C# で foreach ループの現在の反復のインデックスを取得する
Minahil Noor
2023年10月12日
Csharp
Csharp Loop
-
Select()
メソッドを使用して、foreach
ループの現在の反復のindex
を取得する C# プログラム -
インデックス変数
メソッドを使用してforeach
ループの現在の反復のインデックス
を取得する C# プログラム
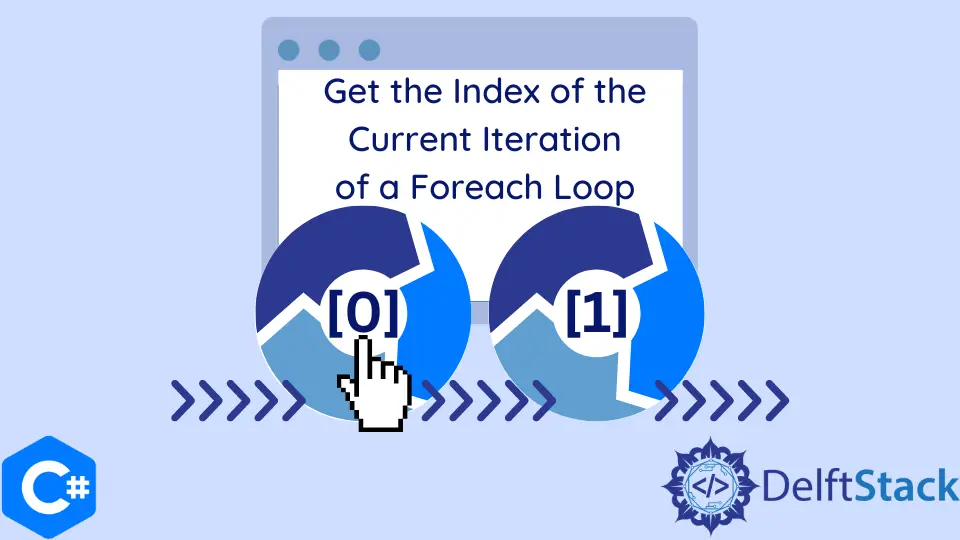
C# では、主に 2つのループ、for
ループと foreach
ループがあります。foreach
ループはすべてのタイプの操作に適しているため、最良のループと見なされます。index
値を必要としないものでもです。
foreach
ループを使用する必要がある場合もありますが、index
番号も取得する必要があります。この問題を解決するために、C# では、foreach
ループの現在の反復の index
を取得するためのさまざまなメソッド(たとえば、Select()
および index
変数メソッド)を使用します。
Select()
メソッドを使用して、foreach
ループの現在の反復の index
を取得する C# プログラム
メソッド Select()
は LINQ
メソッドです。LINQ
は、さまざまなデータベースやデータソースへのアクセスに使用される C# の一部です。Select()
メソッドは、foreach
ループの反復の値と index
を選択します。
このメソッドを使用するための正しい構文は次のとおりです。
Select((Value, Index) => new { Value, Index });
コード例:
using System;
using System.Linq;
using System.Collections.Generic;
public class IndexOfIteration {
public static void Main() {
// Creating integer List
List<int> Numbers = new List<int>() { 1, 2, 3, 4, 8, 10 };
// Visiting each value of List using foreach loop
foreach (var New in Numbers.Select((value, index) => new { value, index })) {
Console.WriteLine("The Index of Iteration is: {0}", New.index);
}
}
}
出力:
The Index of Iteration is: 0
The Index of Iteration is: 1
The Index of Iteration is: 2
The Index of Iteration is: 3
The Index of Iteration is: 4
The Index of Iteration is: 5
インデックス変数
メソッドを使用して foreach
ループの現在の反復のインデックス
を取得する C# プログラム
これは、foreach
ループの反復の index
を見つけるための最も単純で伝統的な方法です。このメソッドでは、変数を使用してゼロで初期化し、各反復でその値をインクリメントします。
これが最も基本的な方法です。このメソッドを実装するには、C# の基本的な知識のみが必要です。
コード例:
using System;
using System.Collections.Generic;
public class IndexOfIteration {
public static void Main() {
// Creating an integer List
List<int> Numbers = new List<int>() { 1, 2, 3, 4, 8, 10 };
int index = 0;
// Visiting each value of List using foreach loop
foreach (var Number in Numbers) {
Console.WriteLine("The Index of Iteration is {0}", index);
index++;
}
}
}
出力:
The Index of Iteration is: 0
The Index of Iteration is: 1
The Index of Iteration is: 2
The Index of Iteration is: 3
The Index of Iteration is: 4
The Index of Iteration is: 5
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe