The yield Keyword in C#
- Understanding the Yield Keyword
- Benefits of Using Yield in C#
- Common Use Cases for Yield in C#
- Conclusion
- FAQ
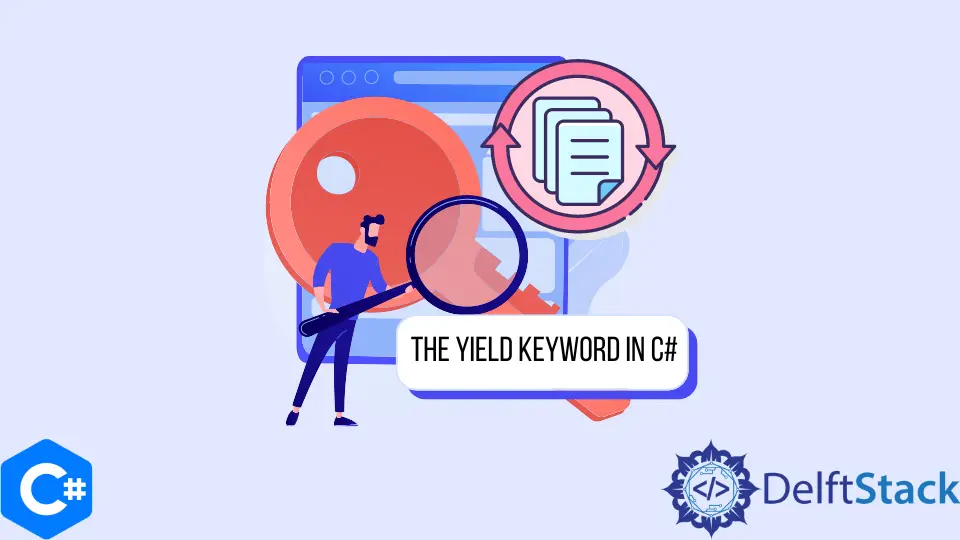
The yield keyword in C# is a powerful feature that allows developers to create iterators in a clean and efficient manner. By specifying that a function or an operator is an iterator, yield simplifies the process of returning a sequence of values one at a time, without the overhead of creating a temporary collection. This not only enhances performance but also improves code readability.
In this article, we will explore the yield keyword, how it works, and its practical applications in C#. Whether you’re a seasoned developer or just starting out, understanding yield can significantly enhance your programming toolkit.
Understanding the Yield Keyword
The yield keyword is used within an iterator method to produce a sequence of values. When a method contains the yield statement, it becomes an iterator, allowing you to iterate through the returned collection without needing to create and manage the entire collection in memory at once. This is particularly useful when dealing with large datasets or when the values are generated on-the-fly.
When you use yield, the state of the method is preserved between calls. This means that the next time the iterator is called, it resumes execution from where it left off. This behavior enables a more efficient use of resources, as only the necessary elements are generated and returned.
Here’s a simple example to illustrate how the yield keyword works:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
foreach (var number in GetNumbers())
{
Console.WriteLine(number);
}
}
static IEnumerable<int> GetNumbers()
{
for (int i = 1; i <= 5; i++)
{
yield return i;
}
}
}
Output:
1
2
3
4
5
In this example, the GetNumbers
method uses the yield keyword to return integers from 1 to 5. When the method is called, it returns an IEnumerable<int>
that can be iterated over. Each time the loop iterates, the method resumes execution and yields the next number, demonstrating the efficiency of using yield in C#.
Benefits of Using Yield in C#
Using the yield keyword in C# offers several advantages that can improve both performance and code maintainability. Here are some key benefits:
-
Memory Efficiency: Since yield generates values on-the-fly, you don’t need to allocate memory for the entire collection upfront. This is particularly beneficial when working with large datasets or streams of data.
-
Simplified Code: The use of yield can significantly reduce the complexity of your code. Instead of managing the state of your collection manually, yield handles it for you, allowing for cleaner and more readable code.
-
Lazy Evaluation: Yield supports lazy evaluation, meaning that values are produced only when requested. This can lead to improved performance, especially in scenarios where not all values are needed at once.
-
State Preservation: The method maintains its state between calls, allowing for more intuitive control over the iteration process. This feature can be particularly useful in scenarios involving complex data generation.
Here’s a more complex example that showcases some of these benefits:
using System;
using System.Collections.Generic;
class Fibonacci
{
public static void Main()
{
foreach (var number in GenerateFibonacci(10))
{
Console.WriteLine(number);
}
}
static IEnumerable<int> GenerateFibonacci(int count)
{
int a = 0, b = 1;
for (int i = 0; i < count; i++)
{
yield return a;
int temp = a;
a = b;
b = temp + b;
}
}
}
Output:
0
1
1
2
3
5
8
13
21
34
In this example, the GenerateFibonacci
method uses yield to produce Fibonacci numbers up to a specified count. The method maintains its state and efficiently generates each number only when requested, demonstrating the power of the yield keyword in C#.
Common Use Cases for Yield in C#
The yield keyword can be applied in various scenarios, making it a versatile tool for developers. Here are some common use cases:
-
Data Streaming: Yield is particularly useful when dealing with data streams, such as reading large files or processing data from a database. You can yield results as they are fetched, minimizing memory usage.
-
Infinite Sequences: When generating potentially infinite sequences, such as prime numbers or mathematical series, yield allows you to produce values without worrying about memory constraints.
-
Custom Collections: If you’re creating a custom collection class, yield can simplify the implementation of the
GetEnumerator
method, providing an easy way to iterate over the collection.
Here’s an example of a custom collection using yield:
using System;
using System.Collections;
using System.Collections.Generic;
class CustomCollection : IEnumerable<int>
{
private List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
public IEnumerator<int> GetEnumerator()
{
foreach (var number in numbers)
{
yield return number;
}
}
IEnumerator IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
}
class Program
{
static void Main()
{
var collection = new CustomCollection();
foreach (var number in collection)
{
Console.WriteLine(number);
}
}
}
Output:
1
2
3
4
5
In this example, the CustomCollection
class implements IEnumerable<int>
and uses yield to return its elements. This approach simplifies the iteration process and enhances code clarity.
Conclusion
The yield keyword in C# is a powerful feature that enhances the way developers create iterators. By allowing for lazy evaluation and state preservation, yield simplifies code and improves memory efficiency. Whether you’re generating sequences, creating custom collections, or streaming data, the yield keyword is a valuable addition to your programming toolkit. Understanding and utilizing yield can lead to cleaner, more efficient code, making it an essential concept for any C# developer.
FAQ
-
What is the yield keyword in C#?
The yield keyword is used to specify that a function or an operator is an iterator, allowing for the generation of a sequence of values on-the-fly. -
How does yield improve memory efficiency?
Yield generates values as they are requested, eliminating the need to store the entire collection in memory, which is especially beneficial for large datasets. -
Can I use yield with infinite sequences?
Yes, yield is particularly useful for generating infinite sequences, as it produces values only when needed, avoiding memory overflow. -
What are some common use cases for yield?
Common use cases include data streaming, generating infinite sequences, and simplifying custom collection implementations. -
How does yield affect code readability?
By reducing complexity and handling state management automatically, yield enhances code readability and maintainability.
#, a powerful feature for creating iterators. Learn how yield enhances memory efficiency, simplifies code, and supports lazy evaluation. Discover practical examples and common use cases to improve your C# programming skills.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn