How to Validate Email in Java
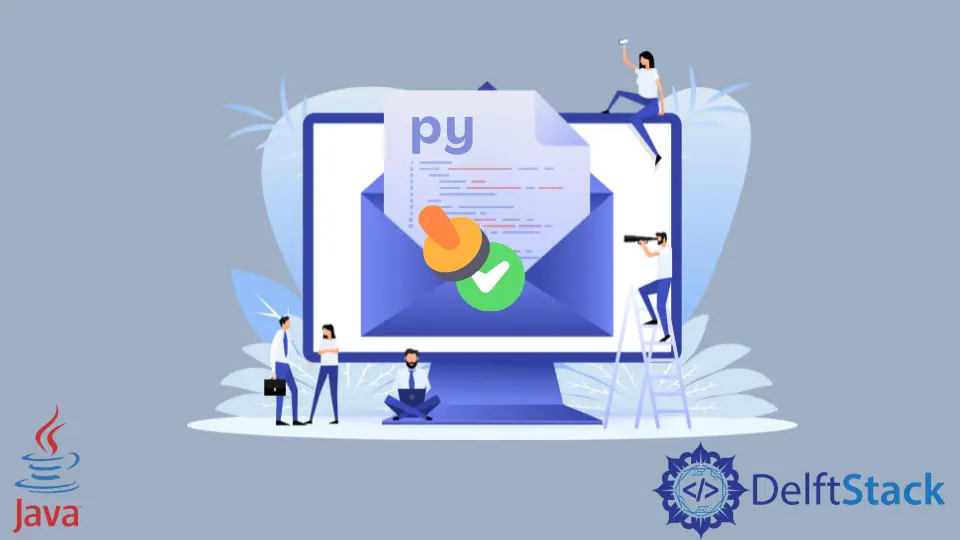
Before using any email, we have to validate it. Emails play a critical role in designing forms. Using the regular expression in Java, we can validate an email. We import the java.util.regex
package to work with regular expressions in Java.
Java provides us with some ways to use a regular expression to validate emails. Several regular expression patterns can help in validating emails in Java.
The first way is using the simplest regex. The ^(.+)@(.+)$
pattern is the simplest expression that checks the @
symbol in the emails. In this method, it will not care about the characters before and after the symbol. It will simply give false for that email.
The below code demonstrates the above method.
import java.util.*;
import java.util.regex.*;
public class Email_Validation {
public static void main(String args[]) {
ArrayList<String> email = new ArrayList<String>();
email.add("example@domain.com");
// Adding an invalid emails in list
email.add("@helloworld.com");
// Regular Expression
String regx = "^(.+)@(.+)$";
// Compile regular expression to get the pattern
Pattern pattern = Pattern.compile(regx);
// Iterate emails array list
for (String email1 : email) {
// Create instance of matcher
Matcher matcher = pattern.matcher(email1);
System.out.println(email1 + " : " + matcher.matches() + "\n");
}
}
}
Output:
example@domain.com : true
@helloworld.com : false
In the above example, we created a list of emails containing valid and invalid emails. We use the matcher
class to create a method that checks whether a given email matches the pattern or not.
The second pattern is used for adding a restriction on the user name part method. Here [A-Za-z0-9+_.-]+@(.+)$
is the regular expression used for validating the email. It checks the username part of the email, and according to it, validates the email. There are some rules for this method. The username can have A-Z characters, a-z characters, 0-9 numbers, dot(.
), underscore(_
). Username having characters other than the above mentioned, then it will not be validated.
For example,
import java.util.*;
import java.util.regex.*;
public class Email_Validation {
public static void main(String args[]) {
ArrayList<String> email = new ArrayList<String>();
email.add("example@domain.com");
email.add("example2@domain.com");
// Adding an invalid emails in list
email.add("@helloworld.com");
email.add("12Bye#domain.com");
// Regular Expression
String regx = "^[A-Za-z0-9+_.-]+@(.+)$";
// Compile regular expression to get the pattern
Pattern pattern = Pattern.compile(regx);
// Iterate emails array list
for (String email1 : email) {
// Create instance of matcher
Matcher matcher = pattern.matcher(email1);
System.out.println(email1 + " : " + matcher.matches() + "\n");
}
}
}