C# で Active Directory からユーザー情報を照会する
-
C# で
System.DirectoryServices
名前空間を使用して、Active Directory からユーザー情報を照会する -
C# で
System.DirectoryServices.AccountManagement
名前空間を使用して、Active Directory からユーザー情報を照会する -
System.DirectoryServices
とSystem.DirectoryServices.AccountManagement
を組み合わせて、AccountManagement
ライブラリによって公開されていないプロパティにアクセスする
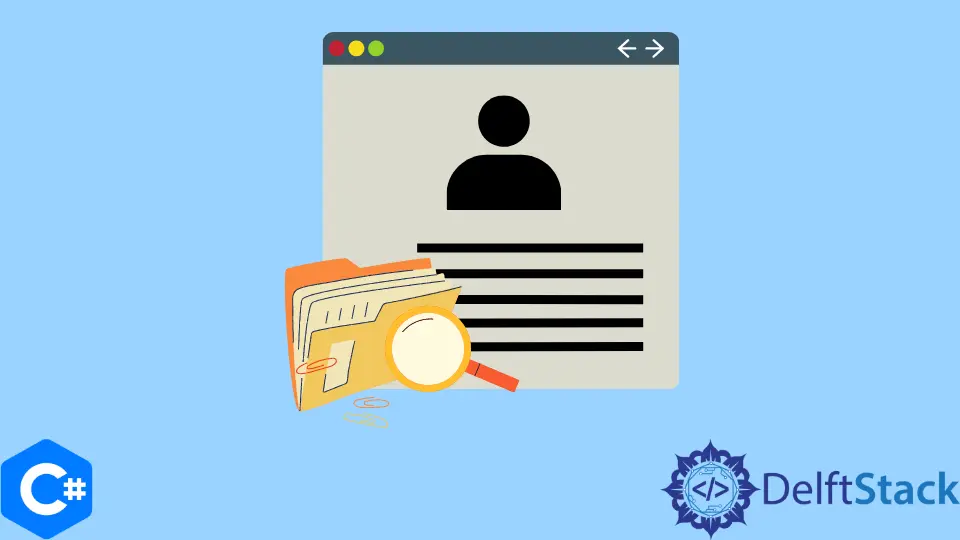
一部のコマンド ラインおよび MSAD ツールは非常に制限的で使いにくいため、Active Directory からユーザー情報を取得するのは困難な場合があります。 一方、C# コードを作成することは、やりがいがあり、カスタマイズ可能です。
C# で情報をクエリするための AD 操作には、2 セットのクラスがあります。 最初のものは System.DirectoryServices
名前空間を使用し、より使いやすくなっています。
ただし、System.DirectoryServices.AccountManagement
名前空間を備えた 2 番目のものほど用途が広くありません。
C# で System.DirectoryServices
名前空間を使用して、Active Directory からユーザー情報を照会する
System.DirectoryServices
は、Active Directory から任意のユーザー情報にアクセスできるようにするための技術的な方法です。 AD
オブジェクトのプロパティ (それらはすべて汎用オブジェクトであるため) は、すべてのユーザー情報を含む配列に保持されます。
この方法は、はるかに難解で詳細志向です。 多くの場合、開発者が Active Directory からユーザー情報を照会するには、UAC
コードを手動で設定する必要があります。
次の実行可能コードは、Active Directory を使用して C# でユーザー情報を照会する方法を示しています。
using System;
using System.Text;
using System.DirectoryServices;
namespace queryInfoAD {
class userActiveDirectory {
static void Main(string[] args) {
// Input the user name (password can also be required) to get a particular user info from
// Active Directory
Console.Write("User Name = ");
String searchUsername = Console.ReadLine();
try {
// create an LDAP connection object that will help you fetch the Active Directory user
DirectoryEntry userLdapConn = createDirectoryEntry();
// create `ObjSearch` as a search object which operates on an LDAP connection object to only
// find a single user's details
DirectorySearcher ObjSearch = new DirectorySearcher(userLdapConn);
ObjSearch.Filter = searchUsername;
// create result objects from the search object
SearchResult checkUser = ObjSearch.FindOne();
if (checkUser != null) {
ResultPropertyCollection fields = checkUser.Properties;
foreach (String ldapUser in fields.PropertyNames) {
foreach (Object InfoChart in fields[ldapUser])
Console.WriteLine(String.Format("{0,-20} : {1}", ldapUser, InfoChart.ToString()));
}
}
else {
Console.WriteLine("User does not exist!");
}
}
catch (Exception e) {
Console.WriteLine("Error!\n\n" + e.ToString());
}
}
static DirectoryEntry createDirectoryEntry() {
// create an LDAP connection with custom settings and return it
DirectoryEntry ldapConnection = new DirectoryEntry("Insert connection domain link...");
ldapConnection.Path =
"Insert the path to the Active Directory which contains user information";
ldapConnection.AuthenticationType = AuthenticationTypes.Secure;
return ldapConnection;
}
}
}
System.DirectoryServices を使用すると、マネージ コードから Active Directory に簡単にアクセスできます。 DirectorySearcher
のsearch
オブジェクトは、共通のユーザー名を持つ 1 人のユーザーのみに検索を絞り込むのに役立ちます。
dSearcher.Filter
メソッドを導入することで、LDAP クエリをさらに絞り込むことができます。このメソッドは、特定のユーザーから興味深い情報を検索できます。 さらに、DirectoryEntry
および DirectorySearcher
オブジェクトは System.DirectoryServices
名前空間に属しているため、それらを破棄することを忘れないでください。
C# で System.DirectoryServices.AccountManagement
名前空間を使用して、Active Directory からユーザー情報を照会する
その主な目的は、.NET を介して Active Directory をより簡単に管理することです。 これは、Active Directory からユーザー情報を取得するための新しいアプローチであり、ほぼすべての AD 操作を実行できます。
このメソッドにより、開発者は user.DisplayName
や user.VoiceTelephoneNumber
などのコマンドを使用して、Active Directory からカスタマイズされた一連のユーザー情報を照会できます。
using System;
using System.DirectoryServices;
using System.DirectoryServices.AccountManagement;
// add the reference in the `System.DirectoryServices.AccountManagement` library to your project
// before executing this code
namespace DSAM_examp {
class AccManagement {
static void Main(string[] args) {
try {
// enter Active Directory settings
PrincipalContext ActiveDirectoryUser =
new PrincipalContext(ContextType.Domain, "Insert the domain link here...");
// create search user and add criteria
Console.Write("Enter User Name: ");
UserPrincipal userName = new UserPrincipal(ActiveDirectoryUser);
userName.SamAccountName = Console.ReadLine();
PrincipalSearcher search = new PrincipalSearcher(userName);
foreach (UserPrincipal result in search.FindAll())
// the user information in AD contains the VoiceTelephoneNumber that is unique and helps
// find the target user
// result.VoiceTelephoneNumber != null is also valid
if (result.DisplayName != null) {
// to display the user name and telephone number from Active Directory. You can also
// retrieve more information using similar commands.
Console.WriteLine(result.DisplayName, result.VoiceTelephoneNumber);
}
search.Dispose();
}
catch (Exception error) {
Console.WriteLine("User not found! Error: " + error.Message);
}
}
}
}
UserPrincipal.FindByIdentity
を System.DirectoryServices.AccountManagement
名前空間のクラスとして使用できます。これは、ユーザー情報をクエリする最も簡単な方法ですが、最も遅い方法でもあります。
SamAccountName
メソッドとユーザー名の手動入力を削除することで、Active Directory 内のすべてのユーザーの情報を照会できます。
System.DirectoryServices
と System.DirectoryServices.AccountManagement
を組み合わせて、AccountManagement
ライブラリによって公開されていないプロパティにアクセスする
AccountManagement
ライブラリが公開していないプロパティは、UserPrincipal
をより一般的なオブジェクトに変換することでアクセスできます。
基になるオブジェクトを Active Directory の UserPrincipal
から取得する場合、両方の DirectoryServices
名前空間が重要な役割を果たします。
using System;
using System.DirectoryServices;
using System.DirectoryServices.AccountManagement;
namespace HybridMethod {
class getUserInfo {
static void Main(string[] args) {
try {
PrincipalContext userActiveDirectory =
new PrincipalContext(ContextType.Domain, "Insert your domain link here...");
// `UserPrincipal` class encapsulates principals that contain user information
UserPrincipal userName = new UserPrincipal(userActiveDirectory);
// retrieves the principal context that is essential to perform the query. Its context
// specifies the server/domain against which search operations are performed.
PrincipalSearcher searchObj = new PrincipalSearcher(userName);
foreach (UserPrincipal result in searchObj.FindAll()) {
if (result.DisplayName != null) {
// access the underlying object, e.g., user properties
DirectoryEntry lowerLdap = (DirectoryEntry)result.GetUnderlyingObject();
Console.WriteLine("{0,30} {1} {2}", result.DisplayName,
lowerLdap.Properties["postofficebox"][0].ToString());
}
}
// disposing the `PrincipalSearcher` object
searchObj.Dispose();
}
catch (Exception exp) {
Console.WriteLine(exp.Message);
}
}
}
}
このチュートリアルでは、C# で Active Directory からユーザー情報を照会する 3つの方法を学習しました。 3 番目のハイブリッド メソッドを使用すると、あいまいなユーザー プロパティを取得することもできます。
これらの方法には、読みやすさや簡潔さなどのいくつかの利点があり、Active Directory からユーザー情報のすべてまたは一部をより迅速かつ簡単に取得するのに非常に役立ちます。
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub