C#에서 유형 별칭 정의
-
using alias
지시문을 사용하여C#
에서 유형 별칭 정의 -
namespace
키워드를 사용하여C#
에서 유형 별칭 정의 -
상속을 사용하여
C#
에서 유형 별칭 정의 -
NuGet
패키지를 사용하여C#
에서 유형 별칭 정의
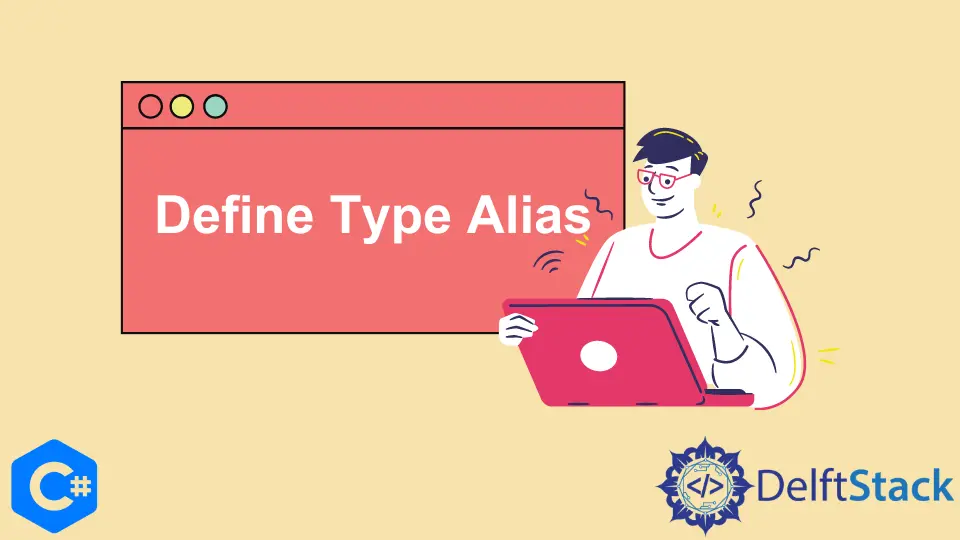
유형 별칭은 유형 안전 프로그래밍과 기존 유형과 의미상 동일한 새 유형을 작성하는 데 필수적인 typedefs
와 같은 것입니다. C#은 기본 제공 형식 별칭을 제공하지 않습니다. 그러나 C# 지시문, 클래스 및 상속을 사용하여 사용자 정의 유형을 구성할 수 있습니다.
이 자습서에서는 C#에서 형식 별칭을 만들고 정의하는 네 가지 기본 방법을 배웁니다.
유형 별칭을 생성하기 위해 상속을 사용하는 것과 같이 각 방법에는 제한이 있습니다. 기본 유형에는 작동하지 않습니다. 결과적으로 봉인된 클래스에서 파생할 수 없으며 대부분의 .NET
클래스는 봉인되어 있습니다.
using alias
지시문을 사용하여 C#
에서 유형 별칭 정의
using
키워드는 C# 또는 .NET의 유형 별칭 관련 기능의 핵심입니다. 프로그래머에게 앨리어싱 속성을 활용하고, 코드를 유연하게 만들고, 가독성을 높이는 방법을 설명합니다.
객체의 범위를 자유롭게 정의할 수 있는 기능은 매우 효율적입니다. 네임스페이스에 대한 별칭을 만드는 데 중요한 부분입니다. 프로그래머가 정적 지시문을 사용하여 단일 클래스의 멤버를 가져오거나 다른 네임스페이스에서 특정 유형을 가져올 수 있습니다.
가장 중요한 점은 유형 별칭이 전체 네임스페이스를 가져오는 것을 방지하여 모호성을 해결하고 여러 유형으로 작업하기 쉽게 만든다는 것입니다. 또한 using alias
를 사용하면 C# 프로젝트에서 작동하는 전역 별칭을 만들 수 있습니다. 매개변수 유형을 전달하면 일반 유형의 별칭을 지정할 수 있습니다(예: using type_alias = System.IO.StreamWriter
및 유형의 일반 별칭:
using type_generic = System.Nullable<System.Int32>
예제 코드:
using System;
using System.Windows.Forms;
using stream_writer = System.IO.StreamWriter; // custom type
namespace type_alias {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
string[] type_data = { "Name: Widow's Sorrow", "Assignment: APK-1229-C", "Success Ratio: 78%",
"Charisma: 11%", "Style: Professional" };
using (stream_writer type_alias = new stream_writer(@"C:\Temp\MyFile.txt")) {
foreach (string line in type_data) {
type_alias.WriteLine(line);
// for compile purposes; MessageBox.Show(line);
}
MessageBox.Show("Process completed!");
}
}
}
}
출력:
Name: Widow's Sorrow
Assignment: APK-1229-C
Success Ratio: 78%
Charisma: 11%
Style: Professional
typedef
를 사용하는 것은 유형 별칭을 만드는 또 다른 방법이지만 프로세스가 매우 복잡해집니다. 단순화하려면 Curiously Recurring Template Pattern을 사용해야 합니다.
lambda 구문
및 typedef
를 비롯한 복잡한 상황 기반 메서드를 무시하고 Visual Studio에서 GenericHandler EventHandler<EventData>
또는 gcInt.MyEvent +=
를 사용하여 대리자 형식을 정의할 수 있습니다. IntelliSense
이벤트 처리 서명.
// you can define a type alias for specific types as follows:
using stream_writer = System.IO.StreamWriter // create stream writer object
using file_stream = System.IO.FileStream // create file stream object
// you can define generic alias for a type as follows:
using alias_int = System.Nullable<System.Int32>;
using alias_double = System.Nullable<System.Double>;
C#은 using Console = System.Console;
과 같은 항목에 더 짧은 이름을 도입하는 방법을 제공합니다. 이러한 바로 가기에는 자체 ID가 없으며 별칭이라고 할 수 있으므로 기존 유형을 다른 이름으로 사용할 수 있습니다.
별칭은 새 유형을 도입하지 않으므로 디버거에서 원래 이름으로 데코레이트된 기호를 볼 수 있습니다. 이는 혼란스러울 수 있지만 효율적이고 효율적입니다.
using
지시문은 typedef
와 완벽하게 동일합니다. 그러나 유형을 열린 상태로 두는 것은 매우 해롭기 때문에 성공적인 작업 후에 일반 유형을 닫을 수 있도록 제네릭 유형에 대한 별칭을 만드는 것이 중요합니다. using NullableThing<T> = System.Nullable<T>;
과 같은 것을 시도하기 전에 using
지시문 내에서 유형 매개변수를 확인하는 것이 중요합니다.
namespace
키워드를 사용하여 C#
에서 유형 별칭 정의
네임스페이스는 클래스를 사용하므로 유형 별칭을 정의하는 완벽한 방법입니다. 유형 또는 네임스페이스 별칭은 using Project = style.custom.myproject;
와 같이 using alias
지시문이라고 합니다.
네임스페이스는 사용자 정의 또는 시스템 정의일 수 있으며 using
지시문은 지정한 네임스페이스에 중첩된 네임스페이스에 대한 액세스 권한을 부여하지 않습니다. 네임스페이스를 사용하면 using example = Innerclass;
와 같이 public에서 유형 별칭을 정의하여 클래스의 전용 멤버를 쉽게 사용할 수 있습니다. Outerclass::example inner;
와 같이 개요를 선언합니다.
예제 코드:
using System;
using System.Windows.Forms;
using class_alias = type_newClass.custom_class;
using typealias_using = namespace_alias.custom_class<int>;
namespace type_newClass {
public class custom_class {
public override string ToString() {
return "You are in type_newClass.custom_class.";
}
}
}
namespace namespace_alias {
class custom_class<T> {
public override string ToString() {
return "You are in namespace_alias.custom_class.";
}
}
}
namespace type_alias {
using type_newClass; // use directive for namespace `type_newClass`
using namespace_alias; // use the directive for namespace `namespace_alias`
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
class_alias inst_typealias_one = new class_alias();
MessageBox.Show(inst_typealias_one.ToString());
typealias_using inst_typealias_two = new typealias_using();
MessageBox.Show(inst_typealias_two.ToString());
}
}
}
출력:
You are in type_newClass.custom_class.
상속을 사용하여 C#
에서 유형 별칭 정의
상속을 사용하는 것은 유사한 개념입니다. 예를 들어 class ComplexList : List<Tuple<int, string, int>> {}
와 네임스페이스
앨리어싱은 충돌 없는 C# 코드를 위한 방법입니다. 상속은 다른 유형의 인스턴스인 새 유형을 만들기 위한 옵션입니다.
상속은 하위 클래스의 기능을 사용하는 이점이 있지만 다른 경우에는 오버헤드, 간접 참조, 속도 저하 및 불안정성이 추가됩니다. 상속은 앨리어싱이 아니지만 일부 조건부 경우에 유사한 결과를 제공할 수 있습니다.
이름 앨리어싱 접근 방식은 모든 곳에서 이름을 재정의해야 하므로 코드 가독성이 떨어집니다. 다음과 같이 기본 클래스에 일부 기능을 추가하는 경우 상속이 더 나은 옵션입니다.
public class SoldierList : System.Collections.Generic.List<Soldier> {...}
또한 IEnumerable
에서 상속하면 열거 가능한 작업에서 클래스를 사용할 수 있으며 모든 클래스 동작을 완전히 정의할 수 있습니다.
예제 코드:
using System;
using System.Windows.Forms;
using System.Collections.Generic;
namespace type_alias {
// a simple approach
class ComplexList : List<Tuple<int, string, int>> {}
// more complex approach - enhances its usability
public class Person {
public string Name { get; set; }
public int Age { get; set; }
public int FavoriteNumber { get; set; }
public Person() {}
public Person(string name, int age, int favoriteNumber) {
this.Name = name;
this.Age = age;
this.FavoriteNumber = favoriteNumber;
}
}
public class PersonList : List<Person> {} // most-refined
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
var complexList = new ComplexList(); // for first approach
var personList = new PersonList(); // for second approach
/*
type your code or program something based on the
new types you created
*/
MessageBox.Show(
"Some function is performed successfully by creating a type alias using inheritance!",
"Type Alias", MessageBoxButtons.OKCancel, MessageBoxIcon.Warning);
}
}
}
출력:
Some function is performed successfully by creating a type alias using inheritance!
NuGet
패키지를 사용하여 C#
에서 유형 별칭 정의
일반적으로 헤더 파일에서 전역 및 클래스 멤버로 선언할 수 있으므로 유형 별칭은 단일 소스 코드 파일 또는 네임스페이스 블록으로 제한되지 않습니다. 유형의 장황함을 피하기 위해 특히 HashMap<TKey, TValue>
와 같은 일반 코드를 사용할 때 함수에서 간결한 이름을 선언할 수 있습니다.
이러한 별칭은 한 번만 만들어 전체 프로젝트에서 공유할 수 있으며 해당 템플릿은 더 나아가 구체적인 형식으로 확인되지 않는 별칭을 만들 수 있습니다.
NuGet
패키지를 사용하여 별칭을 입력하면 많은 코드 중복을 방지하고 매우 일반적인 HashMap<TKey, TValue>
와 매우 구체적인 LocationMap
사이에 있는 map
과 같은 중간 단계에 이름을 지정할 수 있습니다.
LikeType
은 C#에서 유형 별칭에 활용할 수 있는 오픈 소스 라이브러리 및 NuGet
패키지로 GenericClass<int>
동작을 제공합니다. 가장 중요한 것은 using Kleinware.LikeType;
때문에 Visual Studio의 C# 프로젝트에 Kleinware.LikeType 3.00
NuGet 패키지를 추가하는 것을 잊지 마십시오. 이 패키지에 속하며 C# 프로젝트에 일종의 typedef
기능을 제공합니다.
예제 코드:
// install `Kleinware.LikeType 3.00` NuGet Package before the program's execution
using System;
using System.Windows.Forms;
using System.Collections.Generic;
using Kleinware.LikeType; // belongs to the `Kleinware.LikeType 3.00`
namespace type_alias {
// type_alias creation
public class SomeInt : LikeType<int> // inherits the integer properties
{
public SomeInt(int value) : base(value) {} // represents the base value
}
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
/*
some example C# code that benefits from `Kleinware.LikeType 3.00`
*/
MessageBox.Show("Successful! Requires self-obervation.", "Type Alias",
MessageBoxButtons.OKCancel, MessageBoxIcon.Information);
}
}
}
출력:
Successful! Requires self-observation.
이 자습서에서는 C#에서 최적화된 다양한 별칭 지정 방법을 설명했습니다. 이러한 접근 방식을 사용하여 보다 강력하면서도 깔끔한 C# 애플리케이션을 구축할 수 있습니다.
유형 별칭의 핵심 사용 사례와 응용 프로그램에 어떤 이점이 있는지 보여주는 네 가지 기술 예제에서 많은 것을 배울 수 있습니다.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub