How to Create a Java for Loop With Two Variables
-
Java
for
Loop With Multiple Variables of the Same Type -
Java
for
Loop With Multiple Variables of Different Types
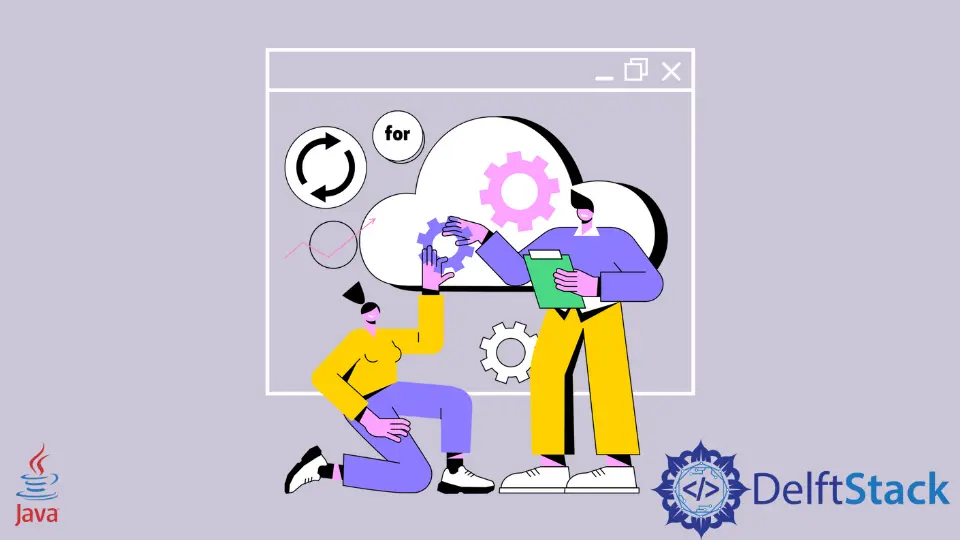
This tutorial explains how we can use multiple variables in Java for
loop. We can achieve this by following the syntax of the java for
loop properly.
Java for
Loop With Multiple Variables of the Same Type
We are using two variables inside the for
loop of the int
type. The part before the first ;
is the initialization part where we can initialize multiple variables separated by a comma. The part before the second ;
is the condition part, and after it is the manipulation part. &&
and ||
operators can be used to make a condition.
public class ForLoop {
public static void main(String[] args) {
for (int i = 0, j = 10; i < 10 && j > 0; i++, j--) {
System.out.println("i = " + i + " :: "
+ "j = " + j);
}
}
}
Output:
i = 0 :: j = 10
i = 1 :: j = 9
i = 2 :: j = 8
i = 3 :: j = 7
i = 4 :: j = 6
i = 5 :: j = 5
i = 6 :: j = 4
i = 7 :: j = 3
i = 8 :: j = 2
i = 9 :: j = 1
In the above code, we declare two variables - i
and j
with the same type as int
. i
will start with 0 and keep on incrementing till 10, and j
will start with 10 and keep on decrementing till 0.
Multiple conditions separated by a comma is not correct as it will give an error which can be understood by a simple example as shown below.
public class ForLoop {
public static void main(String[] args) {
for (int i = 0, j = 10; i<10, j> 0; i++, j--) {
System.out.println("i = " + i + " :: "
+ "j = " + j);
}
}
}
Output:
error: ';' expected
for (int i = 0, j = 10; i < 10 , j > 0; i++, j--) {
^
ForLoopSame.java:6: error: illegal start of expression
for (int i = 0, j = 10; i < 10 , j > 0; i++, j--) {
^
ForLoopSame.java:6: error: ')' expected
for (int i = 0, j = 10; i < 10 , j > 0; i++, j--) {
^
ForLoopSame.java:6: error: illegal start of expression
for (int i = 0, j = 10; i < 10 , j > 0; i++, j--) {
^
ForLoopSame.java:6: error: ';' expected
for (int i = 0, j = 10; i < 10 , j > 0; i++, j--) {
^
ForLoopSame.java:6: error: ';' expected
for (int i = 0, j = 10; i < 10 , j > 0; i++, j--) {
^
ForLoopSame.java:6: error: ';' expected
for (int i = 0, j = 10; i < 10 , j > 0; i++, j--) {
^
7 errors
Java for
Loop With Multiple Variables of Different Types
This example is slightly different. It has two variables, y
and z
, of the same type, which are declared and initialized in the loop. The other variable x
is declared and initialized outside the loop later used in the loop’s condition part. Re-initializing a variable and changing its type will result in an error.
public class ForLoop {
public static void main(String[] args) {
int x = 2;
for (long y = 0, z = 4; x < 10 && y < 10; x++, y++) {
System.out.println("y: " + y);
}
System.out.println("x : " + x);
}
}
Output:
y: 0
y: 1
y: 2
y: 3
y: 4
y: 5
y: 6
y: 7
x : 10
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn