How to Create Timer in JavaFx
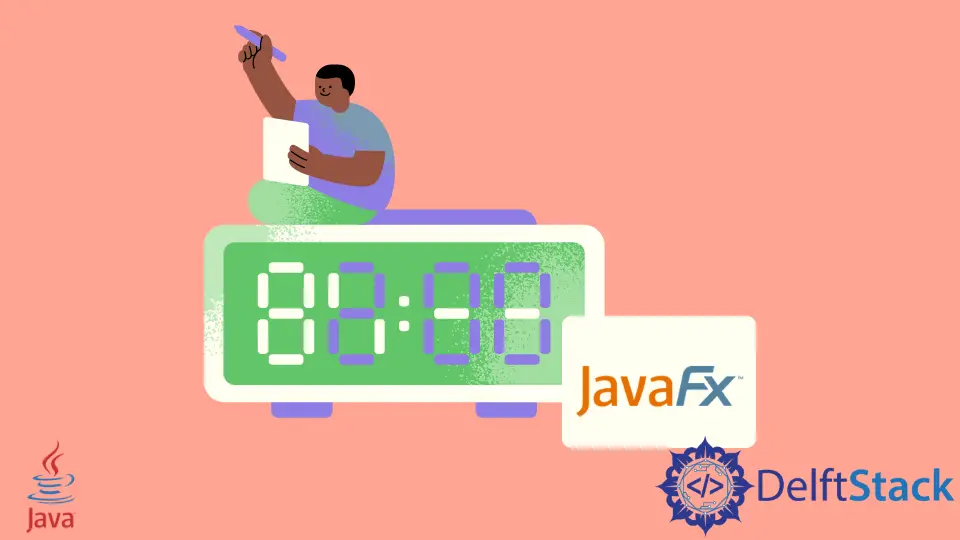
In Java, a need may arise for certain scheduled tasks to be performed later or with a delay. We shall perform this by adding a timer to Java code.
To add a timer to the code, we shall use the Timer
class and the TimerTask
class from the java.util
package.
To use the java.util.Timer
class, we first need to import it. Use the import
keyword followed by the desired class for this purpose.
import java.util.Timer;
Similarly, to use the TimerTask
class, we first need to import it. We use the import
keyword followed by the desired class for this purpose.
We import the java.util.TimerTask
class in the Java project.
import java.util.TimerTask;
We want to schedule a simple task to print the Hello World message within 5 seconds.
Let us look at the code for performing this, followed by a section-by-section explanation of the given code along with the output.
Create Timer in JavaFX
In this example, we first import the Timer
and TimerTask
classes, and then inside the main()
method, we created an object of the Timer
class and an anonymous inner class to perform a task.
Since the TimerTask
class implements the Runnable
interface, we override the run()
method to perform the task and then use the schedule()
method.
We run the task. The schedule()
method takes two arguments, the first is the task object, and the second is the delay time.
See the example below.
import java.util.Timer;
import java.util.TimerTask;
public class SimpleTesting {
public static void main(String[] args) {
Timer timer = new Timer();
TimerTask task = new TimerTask() {
public void run() {
// The task you want to do
System.out.println("Hello World");
}
};
timer.schedule(task, 5000l);
}
}
Output:
Hello World
The main part of scheduling the task is performed using the timer.schedule()
method of the Timer
class. The signature of this function is as follows:
public void schedule(TimerTask task, long delay)
It schedules the specified task for execution after the specified delay.
The parameters are task, scheduled and delayed, and delay in milliseconds before execution.
The possible exceptions it throws are IllegalArgumentException
if the delay is negative, or delay + System.currentTimeMillis()
is negative.
The IllegalStateException
, if the task was already scheduled or canceled, the timer was canceled, or timer thread terminated, NullPointerException
if the task is null.
We write timer.schedule(task, 5000l)
to schedule the task, the first parameter is the previously defined task object, and the second parameter is the required delay.
Since we need a delay of 5 seconds, equal to 5000 milliseconds, we pass 5000l
as the second parameter.
The l
denotes a long data type. As a result, the Hello World
message is printed in output after a delay of 5 seconds or 5000 milliseconds.
We can perform more complicated tasks by replacing the print statement with the required statements.
Conclusion
In this topic, we have learned to add a timer to a Java program following an example to schedule a simple task for printing, giving us a feel of how this concept could be applied in real-world use cases.