Java 中的電子郵件驗證
Siddharth Swami
2023年10月12日
Java
Java Email
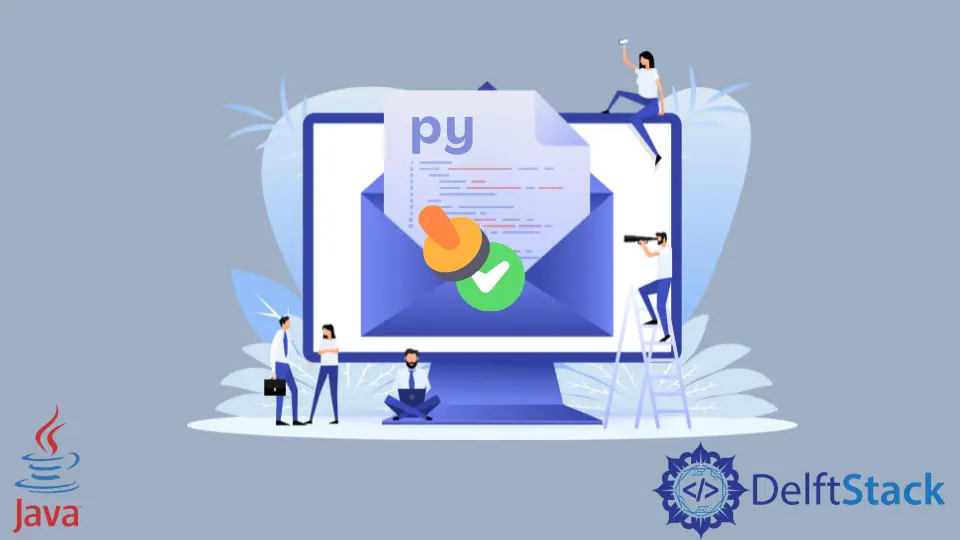
在使用任何電子郵件之前,我們必須對其進行驗證。電子郵件在設計表單中起著至關重要的作用。使用 Java 中的正規表示式,我們可以驗證電子郵件。我們匯入 java.util.regex
包來處理 Java 中的正規表示式。
Java 為我們提供了一些使用正規表示式來驗證電子郵件的方法。幾種正規表示式模式可以幫助驗證 Java 中的電子郵件。
第一種方法是使用最簡單的正規表示式。^(.+)@(.+)$
模式是檢查電子郵件中@
符號的最簡單的表示式。在這種方法中,它不會關心符號前後的字元。它只會為該電子郵件提供 false。
下面的程式碼演示了上述方法。
import java.util.*;
import java.util.regex.*;
public class Email_Validation {
public static void main(String args[]) {
ArrayList<String> email = new ArrayList<String>();
email.add("example@domain.com");
// Adding an invalid emails in list
email.add("@helloworld.com");
// Regular Expression
String regx = "^(.+)@(.+)$";
// Compile regular expression to get the pattern
Pattern pattern = Pattern.compile(regx);
// Iterate emails array list
for (String email1 : email) {
// Create instance of matcher
Matcher matcher = pattern.matcher(email1);
System.out.println(email1 + " : " + matcher.matches() + "\n");
}
}
}
輸出:
example@domain.com : true
@helloworld.com : false
在上面的例子中,我們建立了一個包含有效和無效電子郵件的電子郵件列表。我們使用 matcher
類來建立一個方法來檢查給定的電子郵件是否與模式匹配。
第二種模式用於對使用者名稱部分方法新增限制。這裡 [A-Za-z0-9+_.-]+@(.+)$
是用於驗證電子郵件的正規表示式。它檢查電子郵件的使用者名稱部分,並根據它驗證電子郵件。這種方法有一些規則。使用者名稱可以包含 A-Z 字元、a-z 字元、0-9 數字、點(.
)、下劃線(_
)。使用者名稱包含上述以外的字元,則不會被驗證。
例如,
import java.util.*;
import java.util.regex.*;
public class Email_Validation {
public static void main(String args[]) {
ArrayList<String> email = new ArrayList<String>();
email.add("example@domain.com");
email.add("example2@domain.com");
// Adding an invalid emails in list
email.add("@helloworld.com");
email.add("12Bye#domain.com");
// Regular Expression
String regx = "^[A-Za-z0-9+_.-]+@(.+)$";
// Compile regular expression to get the pattern
Pattern pattern = Pattern.compile(regx);
// Iterate emails array list
for (String email1 : email) {
// Create instance of matcher
Matcher matcher = pattern.matcher(email1);
System.out.println(email1 + " : " + matcher.matches() + "\n");
}
}
}
輸出:
example@domain.com : true
example2@domain.com : true
@helloworld.com : false
12Bye#domain.com : false
RFC 5322 方法允許用於電子郵件驗證的第三種模式。^[a-zA-Z0-9_!#$%&'\*+/=?{|}~^.-]+@[a-zA-Z0-9.-]+$
是常規的用於驗證電子郵件的表示式。使用了 RFC 允許用於電子郵件格式的所有字元。
例如,
import java.util.*;
import java.util.regex.*;
public class Email_Validation {
public static void main(String args[]) {
ArrayList<String> email = new ArrayList<String>();
email.add("example@domain.com");
email.add("exampletwo@domain.com");
email.add("12@domain.com");
// Adding an invalid emails in list
email.add("@helloworld.com");
// Regular Expression
String regx = "^[a-zA-Z0-9_!#$%&'*+/=?`{|}~^.-]+@[a-zA-Z0-9.-]+$";
// Compile regular expression to get the pattern
Pattern pattern = Pattern.compile(regx);
// Iterate emails array list
for (String email1 : email) {
// Create instance of matcher
Matcher matcher = pattern.matcher(email1);
System.out.println(email1 + " : " + matcher.matches() + "\n");
}
}
}
輸出:
example@domain.com : true
exampletwo@domain.com : true
12@domain.com : true
@helloworld.com : false
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe