How to Ping an IP in C#
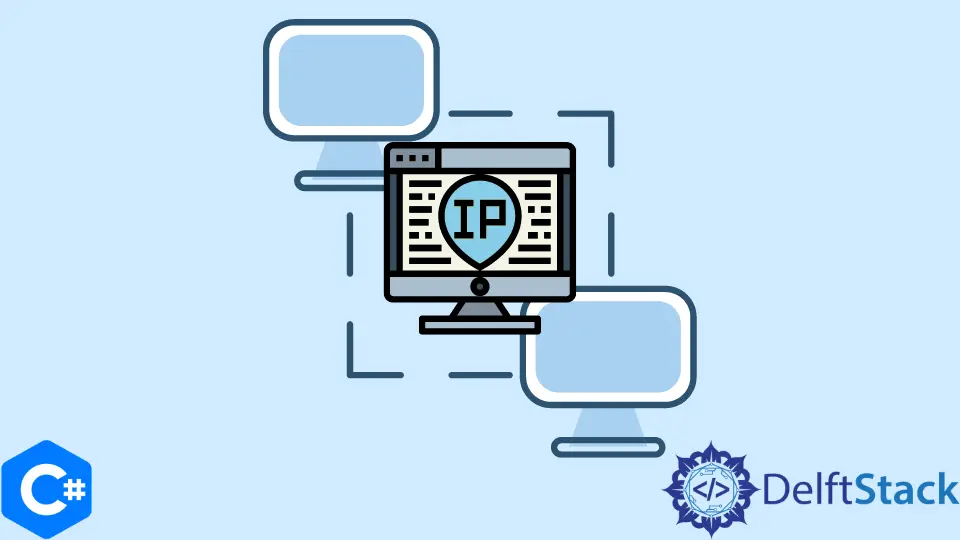
This tutorial will teach you how to ping an IP address using the computer language C#.
Ping an IP in C#
The Send()
function of the Ping class takes the hostname or address as its parameters and a timeout value. If a message is successfully received, the Send()
function will return a PingReply
object.
This object will either contain information about the ICMP (Internet Control Message Protocol) echo message or, if no message is successfully received, it will indicate the cause for the failure. If an ICMP echo message is not received within the amount of time that the timeout parameter has allotted, the Status
property will be changed to TimedOut.
The Status
property will be set to Success
if an attempt to submit an ICMP echo request and get the associated ICMP echo reply is successful.
To begin, we will need to import the libraries and then access the Ping
class inside our application using the System.Net.NetworkInformation
namespace.
using System;
using System.Text;
using System.Net.NetworkInformation;
Make a new class called PingIP,
and then within that class, write the main()
function that will be used to launch the application.
namespace PingProto {
public class PingIP {
static void Main(string[] args) {}
}
}
Now, we need to make a try catch
block to test whether the code is being run properly.
We will implement how to ping an IP in the try
block. If this is not the case, we need to throw an exception in the catch
block.
try {
} catch {
}
Inside the try
block, we need an object of type Ping
to get things going. Afterward, we request a response from the device or server we ping.
To do this, we must use the Send()
function while passing two parameters. The host’s name will come first, followed by the remaining time.
Ping myPing = new Ping();
PingReply reply = myPing.Send("192.168.18.84", 1000);
We apply a condition using an if
statement to ensure the reply is received.
if (reply != null) {
}
After that, we can get the value using the Status, Time,
and Address
fields.
Console.WriteLine("Status : " + reply.Status + " \nTime : " + reply.RoundtripTime.ToString() +
"\nAddress : " + reply.Address);
Inside the catch
block, we will display an error message if the code does not run successfully.
catch {
Console.WriteLine("An error occurred while pinging an IP");
}
Complete Source Code:
using System;
using System.Text;
using System.Net.NetworkInformation;
namespace PingProto {
public class PingIP {
static void Main(string[] args) {
try {
Ping myPing = new Ping();
PingReply reply = myPing.Send("192.168.18.84", 1000);
if (reply != null) {
Console.WriteLine("Status of the Ping: " + reply.Status +
" \nTime of the Ping: " + reply.RoundtripTime.ToString() +
"\nIP Address of the Ping: " + reply.Address);
}
} catch {
Console.WriteLine("An error occurred while pinging an IP");
}
}
}
}
Output:
Status of the Ping: Success
Time of the Ping: 0
IP Address of the Ping: 192.168.18.84
We also have a function known as SendPingAsync()
that may be used in place of the Send()
method. This function accepts a single input referred to as hostNameOrAddress
.
PingReply reply = await myPing.SendPingAsync("192.168.18.84");
To utilize this function, we need to use the async
keyword, as illustrated below.
static async Task Main(string[] args) {}
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn