在 C# 中读取 XLSX 文件
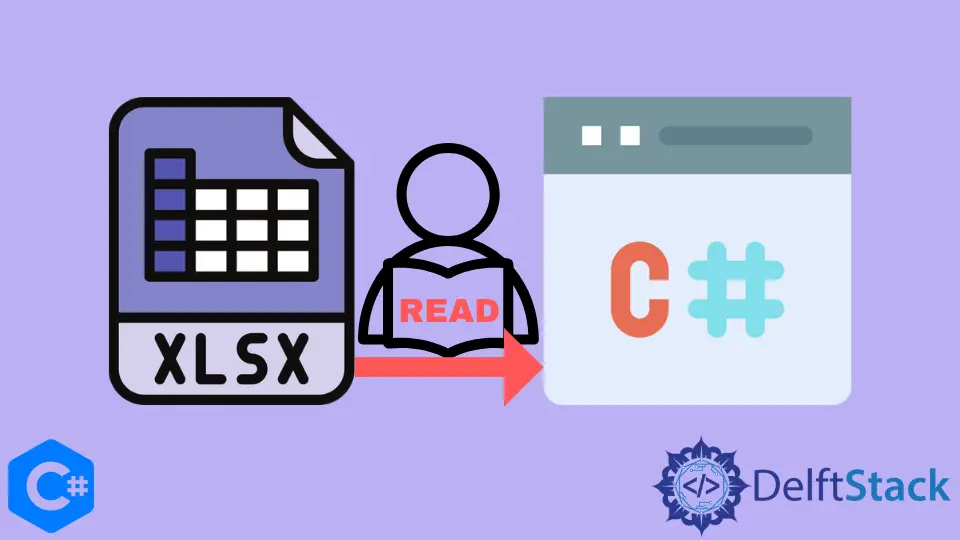
本教程将讨论在 C# 中读取 Excel xlsx 文件的方法。
使用 C# 中的 LinqToExcel
包读取 XLSX 文件
LinqToExcel
程序包用于通过 C# 中的 LINQ 查询 Excel 文件。它使从 C# 中的 Excel 文件中获取经过过滤的数据变得容易。LinqToExcel
软件包是一个外部软件包,需要首先安装此方法才能起作用。我们可以通过在 NuGet 程序包管理器中搜索 linqtoexcel
,使用 NuGet 程序包管理器安装此程序包。我们还需要为 LinqToExcel
软件包安装 Microsoft Access 数据库引擎。最终安装了 LinqToExcel
软件包和 Microsoft Access 数据库引擎时,我们可以读取 xlsx 文件。请参见以下代码示例。
using ExcelDataReader;
using System.IO;
using System.Linq;
namespace read_excel_file {
class Program {
static void Main(string[] args) {
var excelFile = new LinqToExcel.ExcelQueryFactory(@"C:\File\Classes.xlsx");
var result = from row in excelFile.Worksheet("Sheet1") let item =
new {
RollNumber = row["Roll Number"].Cast<string>(),
Name = row["Name"].Cast<string>(),
Class = row["Class"].Cast<string>(),
}
where item.Class == "5" select item;
}
}
}
在上面的代码中,我们使用 LINQ 来查询文件 C:\File\Classes.xlsx
,并使用 C# 中的 LinqToExcel
包从 Sheet1
中获取过滤后的内容。我们将查询的结果值保存在 result
变量中。
使用 C# 中的 ExcelDataReader
包读取 XLSX 文件
我们还可以使用 ExcelDataReader
包从 C# 中的 Excel 文件中读取数据。ExcelDataReader
程序包也是一个外部程序包,并且没有预装有 .NET
框架。我们需要安装它才能使这种方法起作用。我们只需在 NuGet 软件包管理器中搜索 exceldatareader
即可安装此软件包。下面的代码示例向我们展示了如何使用 C# 中的 ExcelDataReader
包从 xlsx 文件读取数据。
using System.Data;
using System.IO;
using System.Linq;
namespace read_excel_file {
class Program {
static void Main(string[] args) {
FileStream fStream = File.Open(@"C:\File\Classes.xlsx", FileMode.Open, FileAccess.Read);
IExcelDataReader excelDataReader = ExcelReaderFactory.CreateOpenXmlReader(fStream);
DataSet resultDataSet = excelDataReader.AsDataSet();
excelDataReader.Close();
}
}
}
在上面的代码中,我们使用 C# 中的 ExcelDataReader
包读取 C:\File\Classes.xlsx
文件中的数据。我们将结果数据以表格的形式保存在 resultDataSet
变量内。最后,我们使用 C# 中的 excelDataReader.Close()
函数释放 excelDataReader
实例所拥有的资源。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn