How to Create New Instance From Type in C#
-
C# Create New Instance From
Type
Using thenew
Operator -
Use the
Activator.CreateInstance
Method to Create a New Instance FromType
in C# -
Use the
Type.GetConstructor
Method to Create a New Instance FromType
in C# -
Use the
FormatterServices.GetUninitializedObject
Method to Create a New Instance FromType
in C# - Conclusion
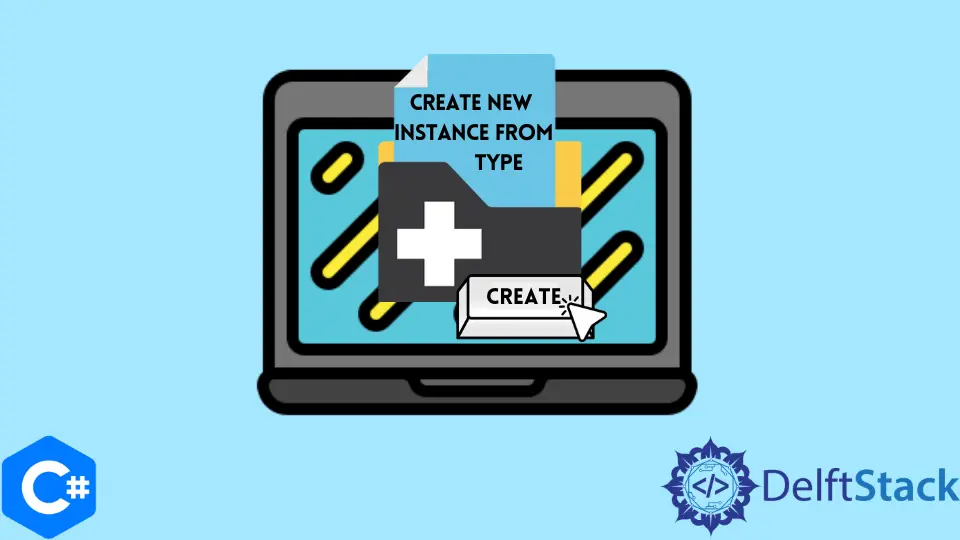
In C#, developers have access to multiple methods for instantiating objects, each tailored to specific use cases. This tutorial explores different techniques for creating new instances from types in C#, providing detailed explanations and example codes for each method.
As we delve into the intricacies of these methods, you’ll discover how to instantiate objects using the conventional new
operator, dynamically create instances at runtime with Activator.CreateInstance
, gain fine-grained control over construction parameters with Type.GetConstructor
, and even create uninitialized instances with FormatterServices.GetUninitializedObject
.
Whether you’re dealing with known types at compile time or dynamically determining types at runtime, understanding these methods will empower you to write more flexible and dynamic C# code. Let’s embark on a journey through the diverse approaches to creating instances from types in C#.
C# Create New Instance From Type
Using the new
Operator
The most straightforward way to create a new instance is by using the new
operator. This method is suitable when the type is known at compile time.
The new
operator in C# is primarily used to create new instances of a class or structure. Its syntax is simple:
ClassName instanceName = new ClassName();
Here, ClassName
represents the type you want to instantiate, and instanceName
is the variable that will reference the newly created object.
Consider a simple class Person
:
public class Person {
public string Name { get; set; }
public Person(string name) {
Name = name;
}
}
Using the new
operator to create an instance of the Person
class:
Person johnDoe = new Person("John Doe");
In this example, a new instance of the Person
class is created, and the constructor is invoked with the parameter "John Doe"
to set the Name
property.
Complete Code:
public class Person {
public string Name { get; set; }
public Person(string name) {
Name = name;
}
}
class Program {
static void Main() {
// Creating a new instance using the new operator
Person johnDoe = new Person("John Doe");
}
}
Instantiating Objects With the new
Operator and Default Constructors
The new
operator is commonly used with classes that have a default (parameterless) constructor. In such cases, you can create an instance without specifying any constructor parameters explicitly.
Consider a class Car
with a default constructor:
public class Car {
public string Model { get; set; }
// Default constructor
public Car() {
Model = "Unknown";
}
}
Creating an instance of the Car
class using the new
operator:
Car myCar = new Car();
Here, the default constructor is called, and the Model
property is initialized to "Unknown"
.
Using the new
Operator With Structs
The new
operator is also applicable to create instances of structures (structs) in C#. Structs are value types, and their instances can be instantiated using the new
operator without the need for the new
keyword.
Consider a simple struct Point
:
public struct Point {
public int X { get; set; }
public int Y { get; set; }
public Point(int x, int y) {
X = x;
Y = y;
}
}
Creating an instance of the Point
struct using the new
operator:
Point origin = new Point(0, 0);
In this example, the new
operator is used to create a new instance of the Point
struct, and the constructor is invoked to set the X
and Y
properties.
Use the Activator.CreateInstance
Method to Create a New Instance From Type
in C#
If we want to create a new instance of a data type at runtime and do not know the data type, we can use the Activator
class and the Type
class to achieve this goal. The Activator
class provides methods for creating instances of objects from types in C#.
The Activator.CreateInstance()
method is used to create an instance of a specified type with the constructor that best suits the specified type in C#. The Type
class represents a data type in C#.
The Activator.CreateInstance
method allows dynamic instantiation of a type. This is useful when the type is determined at runtime.
We can use the Type
class to determine the unknown data type in this scenario. The following code example shows us how we can create a new instance of a data type at runtime without knowing the data type using the Activator
class and the Type
class in C#.
using System;
namespace new_object_from_type {
class Program {
static void Main(string[] args) {
int i = 123;
Type t = i.GetType();
Object n = Activator.CreateInstance(t);
n = 15;
Console.WriteLine(n);
}
}
}
Output:
15
In the above code, we created an instance of the type int32
at the runtime without specifying the data type with the Activator.CreateInstance()
method in C#.
At first, we determined the type of the variable i
with the i.GetType()
method in C#. Then, we created an instance of that type with the Activator.CreateInstance()
method.
The Activator.CreateInstance()
method automatically finds the best constructor for the data type and creates an instance with it. We then initialized the new instance n
with the value 15
and printed it.
Use the Type.GetConstructor
Method to Create a New Instance From Type
in C#
The Type.GetConstructor
method is part of the System.Reflection
namespace in C#. It is used to retrieve information about a type’s constructors, allowing you to obtain a specific constructor based on its parameter types.
Once you have a reference to a constructor, you can use it to dynamically create new instances of the associated type. Using Type.GetConstructor
provides more control over the instantiation process, allowing you to specify constructor parameters.
The syntax for the Type.GetConstructor
method is as follows:
ConstructorInfo GetConstructor(Type[] types);
Here, the types
is an array of Type
objects representing the parameter types of the desired constructor.
Consider a class Person
with a parameterized constructor:
public class Person {
public string Name { get; set; }
public Person(string name) {
Name = name;
}
}
Using Type.GetConstructor
to obtain the constructor information for the Person
class:
Type personType = typeof(Person);
Type[] constructorParameterTypes = { typeof(string) };
ConstructorInfo constructor = personType.GetConstructor(constructorParameterTypes);
In this example, we obtain the Type
object for the Person
class and specify an array of Type
objects representing the parameter types of the constructor we want to retrieve (typeof(string)
in this case).
Once you have obtained the ConstructorInfo
object, you can use it to create new instances of the associated type. The ConstructorInfo.Invoke
method is used for this purpose.
Continuing from the previous example, let’s create a new instance of the Person
class using the obtained constructor:
object[] constructorArguments = { "John Doe" };
object personInstance = constructor.Invoke(constructorArguments);
// Cast the instance to the appropriate type
Person johnDoe = (Person)personInstance;
Here, we provide an array of arguments ("John Doe"
) to the Invoke
method, and it returns an object representing the newly created instance. We then cast this object to the appropriate type (Person
in this case).
Complete Code:
using System;
public class Person {
public string Name { get; set; }
public Person(string name) {
Name = name;
}
}
class Program {
static void Main() {
// Specifying the type dynamically
Type type = typeof(Person);
// Getting the constructor with a parameter
var constructor = type.GetConstructor(new[] { typeof(string) });
// Creating a new instance with constructor parameters
object instance = constructor.Invoke(new object[] { "John Doe" });
// Casting the instance to the appropriate type
Person personInstance = (Person)instance;
}
}
Use the FormatterServices.GetUninitializedObject
Method to Create a New Instance From Type
in C#
The FormatterServices.GetUninitializedObject
method is part of the System.Runtime.Serialization
namespace in C#. It allows developers to create instances of a given type without executing the constructor logic.
This can be particularly useful in scenarios where the initialization logic should be bypassed or when constructing objects with private or internal constructors.
The syntax for the FormatterServices.GetUninitializedObject
method is as follows:
public static object GetUninitializedObject(Type type);
type
: TheType
object represents the type for which an uninitialized instance is required.
The FormatterServices.GetUninitializedObject
method creates an uninitialized instance of a type without invoking its constructor. This is beneficial in scenarios where you need an object without executing its constructor logic.
In the code below, there’s a class MyClass
with a private constructor. Then, we use FormatterServices.GetUninitializedObject
to create an uninitialized instance of the MyClass
.
using System.Runtime.Serialization;
using System;
public class MyClass {
public MyClass() {
// Constructor logic
}
}
class Program {
static void Main() {
// Specifying the type dynamically
Type type = typeof(MyClass);
// Creating an uninitialized instance using GetUninitializedObject
object instance = FormatterServices.GetUninitializedObject(type);
// Casting the instance to the appropriate type
MyClass myInstance = (MyClass)instance;
}
}
In this example, we obtain the Type
object for the MyClass
and use FormatterServices.GetUninitializedObject
to create an uninitialized instance. The resulting object is then cast to the appropriate type (MyClass
).
Conclusion
In C#, creating instances from types can be accomplished through various methods, each with its advantages.
Whether you need to instantiate an object with or without constructor parameters or even without invoking the constructor, the methods outlined above provide the flexibility to meet your specific requirements.
Understanding these techniques enhances your ability to write flexible and dynamic C# code.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn