在 Java 中检查空字符串
- 在 Java 中创建空字符串
- 在 Java 中使用 Apache 库创建空字符串
-
在 Java 中使用
isEmpty()
方法验证空String
-
在 Java 中使用
equals()
方法验证空String
-
在 Java 中使用
isBlank()
方法验证空String
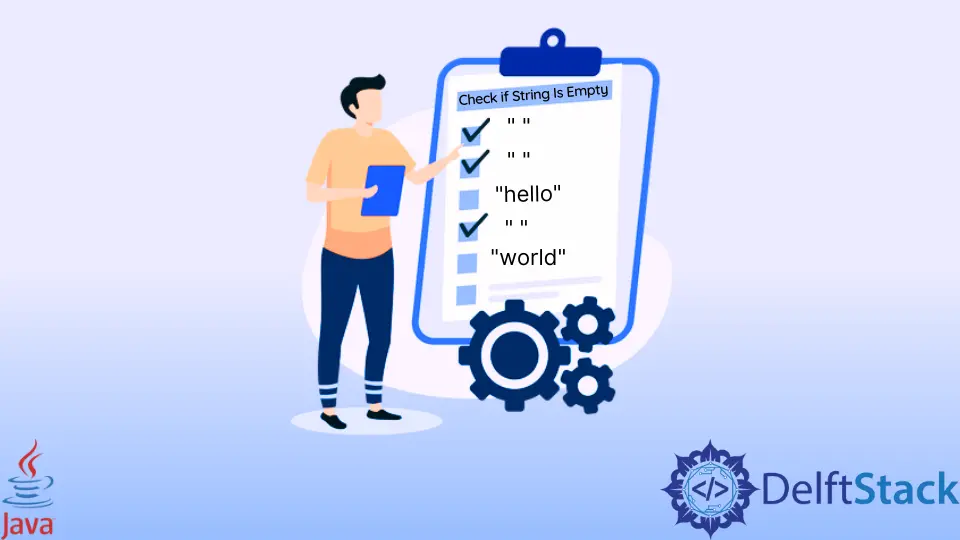
本教程介绍了 Java String 类中为什么没有空字符串常量以及 Java 中如何处理空字符串。
在 Java 中,没有显示空字符串的常量,甚至 String
类也没有这样的规定。到目前为止,要使用空字符串,我们可以使用空双引号来分配它。
在本文中,我们将学习创建一个空字符串,然后使用各种方法对其进行验证以检查该字符串是否真的为空。我们将使用 apache 公共库和 Java 11 String isBlank()
方法。让我们从一些例子开始。
在 Java 中创建空字符串
让我们通过使用空双引号为其分配一个空值来创建一个空字符串。这是最简单的方法。Java 没有提供任何现有的常量,所以我们使用了它。
public class SimpleTesting {
public static void main(String[] args) {
String empty_str = "";
System.out.println(empty_str);
}
}
或者我们可以创建我们自己的常量来引用一个空字符串,然后在代码中使用这个常量来创建一个空字符串。在下面的代码中,我们创建了一个静态最终字符串,其中包含一个空值并将其分配给 empty_str
变量。
它工作正常并且编译成功,没有任何编译错误。
public class SimpleTesting {
private static final String EMPTY_STRING = "";
public static void main(String[] args) {
String empty_str = EMPTY_STRING;
System.out.println(empty_str);
}
}
在 Java 中使用 Apache 库创建空字符串
如果你正在使用 apache 公共库,你可以使用带有 Empty 常量的 StringUtils
类在 Java 中创建一个空字符串。这个类有一个内置的空常量,所以程序员可以直接在代码中使用它。请参阅下面的示例。
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
String empty_str = StringUtils.EMPTY;
System.out.println(empty_str);
}
}
在 Java 中使用 isEmpty()
方法验证空 String
创建空字符串后,我们必须对其进行验证以检查该字符串是否真的为空。为此,我们可以使用 String 类的 isEmpty()
方法,该方法为空值返回 True。
此示例使用 if
块中的 isEmpty()
方法有条件地执行代码。
public class SimpleTesting {
private static final String EMPTY_STRING = "";
public static void main(String[] args) {
String empty_str = EMPTY_STRING;
if (empty_str.isEmpty()) {
System.out.println("String is empty");
} else
System.out.println("String is not empty");
}
}
输出:
String is empty
在 Java 中使用 equals()
方法验证空 String
Java 中的 equals()
方法用于检查两个对象是否相等。我们可以通过调用空字符串并传递参数来使用它来检查空字符串。请参阅下面的示例。
public class SimpleTesting {
private static final String EMPTY_STRING = "";
public static void main(String[] args) {
String empty_str = EMPTY_STRING;
if ("".equals(empty_str)) {
System.out.println("String is empty");
} else
System.out.println("String is not empty");
}
}
输出:
String is empty
在 Java 中使用 isBlank()
方法验证空 String
Java 11 向 String 类添加了一个新方法 isBlank()
。此方法检查字符串是否为空或仅包含空白代码点。如果字符串为空,则返回 true。我们可以使用它来验证空字符串。请参阅下面的示例。
public class SimpleTesting {
private static final String EMPTY_STRING = "";
public static void main(String[] args) {
String empty_str = EMPTY_STRING;
if (empty_str.isBlank()) {
System.out.println("String is empty");
} else
System.out.println("String is not empty");
}
}
输出:
String is empty