How to Fix Error: Class, Interface, or Enum Expected in Java
- Understanding the Error
- Common Causes of the Error
- Solution: Check Your Code Structure
- Solution: Fix Bracket Issues
- Solution: Verify Method Declarations
- Conclusion
- FAQ
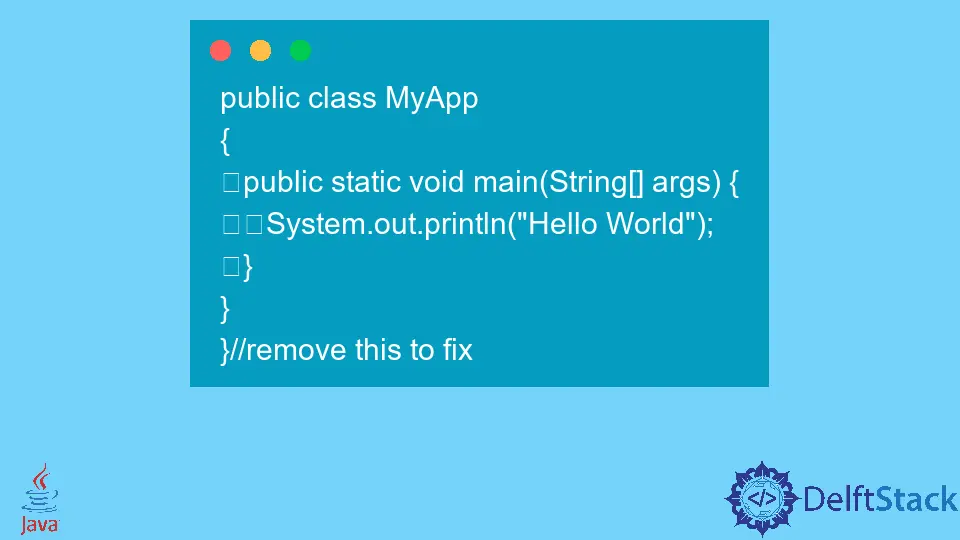
When programming in Java, encountering the “Class, Interface, or Enum Expected” error can be frustrating. This error typically arises when the Java compiler comes across code that doesn’t conform to the expected structure of a Java program. Whether it’s a misplaced bracket, an incomplete declaration, or a syntax error, pinpointing the issue can save you time and effort.
In this tutorial, we will explore the common causes of this error and how to fix it effectively. By the end of this article, you’ll have a clearer understanding of how to troubleshoot and resolve this pesky error, allowing you to write cleaner, more efficient Java code.
Understanding the Error
Before diving into solutions, it’s essential to grasp what triggers the “Class, Interface, or Enum Expected” error. This error often occurs when:
- You have code outside of a class or interface.
- There is a missing or misplaced bracket.
- You are trying to declare a variable or method in the wrong scope.
Recognizing these common pitfalls is the first step toward fixing the error.
Common Causes of the Error
-
Code Outside of Class or Interface: In Java, all executable code must reside within a class or interface. If you attempt to place code outside of these structures, you’ll encounter this error.
-
Missing or Misplaced Brackets: Java uses curly braces to define code blocks. If you forget to close a bracket or place it incorrectly, the compiler may misinterpret your code structure.
-
Incorrect Declarations: Declaring methods or variables in the wrong context can also trigger this error. For instance, you cannot declare a method directly in a class without proper syntax.
By understanding these common causes, you can more easily identify and rectify the issue.
Solution: Check Your Code Structure
The first step in resolving this error is to ensure that all your code is correctly structured within a class or interface. Here’s a simple example of a correctly structured Java program:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Output:
Hello, World!
In this example, the HelloWorld
class encapsulates the main
method, which is the entry point of the program. If you were to place the main
method outside of the HelloWorld
class, you would encounter the “Class, Interface, or Enum Expected” error.
Ensuring that your code is organized in this manner is crucial for avoiding the error. Always double-check that your methods and variables are declared within the appropriate classes.
Solution: Fix Bracket Issues
Another common cause of this error is missing or misplaced brackets. Java relies heavily on curly braces to define the beginning and end of classes, methods, and other code blocks. Here’s an example of code with a bracket issue:
public class Example {
public static void main(String[] args) {
System.out.println("Hello, World!");
// Missing closing bracket
Output:
error: class, interface, or enum expected
To fix this, you need to ensure that every opening curly brace {
has a corresponding closing curly brace }
. The corrected code looks like this:
public class Example {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Output:
Hello, World!
By ensuring that all brackets are correctly placed, you can avoid this common pitfall. A good practice is to use an IDE that highlights matching brackets and provides syntax checking.
Solution: Verify Method Declarations
Declaring methods incorrectly can lead to the “Class, Interface, or Enum Expected” error as well. For instance, if you try to declare a method outside of a class, you will encounter this error. Here’s an example of incorrect method declaration:
public void displayMessage() {
System.out.println("Hello!");
}
Output:
error: class, interface, or enum expected
To fix this, ensure that the method is placed inside a class. Here’s the corrected version:
public class Message {
public void displayMessage() {
System.out.println("Hello!");
}
}
Output:
Hello!
By ensuring that all methods are declared within a class, you can eliminate this source of error. Always remember that Java requires a structured format for all code.
Conclusion
Fixing the “Class, Interface, or Enum Expected” error in Java is often a matter of checking your code structure, verifying bracket placement, and ensuring correct method declarations. By understanding the common causes of this error and implementing the solutions discussed in this article, you can streamline your coding process and minimize frustrating compiler errors. Remember, the key to writing efficient Java code lies in maintaining a clear and organized structure.
FAQ
- What does the “Class, Interface, or Enum Expected” error mean?
This error indicates that the Java compiler has encountered code that is not correctly structured within a class or interface.
-
How can I prevent this error in the future?
Ensure that all your code is encapsulated within classes or interfaces and double-check your bracket placements. -
Can this error occur due to incorrect variable declarations?
Yes, declaring variables or methods outside of a class can trigger this error. -
What tools can help me avoid this error?
Using an Integrated Development Environment (IDE) with syntax highlighting and error checking can help identify issues before compilation. -
Is this error specific to Java?
While similar errors may occur in other programming languages, the specific message “Class, Interface, or Enum Expected” is unique to Java.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack