How to Write to an Excel File in C#
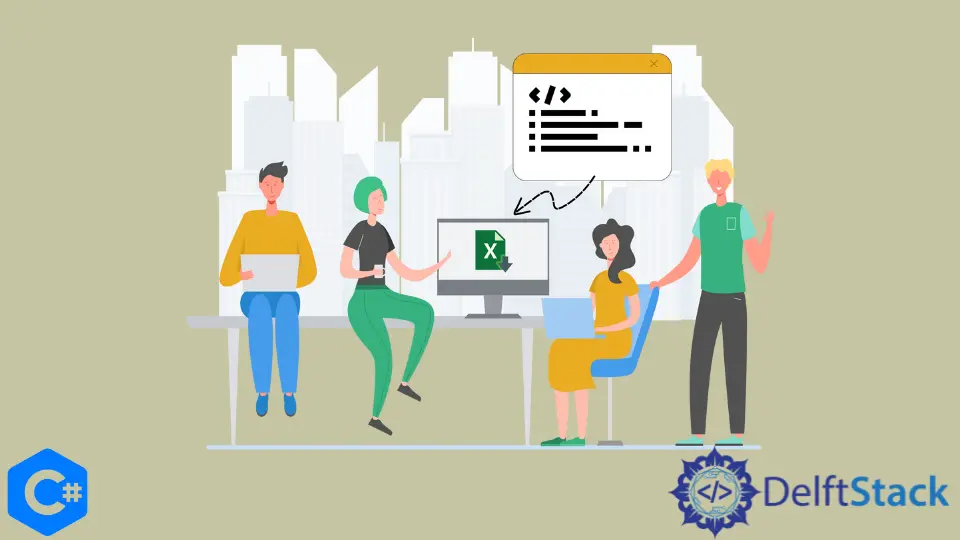
This tutorial will discuss the method to write data to an Excel file in C#.
Write Data to an Excel File With the Microsoft.Office.Interop.Excel
Namespace in C#
The Microsoft.Office.Interop.Excel
namespace provides methods for interacting with the Microsoft Excel application in C#. We can create new Excel sheets, display data of existing sheets, modify the existing Excel sheets’ contents, etc., with this namespace. The following code example shows us how to write our data to an Excel file with the Microsoft.Office.Interop.Excel
namespace in C#. We need to add a reference to the Microsoft.Office.Interop.Excel
namespace from the solution explorer for this approach to work.
using System;
using Excel = Microsoft.Office.Interop.Excel;
namespace write_to_excel {
class Program {
static void writeToExcel() {
Excel.Application myexcelApplication = new Excel.Application();
if (myexcelApplication != null) {
Excel.Workbook myexcelWorkbook = myexcelApplication.Workbooks.Add();
Excel.Worksheet myexcelWorksheet = (Excel.Worksheet)myexcelWorkbook.Sheets.Add();
myexcelWorksheet.Cells[1, 1] = "Value 1";
myexcelWorksheet.Cells[2, 1] = "Value 2";
myexcelWorksheet.Cells[3, 1] = "Value 3";
myexcelApplication.ActiveWorkbook.SaveAs(@"C:\abc.xls",
Excel.XlFileFormat.xlWorkbookNormal);
myexcelWorkbook.Close();
myexcelApplication.Quit();
}
}
static void Main(string[] args) {
writeToExcel();
}
}
}
In the above code, we first initialized an instance of the Excel.Application
class myExcelApplication
. We then initialized the instance myExcelWorkbook
of the Excel.Workbook
class and added a workbook to our myExcelApplication
with the myExcelApplication.Workbooks.Add()
function. After that, we initialized the instance myExcelWorksheet
of the Excel.Worksheet
class and added an excel worksheet to our workbook with the myExcelWorkbook.Sheets.Add()
function.
We then inserted data into the cells inside the myExcelWroksheet
with the myExcelWorksheet.Cells[1, 1] = "Value 1"
. Here, the first index, 1
, is the row index, and the second index, 1
, is the column index. The Excel file was saved by the myExcelApplication.ActiveWorkbook.SaveAs(path, format)
function. In the end, after inserting all the data inside the cells and saving our Excel file, we closed our workbook with myExcelWorkbook.Close()
and exited our application with myExcelApp.Quit()
functions in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn