Final Keyword in C#
-
Java’s
final
Keyword Equivalent in C# -
the
final
Keyword for Classes -
the
final
Keyword for Variables (Field Initialization) -
the
final
Keyword for Methods -
Key Points About the
final
Behavior in C# -
When to Use the
final
Behavior - Conclusion
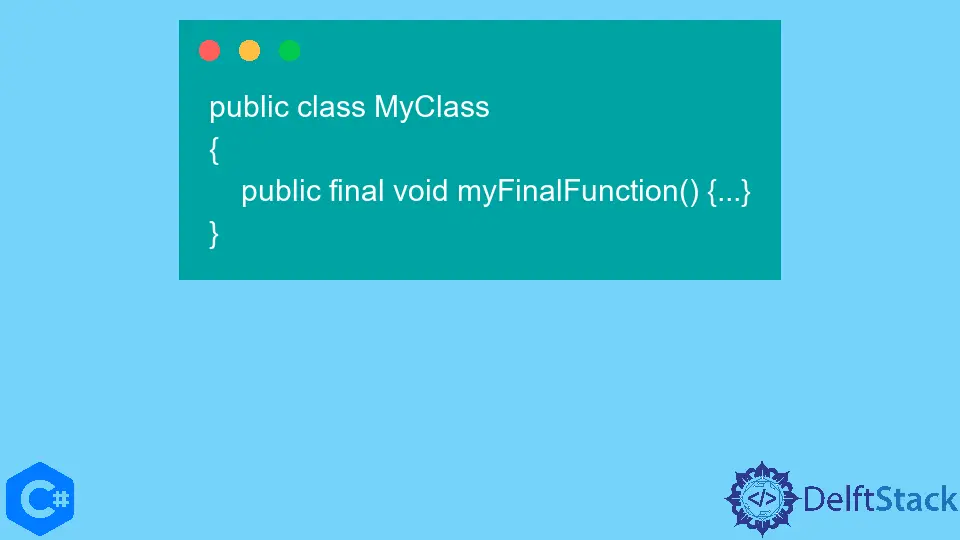
In programming, the final
keyword plays a pivotal role in controlling the behavior of classes, methods, and variables. Unlike languages like Java, C# employs specific modifiers like sealed
for classes and methods and readonly
for variables, achieving similar effects to the final
keyword found in other programming languages.
Understanding the nuances and applications of these modifiers is crucial for ensuring code robustness, immutability, and design integrity. This article will delve into the multifaceted aspects of the final
behavior in C#, elucidating its usage, implications, and significance across different elements of the language.
Java’s final
Keyword Equivalent in C#
Unfortunately, no keyword in C# does the same thing as the final
keyword in Java does. The final
keyword serves different purposes under different contexts.
In class and function declaration, the final
keyword is used to prevent any inheritance from the class or any new definition of the virtual function. In field initialization, the final
keyword is used to specify that the specific field value cannot be modified later on in the program.
In C#, the final
keyword doesn’t exist in the same form as in languages like Java. Instead, C# provides the sealed
modifier to accomplish similar functionality.
When applied to a class, sealed
prevents inheritance, ensuring that other classes cannot extend the class.
Example:
sealed class MyClass {
// Class members and methods
}
the final
Keyword for Classes
The sealed
keyword in C# is equivalent to the final
keyword in the Java language if used for class or function declaration. The following code example shows us how we can use the final
keyword in class declaration in Java.
public final class MyFinalClass {}
The above code prevents us from inheriting the MyFinalClass
class in Java. This goal can be achieved with the sealed
keyword in C#.
The following code example shows us how we can use the sealed
keyword in class declaration in C#.
public sealed class MySealedClass {}
The above code prevents us from inheriting the MySealedClass
class in C#.
The final
keyword also specifies that there can be no more definitions of a virtual function in Java. The following code example shows us how we can use the final
keyword in a function declaration in Java.
public class MyClass {
public final void myFinalFunction() {}
}
This prevents us from making any more definitions of the virtual function myFinalFunction()
in Java.
We can replicate this functionality with the sealed
and override
keywords in C#. The following code example shows us how we can use the sealed
keyword in function declaration in C#.
public class MyChildClass : MyParentClass {
public sealed override void mySealedFunction() {
...
}
}
The above code prevents us from making any more definitions of the virtual function mySealedFunction()
in C#.
the final
Keyword for Variables (Field Initialization)
In C#, the readonly
keyword serves a purpose similar to final
in other languages. It denotes that a field or variable can only be assigned a value once, either at the time of declaration or within the constructor.
The readonly
keyword in C# is equivalent to the final
keyword in the Java language if used for field initialization. The following code example shows us how we can use the final
keyword in field initialization in Java.
public final float g = 10;
This prevents us from changing the value of g
in Java. This goal can be achieved with the readonly
keyword in C#.
The following code example shows us how we can use the readonly
keyword in field initialization in C#.
public readonly float g = 10;
This prevents us from changing the value of g
in C#.
Another example of using the readonly
keyword is below:
class MyClass {
public readonly int myConstant = 10;
public MyClass(int value) {
myConstant = value; // Assigning a value in the constructor
}
}
The readonly
keyword is used to define constants or immutable fields within a class. Once a readonly
variable is assigned a value, it cannot be modified after that, except during its declaration or within constructors.
Therefore, in the provided code, attempting to modify myConstant
outside its declaration or constructor will result in a compilation error due to its readonly
nature.
the final
Keyword for Methods
Similarly, C# employs the sealed
modifier to prevent method overriding within derived classes. By marking a method as sealed
, it cannot be overridden by subclasses, maintaining its implementation intact.
Example:
This code snippet demonstrates the use of the virtual
and override
keywords along with the sealed
modifier in C# to control method inheritance and prevent further overriding.
class BaseClass {
public virtual void MyMethod() {
// Method implementation
}
}
class DerivedClass : BaseClass {
public sealed override void MyMethod() {
// Method implementation in DerivedClass
}
}
Therefore, in this scenario, the DerivedClass
is providing its own implementation of the MyMethod()
inherited from BaseClass
. However, it explicitly seals this overridden method using the sealed
modifier, ensuring that no further derived classes can override this particular implementation of MyMethod()
.
Key Points About the final
Behavior in C#
- Immutability with
sealed
: Thesealed
modifier ensures that a class or method cannot be inherited or overridden, preserving their implementations. readonly
for Constants: Usingreadonly
variables allows for constant values that can’t be changed after initialization, providing a level of immutability to data.- Maintaining Consistency: Applying
sealed
orreadonly
appropriately helps maintain code integrity, preventing unintentional modifications or extensions.
When to Use the final
Behavior
- Security and Design Integrity: Marking classes or methods as
sealed
prevents unauthorized modifications or extensions, enforcing design integrity. - Constant Values: Utilizing
readonly
variables ensures constants remain constant, reducing the risk of accidental changes. - Framework Development: In frameworks or libraries, applying
sealed
ensures specific classes or methods retain their behavior, safeguarding their intended use.
Conclusion
The final
behavior in C# is achieved through the sealed
modifier for classes and methods, as well as the readonly
keyword for variables.
These elements contribute to maintaining code integrity, preventing modifications, and ensuring constants remain constant. Employing these features aids in building robust, secure, and consistent software systems in C#.
By understanding and using the final
behavior appropriately, developers can create more resilient and reliable C# applications, safeguarding critical components from unintended changes or extensions.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn