Java에서 이메일 검증
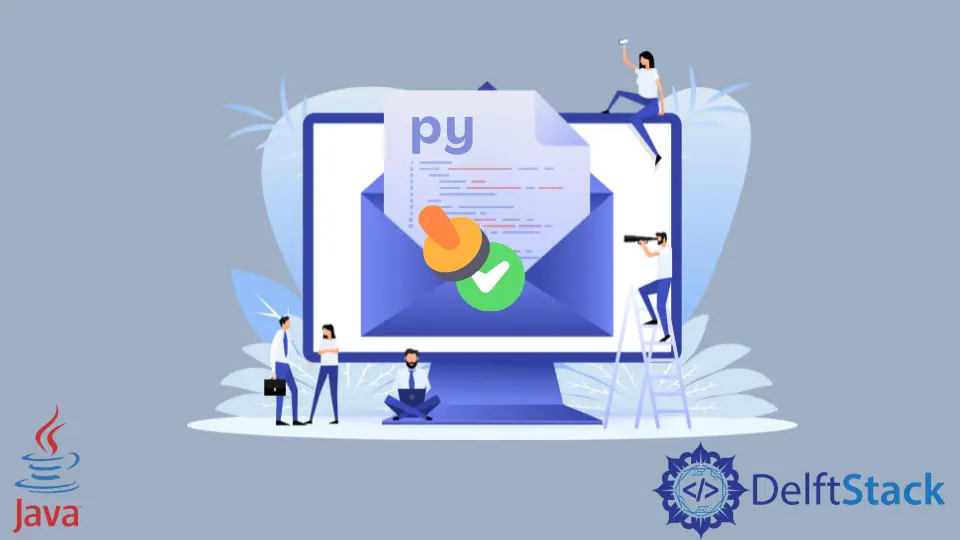
이메일을 사용하기 전에 이메일을 확인해야 합니다. 이메일은 양식을 디자인하는 데 중요한 역할을 합니다. Java의 정규식을 사용하여 이메일을 확인할 수 있습니다. Java에서 정규식으로 작업하기 위해 java.util.regex
패키지를 가져옵니다.
Java는 정규식을 사용하여 이메일을 검증하는 몇 가지 방법을 제공합니다. 여러 정규식 패턴은 Java에서 이메일을 검증하는 데 도움이 될 수 있습니다.
첫 번째 방법은 가장 간단한 정규식을 사용하는 것입니다. ^(.+)@(.+)$
패턴은 이메일에서 @
기호를 확인하는 가장 간단한 표현식입니다. 이 방법에서는 기호 앞과 뒤의 문자를 신경 쓰지 않습니다. 해당 이메일에 대해 단순히 false를 제공합니다.
아래 코드는 위의 방법을 보여줍니다.
import java.util.*;
import java.util.regex.*;
public class Email_Validation {
public static void main(String args[]) {
ArrayList<String> email = new ArrayList<String>();
email.add("example@domain.com");
// Adding an invalid emails in list
email.add("@helloworld.com");
// Regular Expression
String regx = "^(.+)@(.+)$";
// Compile regular expression to get the pattern
Pattern pattern = Pattern.compile(regx);
// Iterate emails array list
for (String email1 : email) {
// Create instance of matcher
Matcher matcher = pattern.matcher(email1);
System.out.println(email1 + " : " + matcher.matches() + "\n");
}
}
}
출력:
example@domain.com : true
@helloworld.com : false
위의 예에서는 유효한 이메일과 잘못된 이메일이 포함된 이메일 목록을 만들었습니다. matcher
클래스를 사용하여 주어진 이메일이 패턴과 일치하는지 여부를 확인하는 메서드를 만듭니다.
두 번째 패턴은 사용자 이름 부분 방식에 대한 제한을 추가하는 데 사용됩니다. 여기 [A-Za-z0-9+_.-]+@(.+)$
는 이메일 유효성 검사에 사용되는 정규식입니다. 이메일의 사용자 이름 부분을 확인하고 그에 따라 이메일의 유효성을 검사합니다. 이 방법에는 몇 가지 규칙이 있습니다. 사용자 이름은 A-Z 문자, a-z 문자, 0-9 숫자, 점(.
), 밑줄(_
)을 사용할 수 있습니다. 위에 언급된 문자가 아닌 다른 문자가 포함된 사용자 이름은 검증되지 않습니다.
예를 들어,
import java.util.*;
import java.util.regex.*;
public class Email_Validation {
public static void main(String args[]) {
ArrayList<String> email = new ArrayList<String>();
email.add("example@domain.com");
email.add("example2@domain.com");
// Adding an invalid emails in list
email.add("@helloworld.com");
email.add("12Bye#domain.com");
// Regular Expression
String regx = "^[A-Za-z0-9+_.-]+@(.+)$";
// Compile regular expression to get the pattern
Pattern pattern = Pattern.compile(regx);
// Iterate emails array list
for (String email1 : email) {
// Create instance of matcher
Matcher matcher = pattern.matcher(email1);
System.out.println(email1 + " : " + matcher.matches() + "\n");
}
}
}
출력:
example@domain.com : true
example2@domain.com : true
@helloworld.com : false
12Bye#domain.com : false
이메일 유효성 검사를 위한 세 번째 패턴은 RFC 5322 방법에 의해 허용됩니다. ^[a-zA-Z0-9_!#$%&'\*+/=?{|}~^.-]+@[a-zA-Z0-9.-]+$
는 일반 이메일 유효성 검사에 사용되는 표현식입니다. RFC에서 이메일 형식에 허용하는 모든 문자가 사용됩니다.
예를 들어,
import java.util.*;
import java.util.regex.*;
public class Email_Validation {
public static void main(String args[]) {
ArrayList<String> email = new ArrayList<String>();
email.add("example@domain.com");
email.add("exampletwo@domain.com");
email.add("12@domain.com");
// Adding an invalid emails in list
email.add("@helloworld.com");
// Regular Expression
String regx = "^[a-zA-Z0-9_!#$%&'*+/=?`{|}~^.-]+@[a-zA-Z0-9.-]+$";
// Compile regular expression to get the pattern
Pattern pattern = Pattern.compile(regx);
// Iterate emails array list
for (String email1 : email) {
// Create instance of matcher
Matcher matcher = pattern.matcher(email1);
System.out.println(email1 + " : " + matcher.matches() + "\n");
}
}
}
출력:
example@domain.com : true
exampletwo@domain.com : true
12@domain.com : true
@helloworld.com : false