How to Exit a Foreach Loop in C#
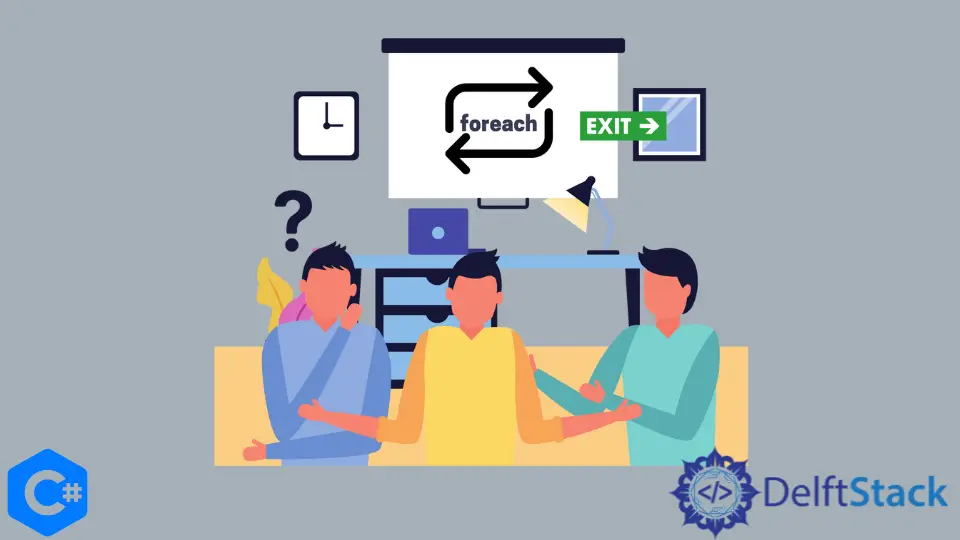
In this guide, we will learn how to exit a foreach loop in C#. The foreach loop is a powerful construct in C# that allows you to iterate over collections like arrays, lists, and dictionaries. However, there are times when you might want to break out of the loop early, either when a condition is met or when you no longer need to process the remaining items. Understanding how to effectively exit a foreach loop can enhance your control over your code’s flow, making it more efficient and easier to read. Let’s dive into the methods available for exiting a foreach loop in C#.
Using the break Statement
The most straightforward way to exit a foreach loop in C# is by using the break
statement. This command immediately terminates the loop and transfers control to the statement following the loop. Here’s how it works:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6 };
foreach (int number in numbers)
{
if (number == 4)
{
break;
}
Console.WriteLine(number);
}
}
}
Output:
1
2
3
In this example, the foreach loop iterates over a list of integers. When the loop encounters the number 4, the break
statement is executed, which exits the loop immediately. As a result, only the numbers 1, 2, and 3 are printed to the console. This method is particularly useful when you are searching for a specific item or condition and want to stop processing once it is found.
Using the return Statement
Another way to exit a foreach loop, especially when you are inside a method, is by using the return
statement. This not only exits the loop but also returns control to the calling method, effectively terminating the entire method execution. Here’s an example:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6 };
PrintNumbers(numbers);
}
static void PrintNumbers(List<int> numbers)
{
foreach (int number in numbers)
{
if (number == 4)
{
return;
}
Console.WriteLine(number);
}
}
}
Output:
1
2
3
In this scenario, the PrintNumbers
method iterates over a list of integers. When it reaches the number 4, the return
statement is executed, which not only exits the loop but also the method itself. As a result, only numbers 1, 2, and 3 are printed. This method is particularly useful when you want to stop processing not just the loop but also the entire method under certain conditions.
Using a Flag Variable
Sometimes, you may want to control the exit of a foreach loop using a flag variable. This method allows for more complex logic and conditions for exiting the loop. Here’s an example of how to implement this:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6 };
bool exitLoop = false;
foreach (int number in numbers)
{
if (exitLoop)
{
break;
}
if (number == 4)
{
exitLoop = true;
}
Console.WriteLine(number);
}
}
}
Output:
1
2
3
In this example, a boolean flag variable named exitLoop
is used to control the exit condition of the loop. Initially set to false
, it changes to true
when the number 4 is encountered. The loop checks this flag at the beginning of each iteration, and if it is true
, the break
statement is executed, exiting the loop. This method is useful when you have multiple conditions that could lead to exiting the loop, allowing for more flexible control.
Conclusion
Exiting a foreach loop in C# can be achieved through various methods, each with its own use cases and benefits. Whether you choose to use the break
statement for immediate exits, the return
statement for method termination, or a flag variable for more complex logic, understanding these techniques can significantly improve the flow and efficiency of your code. Mastering these concepts will not only enhance your coding skills but also make your programs more robust and easier to maintain. Happy coding!
FAQ
-
Can I use a foreach loop with any collection type?
Yes, foreach loops can be used with any collection that implements the IEnumerable interface, including arrays, lists, and dictionaries. -
Is it possible to skip iterations in a foreach loop?
Yes, you can use thecontinue
statement to skip the current iteration and move on to the next one. -
What happens if I don’t exit the foreach loop?
If you don’t exit the loop, it will continue to iterate through the entire collection until it has processed all items. -
Can I use multiple break statements in a foreach loop?
Yes, you can use multiple break statements within different conditional blocks in the loop, but only one will be executed during a single iteration. -
Is there a performance impact when using break or return statements?
No, using break or return statements does not significantly impact performance, but they can improve the efficiency of your code by avoiding unnecessary iterations.
#. Explore methods like using break and return statements, as well as flag variables for controlling loop execution. Enhance your C# coding skills with practical examples and clear explanations.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn