How to Check Empty String in Java
- Create Empty String in Java
- Create Empty String Using Apache Library in Java
-
Verify Empty
String
UsingisEmpty()
Method in Java -
Verify Empty
String
Usingequals()
Method in Java -
Verify Empty
String
UsingisBlank()
Method in Java
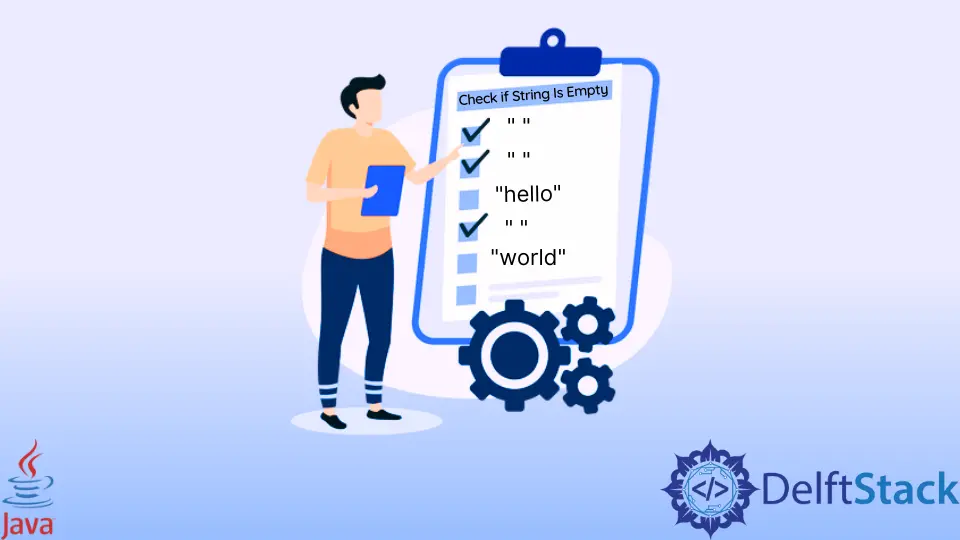
This tutorial introduces why there is no empty string constant in the Java String class and how to deal with an empty string in Java.
In Java, there is no constant that shows an empty string, and even the String
class does not have such provision. So far, to use empty string, we can assign it by using empty double-quotes.
In this article, we will learn to create an empty string and then validate it with various methods to check whether the string is really empty or not. We are going to use the apache commons library and Java 11 String isBlank()
method. Let’s start with some examples.
Create Empty String in Java
Let’s create an empty string by assigning an empty value to it by using the empty double-quotes. It is the simplest way to do this. Java does not provide any existing constant, so we used it.
public class SimpleTesting {
public static void main(String[] args) {
String empty_str = "";
System.out.println(empty_str);
}
}
Or we can create our own constant that refers to an empty string and then uses this constant in the code to create an empty string. In the code below, we created a static final string that holds an empty value and assigned it to the empty_str
variable.
It works fine and compiles successfully without any compilation error.
public class SimpleTesting {
private static final String EMPTY_STRING = "";
public static void main(String[] args) {
String empty_str = EMPTY_STRING;
System.out.println(empty_str);
}
}
Create Empty String Using Apache Library in Java
If you are working with the apache commons library, you can use the StringUtils
class with an Empty constant to create an empty string in Java. This class has a built-in empty constant so the programmer can directly use it in the code. See the example below.
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
String empty_str = StringUtils.EMPTY;
System.out.println(empty_str);
}
}
Verify Empty String
Using isEmpty()
Method in Java
After creating an empty string, we must verify it to check whether the string is really empty or not. For this purpose, we can use the isEmpty()
method of the String class that returns True for an empty value.
This example used the isEmpty()
method in the if
block to execute the code conditionally.
public class SimpleTesting {
private static final String EMPTY_STRING = "";
public static void main(String[] args) {
String empty_str = EMPTY_STRING;
if (empty_str.isEmpty()) {
System.out.println("String is empty");
} else
System.out.println("String is not empty");
}
}
Output:
String is empty
Verify Empty String
Using equals()
Method in Java
The equals()
method in Java is used to check whether two objects are equal or not. We can use this to check empty strings by calling on an empty string and passing the argument. See the example below.
public class SimpleTesting {
private static final String EMPTY_STRING = "";
public static void main(String[] args) {
String empty_str = EMPTY_STRING;
if ("".equals(empty_str)) {
System.out.println("String is empty");
} else
System.out.println("String is not empty");
}
}
Output:
String is empty
Verify Empty String
Using isBlank()
Method in Java
Java 11 added a new method, isBlank()
, to the String class. This method checks whether a string is empty or contains only white space codepoints. It returns true if the string is empty. We can use this to verify empty string. See the example below.
public class SimpleTesting {
private static final String EMPTY_STRING = "";
public static void main(String[] args) {
String empty_str = EMPTY_STRING;
if (empty_str.isBlank()) {
System.out.println("String is empty");
} else
System.out.println("String is not empty");
}
}
Output:
String is empty