How to Define the Type Alias in C#
-
Use the
using alias
Directive to Define the Type Alias inC#
-
Use the
namespace
Keyword to Define the Type Alias inC#
-
Use Inheritance to Define the Type Alias in
C#
-
Use the
NuGet
Packages to Define the Type Alias inC#
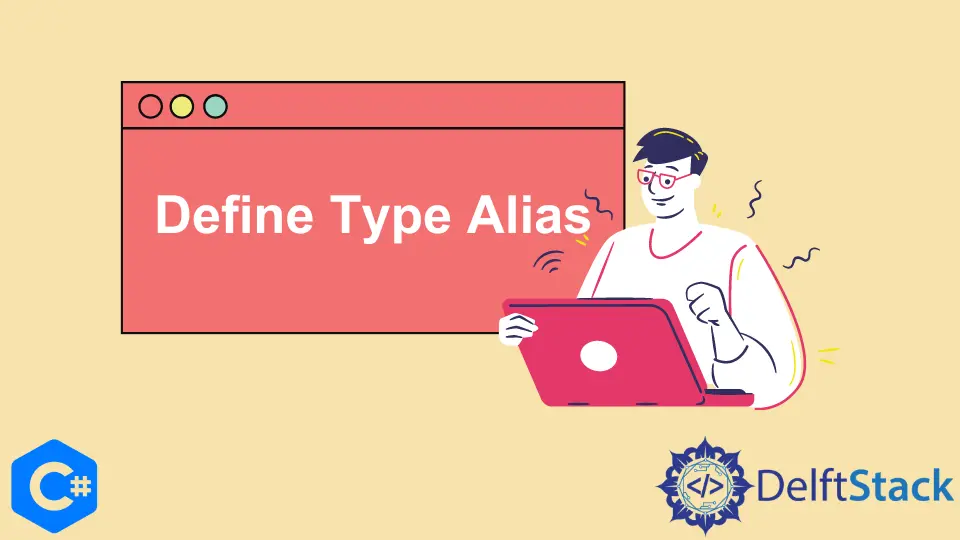
A type alias is something like typedefs
essential for type-safe programming and to create a new type semantically identical to an existing type. C# doesn’t offer a built-in type alias; however, you can use C# directives, classes, and inheritance to compose custom types.
In this tutorial, you will learn the four primary ways to create and define type alias in C#.
Each method has limitations, like using inheritance for creating type alias will not work for primitive types. As a result, you cannot derive from the sealed classes, and most .NET
classes are sealed.
Use the using alias
Directive to Define the Type Alias in C#
The using
keyword is at the heart of type-alias-related features in C# or .NET. It clarifies to programmers how to utilize aliasing properties, make your code flexible, and enhance its readability.
Its ability to define the scope of objects at its disposal makes it extremely efficient; it is an important part of creating an alias for a namespace. It can lead programmers to import members of a single class with the static directive or import specific types from other namespaces.
Most importantly, type alias resolves ambiguity by avoiding importing a whole namespace and makes it easy to work with multiple types. Furthermore, the using alias
allows the creation of global aliases that work across C# projects; passing a parameter type enables you to alias generic types, e.g., using type_alias = System.IO.StreamWriter
and for the generic alias of a type:
using type_generic = System.Nullable<System.Int32>
Example Code:
using System;
using System.Windows.Forms;
using stream_writer = System.IO.StreamWriter; // custom type
namespace type_alias {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
string[] type_data = { "Name: Widow's Sorrow", "Assignment: APK-1229-C", "Success Ratio: 78%",
"Charisma: 11%", "Style: Professional" };
using (stream_writer type_alias = new stream_writer(@"C:\Temp\MyFile.txt")) {
foreach (string line in type_data) {
type_alias.WriteLine(line);
// for compile purposes; MessageBox.Show(line);
}
MessageBox.Show("Process completed!");
}
}
}
}
Output:
Name: Widow's Sorrow
Assignment: APK-1229-C
Success Ratio: 78%
Charisma: 11%
Style: Professional
Using typedef
is another approach to creating a type alias, but it makes the process extremely complex. To simplify it, you must use the Curiously Recurring Template Pattern.
Ignoring the complex and situational-based methods including, lambda syntax
and typedef
, you can define a delegate type by using GenericHandler EventHandler<EventData>
or gcInt.MyEvent +=
in Visual Studio because it provides a complete IntelliSense
event handling signature.
// you can define a type alias for specific types as follows:
using stream_writer = System.IO.StreamWriter // create stream writer object
using file_stream = System.IO.FileStream // create file stream object
// you can define generic alias for a type as follows:
using alias_int = System.Nullable<System.Int32>;
using alias_double = System.Nullable<System.Double>;
C# provides ways to introduce shorter names for things like using Console = System.Console;
. These shortcuts do not have their own identities and can be called aliases, allowing an existing type to go by a different name.
The alias does not introduce new types, which is why you see symbols decorated with their original names in the debugger, which can be confusing but good and efficient.
The using
directive is a perfect equivalent of typedef
; however, it’s important to create an alias for a generic type to close it after a successful operation because it is incredibly harmful to leave the type open. It’s important to resolve type parameters within the using
directive before attempting something like using NullableThing<T> = System.Nullable<T>;
.
Use the namespace
Keyword to Define the Type Alias in C#
The namespaces use classes, making them a perfect way to define type alias. Type or namespace alias is called using alias
directive, like using Project = style.custom.myproject;
.
Namespaces can be user-defined or system-defined, and a using
directive does not give you access to any namespace nested in the namespace you specify. The namespaces make it easy to use the private members of a class by defining type alias in public like using example = Innerclass;
and declaring the outline like Outerclass::example inner;
.
Example Code:
using System;
using System.Windows.Forms;
using class_alias = type_newClass.custom_class;
using typealias_using = namespace_alias.custom_class<int>;
namespace type_newClass {
public class custom_class {
public override string ToString() {
return "You are in type_newClass.custom_class.";
}
}
}
namespace namespace_alias {
class custom_class<T> {
public override string ToString() {
return "You are in namespace_alias.custom_class.";
}
}
}
namespace type_alias {
using type_newClass; // use directive for namespace `type_newClass`
using namespace_alias; // use the directive for namespace `namespace_alias`
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
class_alias inst_typealias_one = new class_alias();
MessageBox.Show(inst_typealias_one.ToString());
typealias_using inst_typealias_two = new typealias_using();
MessageBox.Show(inst_typealias_two.ToString());
}
}
}
Output:
You are in type_newClass.custom_class.
Use Inheritance to Define the Type Alias in C#
Using inheritance is a similar concept, e.g., class ComplexList : List<Tuple<int, string, int>> {}
and the namespace
aliasing is a way to go for a clash-free C# code. Inheritance is an option for making a new type, an instance of another type.
Inheritance benefits you use the features of subclasses, but in any other case, this adds overhead, indirection, slowness, and unreliability. Inheritance is not aliasing but can give similar results in some conditional cases.
The name aliasing approach can force you to redefine it everywhere, resulting in less readable code. Inheritance is a better option in case you add some functionality to the base class, something like:
public class SoldierList : System.Collections.Generic.List<Soldier> {...}
Additionally, inheritance from IEnumerable
makes the class usable in enumerable operations and allows you to define all class behavior completely.
Example Code:
using System;
using System.Windows.Forms;
using System.Collections.Generic;
namespace type_alias {
// a simple approach
class ComplexList : List<Tuple<int, string, int>> {}
// more complex approach - enhances its usability
public class Person {
public string Name { get; set; }
public int Age { get; set; }
public int FavoriteNumber { get; set; }
public Person() {}
public Person(string name, int age, int favoriteNumber) {
this.Name = name;
this.Age = age;
this.FavoriteNumber = favoriteNumber;
}
}
public class PersonList : List<Person> {} // most-refined
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
var complexList = new ComplexList(); // for first approach
var personList = new PersonList(); // for second approach
/*
type your code or program something based on the
new types you created
*/
MessageBox.Show(
"Some function is performed successfully by creating a type alias using inheritance!",
"Type Alias", MessageBoxButtons.OKCancel, MessageBoxIcon.Warning);
}
}
}
Output:
Some function is performed successfully by creating a type alias using inheritance!
Use the NuGet
Packages to Define the Type Alias in C#
A type alias is not limited to a single source code file or namespace block, as you can commonly declare them in header files as globals and class members. To avoid type verbosity, you can declare terse names in functions, especially when using generic code such as HashMap<TKey, TValue>
.
These aliases can be created once and shared across the whole project, and their templates go even further, allowing you to create aliases that don’t resolve to a concrete type.
Using NuGet
packages to type alias can prevent a lot of code duplication and give names to in-between steps such as map
that lives between the very generic HashMap<TKey, TValue>
and the very concrete LocationMap
.
The LikeType
gives you the GenericClass<int>
behavior as an open-source library and a NuGet
package that you can utilize for type alias in C#. Most importantly, don’t forget to add the Kleinware.LikeType 3.00
NuGet package to your C# project in Visual Studio because the using Kleinware.LikeType;
belongs to this package and offers a kind of typedef
functionality to your C# project.
Example Code:
// install `Kleinware.LikeType 3.00` NuGet Package before the program's execution
using System;
using System.Windows.Forms;
using System.Collections.Generic;
using Kleinware.LikeType; // belongs to the `Kleinware.LikeType 3.00`
namespace type_alias {
// type_alias creation
public class SomeInt : LikeType<int> // inherits the integer properties
{
public SomeInt(int value) : base(value) {} // represents the base value
}
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
/*
some example C# code that benefits from `Kleinware.LikeType 3.00`
*/
MessageBox.Show("Successful! Requires self-obervation.", "Type Alias",
MessageBoxButtons.OKCancel, MessageBoxIcon.Information);
}
}
}
Output:
Successful! Requires self-observation.
This tutorial taught you different optimized ways of aliasing in C#. You can use these approaches to build more robust yet clean C# applications.
You can learn a lot from the four technical examples demonstrating the core use cases for type alias and how they might benefit your applications.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub