How to Exit a While Loop in Java
-
Exit a
while
Loop After Completing the Program Execution in Java -
Exit a
while
Loop by Usingbreak
in Java -
Exit a
while
Loop by Usingreturn
in Java
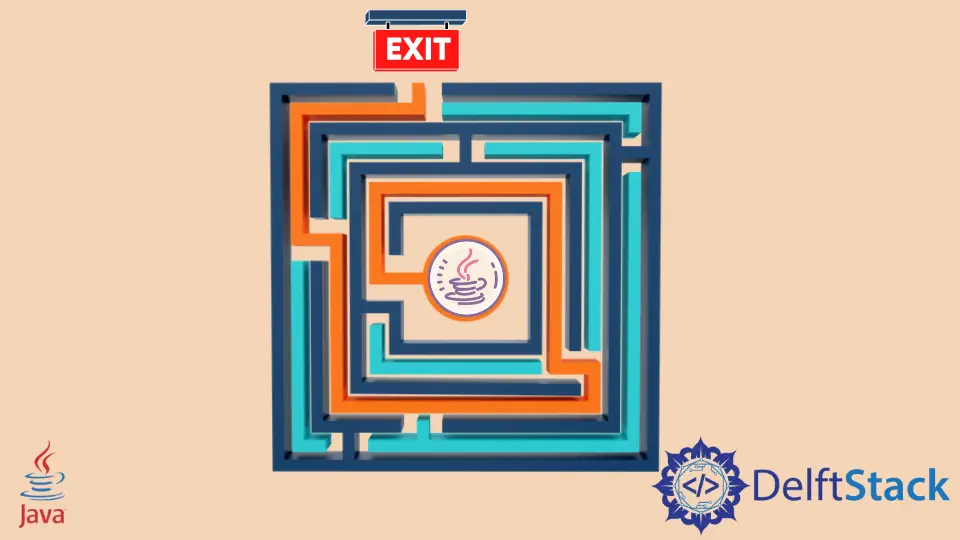
This tutorial introduces how you can exit a while-loop in Java and handle it with some example codes to help you understand the topic further.
The while-loop is one of the Java loops used to iterate or repeat the statements until they meet the specified condition. To exit the while-loop, you can do the following methods:
- Exit after completing the loop normally
- Exit by using the
break
statement - Exit by using the
return
statement
Exit a while
Loop After Completing the Program Execution in Java
This method is a simple example where a while-loop exits itself after the specified condition marks as false
.
The while-loop repeatedly executes until the specified condition is true
and exits if the condition is false
. See the example below where we are iterate list elements using the while-loop and have the loop exit when all the elements get traversed.
import java.util.Arrays;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<Integer> list = Arrays.asList(new Integer[] {12, 34, 21, 33, 22, 55});
int i = 0;
while (i < list.size()) {
System.out.println(list.get(i));
i++;
}
}
}
Output:
12
34
21
33
22
55
Exit a while
Loop by Using break
in Java
This way is another solution where we used a break-statement to exit the loop. The break-statement is used to cut the current execution thread, and control goes outside the loop that leads the loop to exit in between. You can use break
to exit the while-loop explicitly. See the example below:
import java.util.Arrays;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<Integer> list = Arrays.asList(new Integer[] {12, 34, 21, 33, 22, 55});
int i = 0;
while (i < list.size()) {
if (i == 3)
break;
System.out.println(list.get(i));
i++;
}
}
}
Output:
12
34
21
Exit a while
Loop by Using return
in Java
Java uses a return-statement to return a response to the caller method, and control immediately transfers to the caller by exiting a loop(if it exists). So we can use return
to exit the while-loop too. Check the code below to see how we used return
.
import java.util.Arrays;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
boolean result = show();
if (result) {
System.out.println("Loop Exit explicitly");
} else
System.out.println("Loop not exit explicitly");
}
static boolean show() {
List<Integer> list = Arrays.asList(new Integer[] {12, 34, 21, 33, 22, 55});
int i = 0;
while (i < list.size()) {
if (i == 3)
return true;
System.out.println(list.get(i));
i++;
}
return false;
}
}
Output:
12
34
21
Loop Exit explicitly