The nameof Expression in C#
- Understanding the nameof Expression
- Using nameof with Variables
- Using nameof with Methods and Events
- Using nameof with Properties
- Conclusion
- FAQ
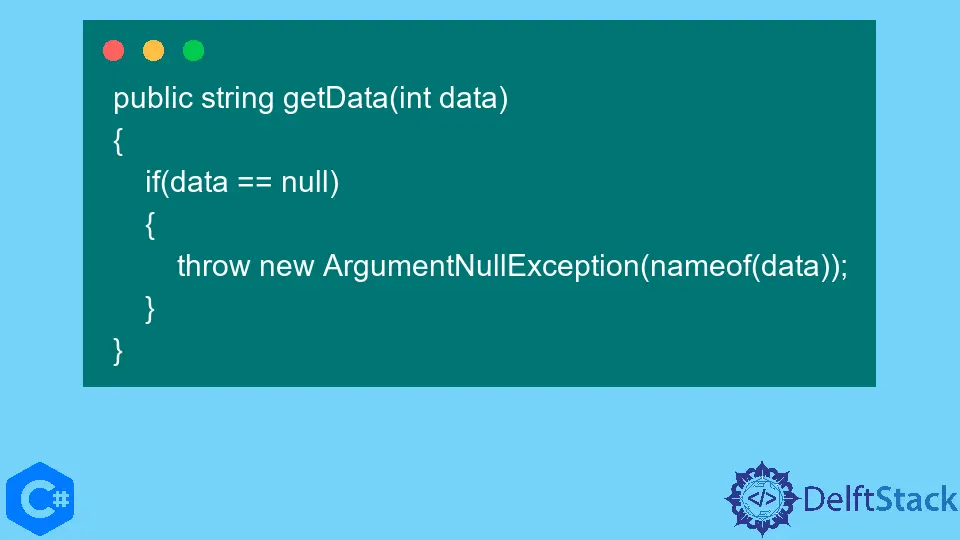
The nameof expression in C# is a powerful feature that allows developers to retrieve the name of variables, types, fields, or members as strings. This can be particularly useful in various scenarios, such as when implementing logging, validation, or when you need to provide meaningful error messages. By using nameof, you can avoid hardcoding strings, which can lead to potential errors during refactoring.
This article will explore the nameof expression in detail, providing practical examples and insights on how to effectively use it in your C# projects. Whether you’re a seasoned developer or just starting out, understanding how to leverage this feature can enhance your coding practices and improve the maintainability of your code.
Understanding the nameof Expression
The nameof expression was introduced in C# 6.0 and serves a simple yet significant purpose. It allows you to obtain the name of a variable, type, or member as a string without using quotes. This feature is particularly beneficial for reducing errors caused by typos in string literals. For instance, if you rename a variable, the nameof expression automatically reflects that change, eliminating the need for manual updates throughout your code.
Here’s a quick example to illustrate its usage:
string myVariable = "Hello, World!";
string variableName = nameof(myVariable);
Output:
myVariable
In this example, the variableName will hold the string “myVariable”. This demonstrates how you can easily obtain the name of the variable without worrying about string literals. The nameof expression can be used with methods, properties, and even classes, making it a versatile tool in your C# toolkit.
Using nameof with Variables
One of the most common uses of the nameof expression is to retrieve the names of variables. This can be particularly useful in scenarios where you want to log variable names for debugging purposes or create validation frameworks where you need to reference property names dynamically.
Consider the following code snippet:
public class Person
{
public string Name { get; set; }
public void PrintName()
{
Console.WriteLine(nameof(Name));
}
}
Output:
Name
In this example, the PrintName method uses nameof to print the name of the property “Name”. If you were to rename the property to “FullName”, the output would automatically update, ensuring consistency across your codebase. This reduces the risk of errors and enhances code readability, as the intent is clear.
Using nameof with variables can also streamline your unit tests. For instance, if you are validating properties, you can use nameof to specify which property is being validated without hardcoding its name. This makes your tests more maintainable and less prone to errors.
Using nameof with Methods and Events
The nameof expression is not limited to just variables; it can also be applied to methods and events. This can be particularly helpful in scenarios such as event handling, where you may want to pass the name of an event or method as a string.
Here’s an example demonstrating this:
public class Notifier
{
public event EventHandler Notified;
public void Notify()
{
Notified?.Invoke(this, EventArgs.Empty);
Console.WriteLine(nameof(Notified));
}
}
Output:
Notified
In this case, when the Notify method is called, it invokes the Notified event and prints its name using nameof. This can be particularly useful for logging purposes, allowing you to track which events are being triggered without hardcoding their names.
Moreover, using nameof in this context helps maintain a clean separation of concerns. If you later decide to change the event name, the nameof expression will automatically reflect that change throughout your code, ensuring that your logging or event handling remains accurate.
Using nameof with Properties
When working with properties in C#, the nameof expression can help streamline code that involves property change notifications. This is especially common in scenarios involving data binding, such as in WPF or Xamarin applications.
Here’s how you can use nameof with properties:
public class Employee : INotifyPropertyChanged
{
private string _name;
public string Name
{
get { return _name; }
set
{
_name = value;
OnPropertyChanged(nameof(Name));
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
Output:
Name
In this example, the OnPropertyChanged method is called whenever the Name property is set. By using nameof(Name), you ensure that the property name is always accurate, even if you decide to rename the property later. This practice enhances the maintainability of your code, as it reduces the chances of errors related to string literals.
The use of nameof in property change notifications is not only a best practice but also a crucial aspect of implementing the INotifyPropertyChanged interface effectively. It allows for cleaner, more reliable data binding in your applications.
Conclusion
The nameof expression in C# is a simple yet powerful feature that can significantly enhance your coding practices. By providing a way to retrieve the names of variables, types, fields, and members as strings, it helps reduce errors and improve code maintainability. Whether you’re using it for logging, validation, or property change notifications, the nameof expression can streamline your development process and make your code cleaner and more efficient. Embracing this feature can lead to better coding habits and a more robust application.
FAQ
-
What is the nameof expression in C#?
The nameof expression is a feature in C# that allows you to obtain the name of a variable, type, field, or member as a string without using quotes. -
When was the nameof expression introduced?
The nameof expression was introduced in C# 6.0. -
How does nameof help with code maintenance?
By using nameof, you avoid hardcoding strings, which can lead to errors during refactoring. If you rename a variable or property, the nameof expression automatically reflects that change. -
Can I use nameof with methods and events?
Yes, the nameof expression can be used with methods and events, making it useful for logging and event handling. -
Why is nameof preferred over string literals?
Using nameof reduces the risk of typos and errors associated with string literals, enhancing code readability and maintainability.
#. Learn how to retrieve variable, type, field, and member names effortlessly. This guide offers practical examples and insights on using nameof for better code maintenance, logging, and property change notifications. Perfect for developers looking to enhance their C# skills.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn