C# で IP を ping する
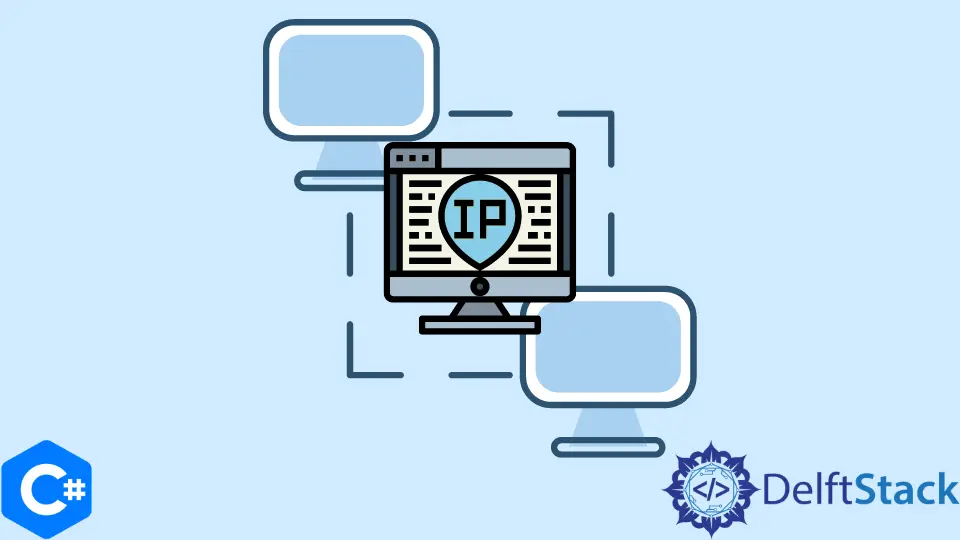
このチュートリアルでは、コンピューター言語 C# を使用して IP アドレスに ping を実行する方法を説明します。
C#
で IP を ping する
Ping クラスの Send()
関数は、ホスト名またはアドレスをパラメーターとタイムアウト値として受け取ります。 メッセージが正常に受信された場合、Send()
関数は PingReply
オブジェクトを返します。
このオブジェクトには、ICMP (Internet Control Message Protocol) エコー メッセージに関する情報が含まれるか、メッセージが正常に受信されない場合は、失敗の原因が示されます。 タイムアウト パラメータで割り当てられた時間内に ICMP エコー メッセージが受信されない場合、Status
プロパティは TimedOut.
に変更されます。
ICMP エコー要求を送信し、関連する ICMP エコー応答を取得する試みが成功した場合、Status
プロパティは Success
に設定されます。
まず、ライブラリをインポートし、System.Net.NetworkInformation
名前空間を使用してアプリケーション内の Ping
クラスにアクセスする必要があります。
using System;
using System.Text;
using System.Net.NetworkInformation;
PingIP,
という名前の新しいクラスを作成し、そのクラス内に、アプリケーションの起動に使用される main()
関数を記述します。
namespace PingProto {
public class PingIP {
static void Main(string[] args) {}
}
}
ここで、コードが適切に実行されているかどうかをテストするために try catch
ブロックを作成する必要があります。
try
ブロックで IP を ping する方法を実装します。 そうでない場合は、catch
ブロックで例外をスローする必要があります。
try {
} catch {
}
try
ブロック内では、物事を進めるために Ping
型のオブジェクトが必要です。 その後、ping を実行したデバイスまたはサーバーからの応答を要求します。
これを行うには、Send()
関数を使用して 2つのパラメーターを渡す必要があります。 ホストの名前が最初に表示され、その後に残り時間が表示されます。
Ping myPing = new Ping();
PingReply reply = myPing.Send("192.168.18.84", 1000);
if
ステートメントを使用して条件を適用し、返信が確実に受信されるようにします。
if (reply != null) {
}
その後、Status, Time,
および Address
フィールドを使用して値を取得できます。
Console.WriteLine("Status : " + reply.Status + " \nTime : " + reply.RoundtripTime.ToString() +
"\nAddress : " + reply.Address);
catch
ブロック内では、コードが正常に実行されない場合にエラー メッセージが表示されます。
catch {
Console.WriteLine("An error occurred while pinging an IP");
}
完全なソース コード:
using System;
using System.Text;
using System.Net.NetworkInformation;
namespace PingProto {
public class PingIP {
static void Main(string[] args) {
try {
Ping myPing = new Ping();
PingReply reply = myPing.Send("192.168.18.84", 1000);
if (reply != null) {
Console.WriteLine("Status of the Ping: " + reply.Status +
" \nTime of the Ping: " + reply.RoundtripTime.ToString() +
"\nIP Address of the Ping: " + reply.Address);
}
} catch {
Console.WriteLine("An error occurred while pinging an IP");
}
}
}
}
出力:
Status of the Ping: Success
Time of the Ping: 0
IP Address of the Ping: 192.168.18.84
Send()
メソッドの代わりに使用できる SendPingAsync()
として知られる関数もあります。 この関数は、hostNameOrAddress
と呼ばれる単一の入力を受け入れます。
PingReply reply = await myPing.SendPingAsync("192.168.18.84");
この関数を利用するには、以下に示すように async
キーワードを使用する必要があります。
static async Task Main(string[] args) {}
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn