How to Query User Information From Active Directory in C#
-
Use
System.DirectoryServices
Namespace in C# to Query User Information From Active Directory -
Use
System.DirectoryServices.AccountManagement
Namespace in C# to Query User Information From Active Directory -
Combine
System.DirectoryServices
andSystem.DirectoryServices.AccountManagement
to Access Properties Not Exposed by theAccountManagement
Libraries
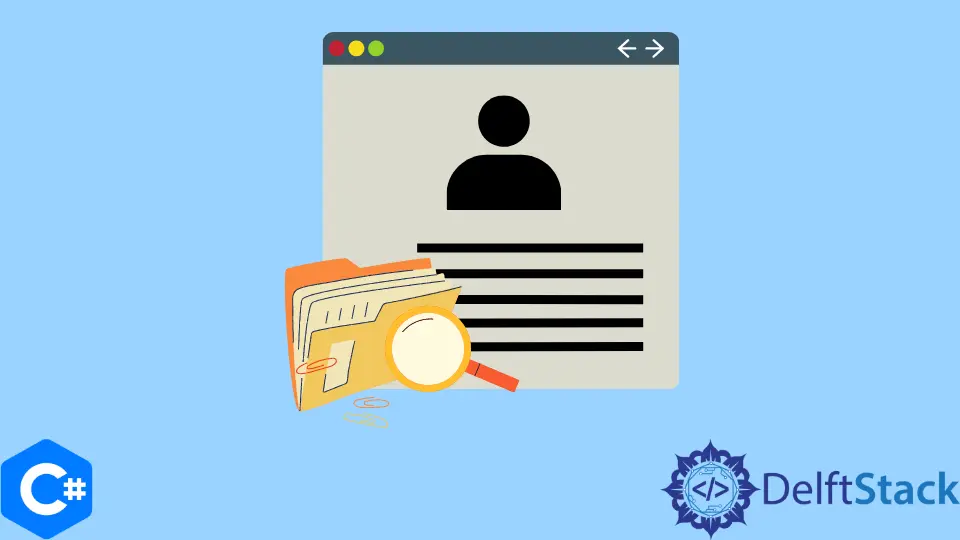
Retrieving the user information from Active Directory can be challenging as some of the command line and MSAD tools are quite limiting and difficult to use. On the other hand, writing your C# code is more rewarding and customizable.
There are two sets of classes for AD operations to query information in C#. The first one uses the System.DirectoryServices
namespace and is easier to use.
However, it’s not as versatile as the second one that features the System.DirectoryServices.AccountManagement
namespace.
Use System.DirectoryServices
Namespace in C# to Query User Information From Active Directory
System.DirectoryServices
is a technical way to let you access any user information from Active Directory. The properties of AD
objects (as they are all generic objects) are held in an array that contains all the user information.
This method is much more cryptic and detailed-oriented. It often requires UAC
codes to be manually set by developers to query the user information from Active Directory.
The following executable code will show you how to use Active Directory to query user information in C#.
using System;
using System.Text;
using System.DirectoryServices;
namespace queryInfoAD {
class userActiveDirectory {
static void Main(string[] args) {
// Input the user name (password can also be required) to get a particular user info from
// Active Directory
Console.Write("User Name = ");
String searchUsername = Console.ReadLine();
try {
// create an LDAP connection object that will help you fetch the Active Directory user
DirectoryEntry userLdapConn = createDirectoryEntry();
// create `ObjSearch` as a search object which operates on an LDAP connection object to only
// find a single user's details
DirectorySearcher ObjSearch = new DirectorySearcher(userLdapConn);
ObjSearch.Filter = searchUsername;
// create result objects from the search object
SearchResult checkUser = ObjSearch.FindOne();
if (checkUser != null) {
ResultPropertyCollection fields = checkUser.Properties;
foreach (String ldapUser in fields.PropertyNames) {
foreach (Object InfoChart in fields[ldapUser])
Console.WriteLine(String.Format("{0,-20} : {1}", ldapUser, InfoChart.ToString()));
}
}
else {
Console.WriteLine("User does not exist!");
}
}
catch (Exception e) {
Console.WriteLine("Error!\n\n" + e.ToString());
}
}
static DirectoryEntry createDirectoryEntry() {
// create an LDAP connection with custom settings and return it
DirectoryEntry ldapConnection = new DirectoryEntry("Insert connection domain link...");
ldapConnection.Path =
"Insert the path to the Active Directory which contains user information";
ldapConnection.AuthenticationType = AuthenticationTypes.Secure;
return ldapConnection;
}
}
}
System.DirectoryServices
provides easy access to the active directory from managed code. The search
object from DirectorySearcher
helps narrow the search to only one user with a common user name.
You can further narrow down your LDAP query by introducing a dSearcher.Filter
method, which can search a particular user for interesting information. Furthermore, don’t forget to dispose of the DirectoryEntry
and DirectorySearcher
objects as they belong to the System.DirectoryServices
namespace.
Use System.DirectoryServices.AccountManagement
Namespace in C# to Query User Information From Active Directory
Its primary purpose is to manage the Active Directory through .NET much easier. It is the newer approach to retrieving user information from the active directory and can perform pretty much every AD operation.
This method allows the developers to use commands like user.DisplayName
and user.VoiceTelephoneNumber
, which helps query a customized set of user information from Active Directory.
using System;
using System.DirectoryServices;
using System.DirectoryServices.AccountManagement;
// add the reference in the `System.DirectoryServices.AccountManagement` library to your project
// before executing this code
namespace DSAM_examp {
class AccManagement {
static void Main(string[] args) {
try {
// enter Active Directory settings
PrincipalContext ActiveDirectoryUser =
new PrincipalContext(ContextType.Domain, "Insert the domain link here...");
// create search user and add criteria
Console.Write("Enter User Name: ");
UserPrincipal userName = new UserPrincipal(ActiveDirectoryUser);
userName.SamAccountName = Console.ReadLine();
PrincipalSearcher search = new PrincipalSearcher(userName);
foreach (UserPrincipal result in search.FindAll())
// the user information in AD contains the VoiceTelephoneNumber that is unique and helps
// find the target user
// result.VoiceTelephoneNumber != null is also valid
if (result.DisplayName != null) {
// to display the user name and telephone number from Active Directory. You can also
// retrieve more information using similar commands.
Console.WriteLine(result.DisplayName, result.VoiceTelephoneNumber);
}
search.Dispose();
}
catch (Exception error) {
Console.WriteLine("User not found! Error: " + error.Message);
}
}
}
}
You can use UserPrincipal.FindByIdentity
as a class of the System.DirectoryServices.AccountManagement
namespace which is the simplest way to query user information but also the slowest.
By removing the SamAccountName
method and manual user name input, you can query the information of all users in the Active Directory.
Combine System.DirectoryServices
and System.DirectoryServices.AccountManagement
to Access Properties Not Exposed by the AccountManagement
Libraries
Properties that the AccountManagement
libraries do not expose can be accessed by converting UserPrincipal
into a more general object.
When retrieving underlying objects from the UserPrincipal
in Active Directory, both DirectoryServices
namespaces play an important part.
using System;
using System.DirectoryServices;
using System.DirectoryServices.AccountManagement;
namespace HybridMethod {
class getUserInfo {
static void Main(string[] args) {
try {
PrincipalContext userActiveDirectory =
new PrincipalContext(ContextType.Domain, "Insert your domain link here...");
// `UserPrincipal` class encapsulates principals that contain user information
UserPrincipal userName = new UserPrincipal(userActiveDirectory);
// retrieves the principal context that is essential to perform the query. Its context
// specifies the server/domain against which search operations are performed.
PrincipalSearcher searchObj = new PrincipalSearcher(userName);
foreach (UserPrincipal result in searchObj.FindAll()) {
if (result.DisplayName != null) {
// access the underlying object, e.g., user properties
DirectoryEntry lowerLdap = (DirectoryEntry)result.GetUnderlyingObject();
Console.WriteLine("{0,30} {1} {2}", result.DisplayName,
lowerLdap.Properties["postofficebox"][0].ToString());
}
}
// disposing the `PrincipalSearcher` object
searchObj.Dispose();
}
catch (Exception exp) {
Console.WriteLine(exp.Message);
}
}
}
}
In this tutorial, you have learned three ways to query the user information from Active Directory in C#. With the third hybrid method, you can even get obscure user properties.
These methods offer you several advantages like readability and brevity and are extremely useful to get all or a portion of user information from Active Directory faster and easier.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub