How to Create Label Text Color in Java With JavaFx Library
-
Use the
setStyle()
Method to Color Texts Label in Java - Alternative Way to Change the Label Text Color
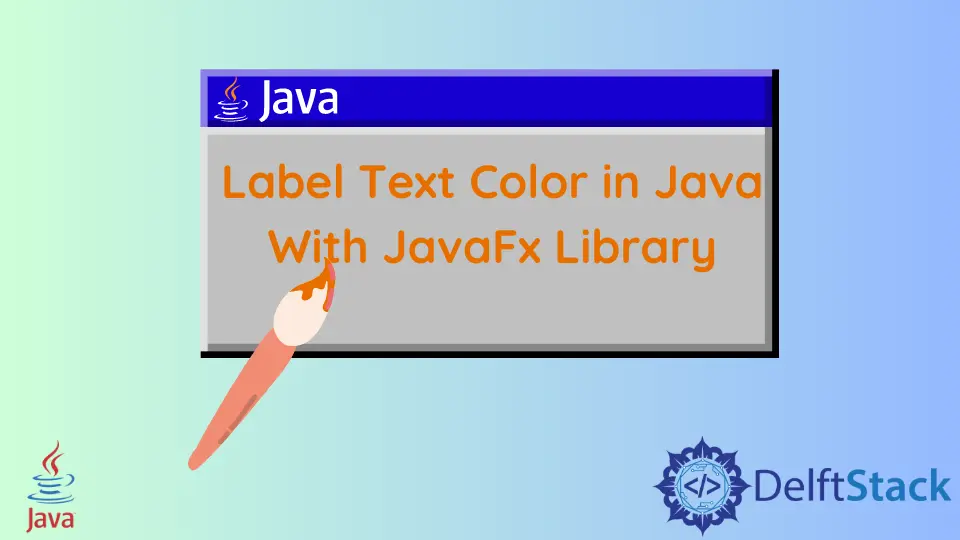
Sometimes we need to colorize the texts to make them focused on users. In JavaFX, we can do this very easily by including the setStyle()
method.
In this article, we are going to see how we can change the label’s text color, and we also see a necessary example with a proper explanation so that the topic is much easier to understand.
Use the setStyle()
Method to Color Texts Label in Java
In our below example, we just set the color of the text to read and the background color to yellow. First, we import the following JavaFx libraries needed to make it work.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.stage.Stage;
Full Source Code:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.stage.Stage;
public class FXlabel extends Application {
public void start(Stage PrimaryStage) throws Exception {
PrimaryStage.setTitle("Label Color"); // Setting the application title
Label lbl = new Label("This is the colored text !!!"); // Creating a simple label with a text
// "This is the colored text !!!"
lbl.setStyle(
"-fx-text-fill: red; -fx-background-color: yellow"); // Styling the text by applying
// necessary CSS properties.
Scene scene = new Scene(lbl, 200, 100); // Creating a scene
PrimaryStage.setScene(scene); // Setting the scene to stage
PrimaryStage.show(); // Make the stage visible
}
public static void main(String[] args) {
Application.launch(args); // Launching the application
}
}
In the line lbl.setStyle("-fx-text-fill: red; -fx-background-color: yellow");
, we applied some additional CSS properties to the label by using setStyle()
method. In detail, the first property we used here is -fx-text-fill: red;
through which we set the text color to red, and the property -fx-background-color: yellow
is used to set the background color to yellow.
After compiling the above example code and running it in your environment, you will get the below output.
Output:
Alternative Way to Change the Label Text Color
JavaFX supports CSS that works will FXML. Now, when designing the User Interface with JavaFX GUI building tool like Scene Builder provided by Oracle, You can easily define the text color with the CSS property while developing the UI.
Also, you can add a CSS file on which you can add below two properties.
-fx-text-fill: red;
-fx-background-color: yellow;
You can use the code below to include your CSS file in your code directly.
Code:
scene.getStylesheets().add("YourCSS.css")
Remember, if your IDE doesn’t support the automatic inclusion of Libraries and Packages. Then you may need to manually include these necessary Libraries and Packages before compiling.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn