C#에서 IP 핑
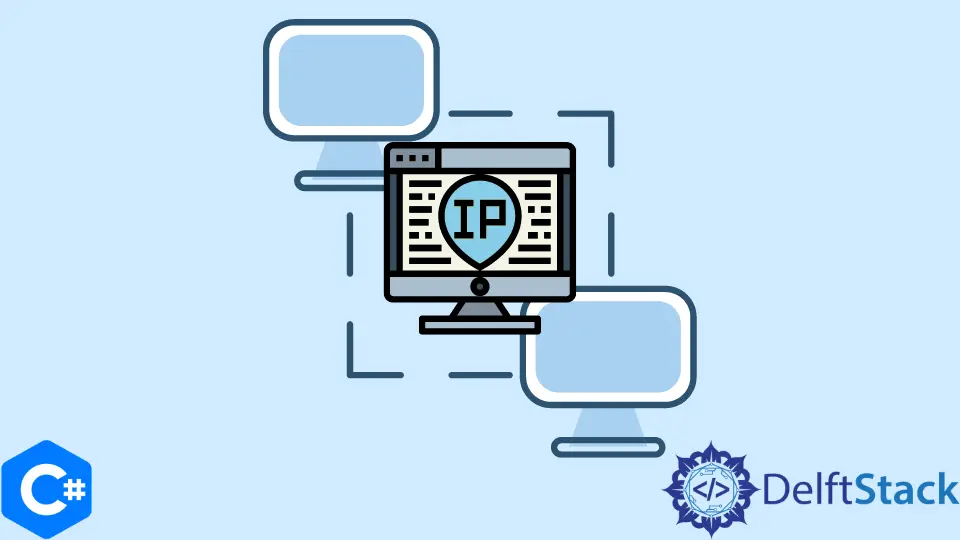
이 자습서에서는 컴퓨터 언어 C#을 사용하여 IP 주소를 ping하는 방법을 알려줍니다.
C#
에서 IP 핑
Ping 클래스의 Send()
기능은 호스트 이름 또는 주소를 매개변수 및 시간 초과 값으로 사용합니다. 메시지가 성공적으로 수신되면 Send()
함수는 PingReply
개체를 반환합니다.
이 객체는 ICMP(Internet Control Message Protocol) 에코 메시지에 대한 정보를 포함하거나 메시지가 성공적으로 수신되지 않은 경우 실패 원인을 나타냅니다. timeout 매개변수가 할당한 시간 내에 ICMP 에코 메시지가 수신되지 않으면 Status
속성이 TimedOut.
으로 변경됩니다.
ICMP 에코 요청을 제출하고 관련 ICMP 에코 응답을 받으려는 시도가 성공하면 Status
속성이 Success
로 설정됩니다.
시작하려면 라이브러리를 가져온 다음 System.Net.NetworkInformation
네임스페이스를 사용하여 애플리케이션 내부의 Ping
클래스에 액세스해야 합니다.
using System;
using System.Text;
using System.Net.NetworkInformation;
PingIP,
라는 새 클래스를 만든 다음 해당 클래스 내에서 응용 프로그램을 시작하는 데 사용할 main()
함수를 작성합니다.
namespace PingProto {
public class PingIP {
static void Main(string[] args) {}
}
}
이제 코드가 제대로 실행되고 있는지 테스트하기 위해 try catch
블록을 만들어야 합니다.
try
블록에서 IP를 ping하는 방법을 구현합니다. 그렇지 않은 경우 catch
블록에서 예외를 발생시켜야 합니다.
try {
} catch {
}
try
블록 안에는 Ping
유형의 객체가 필요합니다. 그런 다음 핑하는 장치 또는 서버의 응답을 요청합니다.
이를 위해서는 두 개의 매개변수를 전달하면서 Send()
함수를 사용해야 합니다. 호스트의 이름이 먼저 나오고 나머지 시간이 그 뒤에 나옵니다.
Ping myPing = new Ping();
PingReply reply = myPing.Send("192.168.18.84", 1000);
응답이 수신되었는지 확인하기 위해 if
문을 사용하여 조건을 적용합니다.
if (reply != null) {
}
그런 다음 상태, 시간
및 주소
필드를 사용하여 값을 가져올 수 있습니다.
Console.WriteLine("Status : " + reply.Status + " \nTime : " + reply.RoundtripTime.ToString() +
"\nAddress : " + reply.Address);
코드가 성공적으로 실행되지 않으면 catch
블록 내에서 오류 메시지를 표시합니다.
catch {
Console.WriteLine("An error occurred while pinging an IP");
}
완전한 소스 코드:
using System;
using System.Text;
using System.Net.NetworkInformation;
namespace PingProto {
public class PingIP {
static void Main(string[] args) {
try {
Ping myPing = new Ping();
PingReply reply = myPing.Send("192.168.18.84", 1000);
if (reply != null) {
Console.WriteLine("Status of the Ping: " + reply.Status +
" \nTime of the Ping: " + reply.RoundtripTime.ToString() +
"\nIP Address of the Ping: " + reply.Address);
}
} catch {
Console.WriteLine("An error occurred while pinging an IP");
}
}
}
}
출력:
Status of the Ping: Success
Time of the Ping: 0
IP Address of the Ping: 192.168.18.84
또한 Send()
메서드 대신 사용할 수 있는 SendPingAsync()
라는 함수가 있습니다. 이 함수는 hostNameOrAddress
라는 단일 입력을 허용합니다.
PingReply reply = await myPing.SendPingAsync("192.168.18.84");
이 기능을 활용하려면 아래 그림과 같이 async
키워드를 사용해야 합니다.
static async Task Main(string[] args) {}
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn