How to Read XLSX File in C#
-
Read XLSX File With the
LinqToExcel
Package inC#
-
Read XLSX File With the
ExcelDataReader
Package inC#
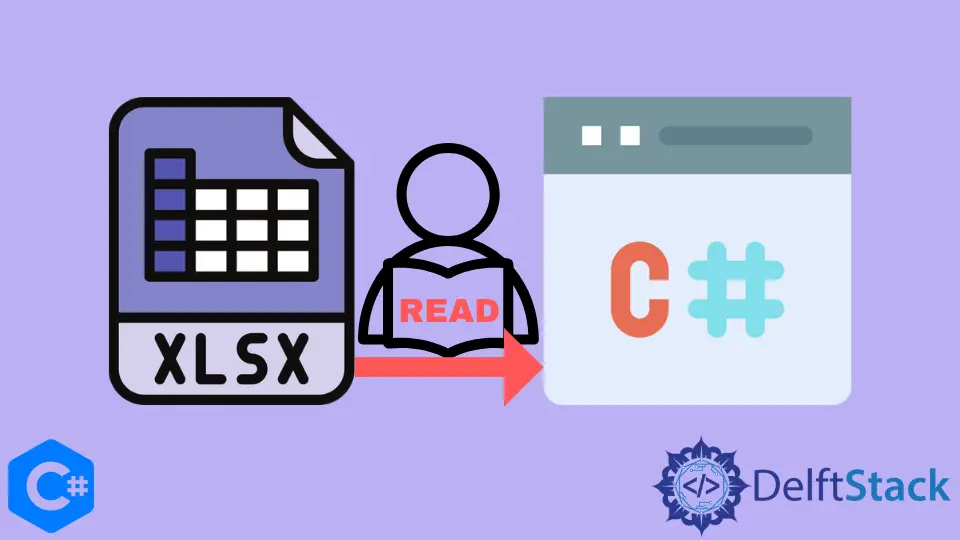
This tutorial will discuss the methods to read an Excel xlsx file in C#.
Read XLSX File With the LinqToExcel
Package in C#
The LinqToExcel
package is used to query Excel files with the LINQ in C#. It makes it easy to get filtered data from an Excel file in C#. The LinqToExcel
package is an external package and needs to be installed first for this approach to work. We can install this package using the NuGet package manager by searching linqtoexcel
in the NuGet package manager. We also need to install the Microsoft Access Database Engine for the LinqToExcel
package. When both the LinqToExcel
package and the Microsoft Access Database Engine are finally installed, we can read the xlsx files. See the following code example.
using ExcelDataReader;
using System.IO;
using System.Linq;
namespace read_excel_file {
class Program {
static void Main(string[] args) {
var excelFile = new LinqToExcel.ExcelQueryFactory(@"C:\File\Classes.xlsx");
var result = from row in excelFile.Worksheet("Sheet1") let item =
new {
RollNumber = row["Roll Number"].Cast<string>(),
Name = row["Name"].Cast<string>(),
Class = row["Class"].Cast<string>(),
}
where item.Class == "5" select item;
}
}
}
In the above code, we used the LINQ to query through the file C:\File\Classes.xlsx
and get filtered content from the Sheet1
with the LinqToExcel
package in C#. We saved the resultant value of the query inside the result
variable.
Read XLSX File With the ExcelDataReader
Package in C#
We can also use the ExcelDataReader
package to read data from Excel files in C#. The ExcelDataReader
package is also an external package and does not come pre-installed with the .NET
framework. We need to install it for this approach to work. We can install this package by simply just searching the exceldatareader
in the NuGet package manager. The following code example shows us how we can read data from an xlsx file with the ExcelDataReader
package in C#.
using System.Data;
using System.IO;
using System.Linq;
namespace read_excel_file {
class Program {
static void Main(string[] args) {
FileStream fStream = File.Open(@"C:\File\Classes.xlsx", FileMode.Open, FileAccess.Read);
IExcelDataReader excelDataReader = ExcelReaderFactory.CreateOpenXmlReader(fStream);
DataSet resultDataSet = excelDataReader.AsDataSet();
excelDataReader.Close();
}
}
}
In the above code, we read the data inside the C:\File\Classes.xlsx
file with the ExcelDataReader
package in C#. We saved the resultant data in the form of tables inside the resultDataSet
variable. In the end, we free up the resources held by the excelDataReader
instance with the excelDataReader.Close()
function in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn