How to Get the Index of the Current Iteration of a Foreach Loop in C#
-
C# Program to Get the
index
of the Current Iteration of aforeach
Loop UsingSelect()
Method -
C# Program to Get the
index
of the Current Iteration of aforeach
Loop Usingindex variable
Method
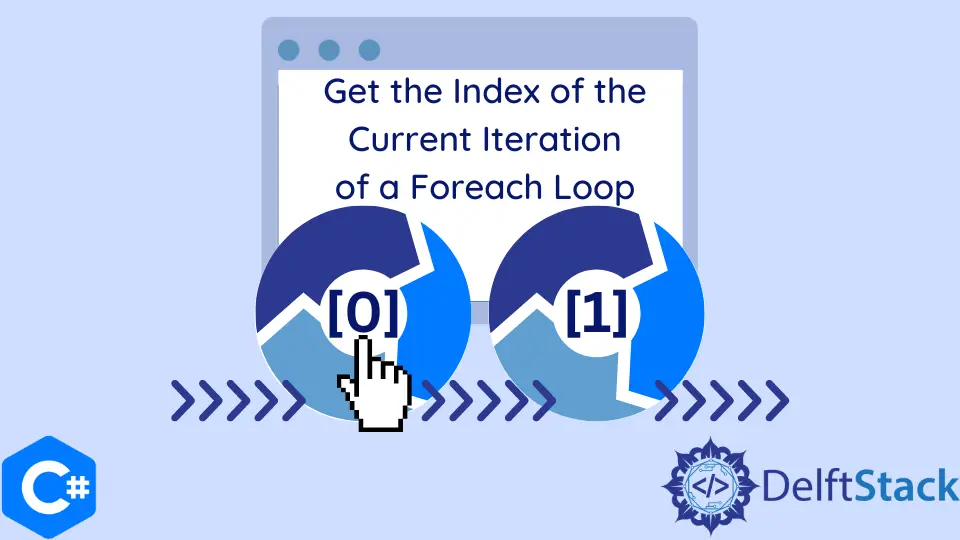
In C#, we have mainly two loops, for
loop and foreach
loop. The foreach
loop is considered to be the best because it is suitable for all types of operations. Even for the ones in which we do not need the index
value.
There are some cases in which we need to use the foreach
loop but we also have to get the index
number. To solve this problem, in C#, we have different methods to get the index
of the current iteration of a foreach
loop, for example, Select()
and index variable method.
C# Program to Get the index
of the Current Iteration of a foreach
Loop Using Select()
Method
The method Select()
is a LINQ
method. LINQ
is a part of C# that is used to access different databases and data sources. The Select()
method selects the value and index
of the iteration of a foreach
loop.
The correct syntax to use this method is as follows:
Select((Value, Index) => new { Value, Index });
Example Code:
using System;
using System.Linq;
using System.Collections.Generic;
public class IndexOfIteration {
public static void Main() {
// Creating integer List
List<int> Numbers = new List<int>() { 1, 2, 3, 4, 8, 10 };
// Visiting each value of List using foreach loop
foreach (var New in Numbers.Select((value, index) => new { value, index })) {
Console.WriteLine("The Index of Iteration is: {0}", New.index);
}
}
}
Output:
The Index of Iteration is: 0
The Index of Iteration is: 1
The Index of Iteration is: 2
The Index of Iteration is: 3
The Index of Iteration is: 4
The Index of Iteration is: 5
C# Program to Get the index
of the Current Iteration of a foreach
Loop Using index variable
Method
This is the traditional and the most simple method to find the index
of iteration of a foreach
loop. In this method, we use a variable and initialize it with zero and then do increment in its value in each iteration.
This is the most basic method. It only requires the basic knowledge of C# to implement this method.
Example Code:
using System;
using System.Collections.Generic;
public class IndexOfIteration {
public static void Main() {
// Creating an integer List
List<int> Numbers = new List<int>() { 1, 2, 3, 4, 8, 10 };
int index = 0;
// Visiting each value of List using foreach loop
foreach (var Number in Numbers) {
Console.WriteLine("The Index of Iteration is {0}", index);
index++;
}
}
}
Output:
The Index of Iteration is: 0
The Index of Iteration is: 1
The Index of Iteration is: 2
The Index of Iteration is: 3
The Index of Iteration is: 4
The Index of Iteration is: 5