How to Create swing timer in Java
- Understanding Swing Timer
- Creating a Basic Swing Timer
- Customizing Timer Behavior
- Using Timer for Animation
- Conclusion
- FAQ
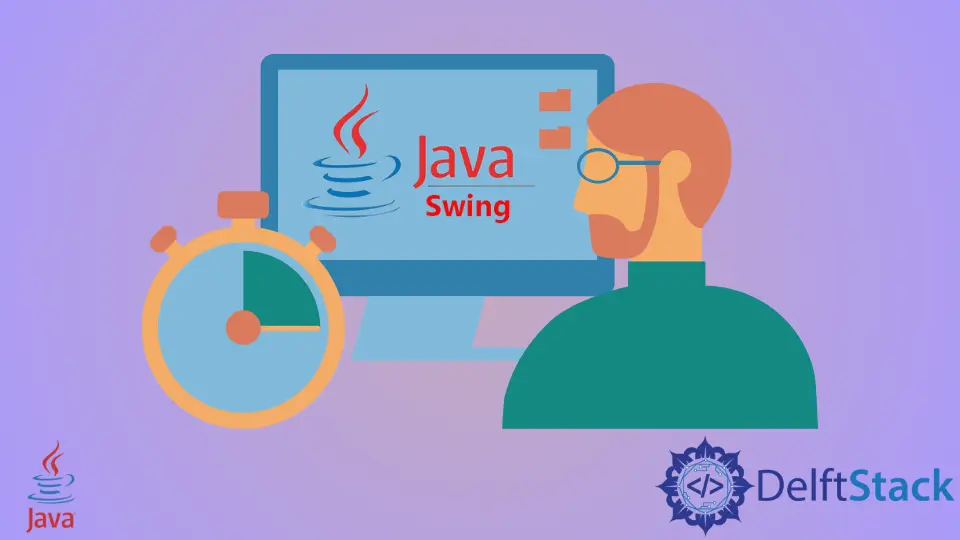
Creating a Swing timer in Java can greatly enhance your application’s interactivity and responsiveness. Whether you’re developing a simple clock, an animation, or a game, a timer can help manage time-based events effectively.
In this article, we will explore the concept of the Swing timer in Java, how it works, and provide clear, concise code examples to get you started. You’ll learn how to utilize the javax.swing.Timer
class to perform tasks at specified intervals, making your Java applications more dynamic and engaging. Let’s dive into the world of Swing timers and see how you can implement them in your projects.
Understanding Swing Timer
Before we jump into coding, it’s essential to understand what a Swing timer is and how it fits into the Java ecosystem. The Swing timer is a class that is part of the javax.swing
package, designed to generate action events at specified intervals. Unlike traditional timers, which can block the main thread, Swing timers run on the Event Dispatch Thread (EDT), ensuring that your user interface remains responsive. This is crucial for applications with graphical user interfaces (GUIs), as it prevents freezing or lagging during time-consuming tasks.
To create a Swing timer, you typically need to specify two main parameters: the delay (in milliseconds) and an ActionListener
that defines what happens when the timer ticks. This makes it easy to schedule periodic tasks without complicating your code.
Creating a Basic Swing Timer
Let’s start with a straightforward example of creating a Swing timer that updates a label every second. This example will help you grasp the fundamentals of using the javax.swing.Timer
class.
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class BasicSwingTimer {
public static void main(String[] args) {
JFrame frame = new JFrame("Swing Timer Example");
JLabel label = new JLabel("Timer: 0");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 200);
frame.add(label);
frame.setVisible(true);
Timer timer = new Timer(1000, new ActionListener() {
int count = 0;
@Override
public void actionPerformed(ActionEvent e) {
count++;
label.setText("Timer: " + count);
}
});
timer.start();
}
}
Output:
Timer: 1
Timer: 2
Timer: 3
...
In this example, we create a simple JFrame with a JLabel that displays the timer’s value. The Timer
is initialized with a delay of 1000 milliseconds (1 second) and an ActionListener
that increments a counter and updates the label each time the timer ticks. The start()
method begins the timer, and it will continue to run until it is stopped or the application is closed.
Customizing Timer Behavior
Now that you’ve created a basic Swing timer, let’s explore how to customize its behavior. You can modify the timer’s delay, stop and start it dynamically, or even create multiple timers for different tasks. Here’s an example that demonstrates these features.
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class CustomSwingTimer {
public static void main(String[] args) {
JFrame frame = new JFrame("Custom Swing Timer");
JButton startButton = new JButton("Start Timer");
JButton stopButton = new JButton("Stop Timer");
JLabel label = new JLabel("Timer: 0");
frame.setLayout(new java.awt.FlowLayout());
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 200);
frame.add(startButton);
frame.add(stopButton);
frame.add(label);
frame.setVisible(true);
Timer timer = new Timer(1000, new ActionListener() {
int count = 0;
@Override
public void actionPerformed(ActionEvent e) {
count++;
label.setText("Timer: " + count);
}
});
startButton.addActionListener(e -> timer.start());
stopButton.addActionListener(e -> timer.stop());
}
}
Output:
Timer: 1
Timer: 2
Timer: 3
...
In this example, we’ve added two buttons to start and stop the timer. Clicking the “Start Timer” button begins the timer, while the “Stop Timer” button halts it. This flexibility allows users to control the timer based on their needs, making your application more interactive.
Using Timer for Animation
Swing timers are also excellent for creating animations. You can use the timer to update graphics on the screen at regular intervals. Here’s an example that demonstrates how to animate a simple moving component.
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class AnimationWithSwingTimer {
private static int x = 0;
public static void main(String[] args) {
JFrame frame = new JFrame("Animation Example");
frame.setSize(400, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Timer timer = new Timer(10, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
x += 2;
if (x > frame.getWidth()) {
x = 0;
}
frame.repaint();
}
});
frame.add(new JPanel() {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.fillOval(x, 100, 30, 30);
}
});
frame.setVisible(true);
timer.start();
}
}
Output:
(An animated circle moving across the screen)
In this animation example, we create a JFrame and override the paintComponent
method of a JPanel to draw a circle. The timer updates the circle’s position every 10 milliseconds, creating a smooth animation effect. When the circle moves off the screen, it resets to the starting position, providing a continuous loop of movement.
Conclusion
Swing timers in Java are a powerful tool for managing time-based events in GUI applications. By using the javax.swing.Timer
class, you can create responsive interfaces that enhance user experience. Whether you’re building simple applications or complex animations, understanding how to implement and customize Swing timers is essential for any Java developer. With the examples provided in this article, you should now have a solid foundation to start experimenting with timers in your own projects. Happy coding!
FAQ
-
What is a Swing timer?
A Swing timer is a class in Java that generates action events at specified intervals, allowing for time-based tasks in GUI applications. -
How do I stop a Swing timer?
You can stop a Swing timer by calling thestop()
method on the timer instance. -
Can a Swing timer run multiple tasks?
Yes, you can create multiple Swing timers, each with its own action listener to perform different tasks. -
Is the Swing timer thread-safe?
Yes, Swing timers run on the Event Dispatch Thread (EDT), which makes them thread-safe for updating GUI components. -
Can I use Swing timers for animations?
Absolutely! Swing timers are ideal for creating smooth animations by updating graphics at regular intervals.