C# で Foreach ループを終了する
Haider Ali
2023年10月12日
Csharp
Csharp Loop
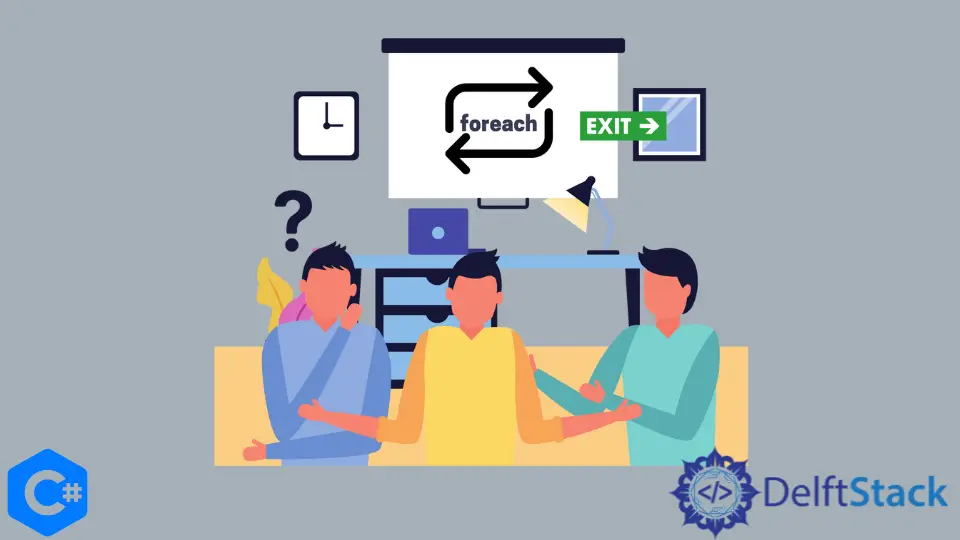
このガイドでは、C# で foreach
ループを終了する方法を説明します。これはさらに別の簡単な方法であり、複雑さはありません。
このガイドに飛び込んで、このコードの実装を見てみましょう。
C#
で foreach
ループを終了する
foreach
ループまたはその他のループを終了するために使用できる方法は 2つあります。foreach
ループを終了することは、他のループを終了することと同じです。
これらの方法は両方とも非常に一般的であり、他の多くの言語でも主に使用されている方法です。たとえば、C、C++、Java など。
break
メソッドまたは return
メソッドのいずれかを使用できます。これらの両方の方法を使用して、foreach
ループを終了できます。
次のコードを見てください。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace For_Each_Loop {
class Program {
static void Main(string[] args) {
int[] numbers_arr = { 4, 5, 6, 1, 2, 3, -2, -1, 0 }; // Example Array
foreach (int i in numbers_arr) {
System.Console.Write("{0} ", i);
Console.ReadKey(); // To Stay On Screen
if (i == 3) {
break; // IF You want To break and end the function call
}
}
foreach (int i in numbers_arr) {
System.Console.Write("{0} ", i);
Console.ReadKey(); // To Stay On Screen
if (i == 2) {
return; // IF You want To break and end the function call
}
}
}
}
}
上記のコードでは、break
と return
の両方を使用しています。あなたがする必要があるのは break;
を書くことだけですまたは return;
必要に応じて、foreach
ループ内。
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Haider Ali
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn