offered by custom iterators empower developers to navigate through collections in a way that aligns precisely with the requirements of their applications.
Conclusion
The exploration of custom iterators in Java, employing the Iterator interface, Java 8 Streams, and Java Collections, provides developers with versatile tools for tailored collection traversal. Whether adhering to traditional Iterator patterns or embracing the functional paradigm of Java 8 Streams, these methods enhance code flexibility, readability, and efficiency.
The ability to create custom iterators aligned with specific application requirements underscores their significance in facilitating expressive and effective collection handling within Java programming.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn