C#의 Active Directory에서 사용자 정보 쿼리
-
C#에서
System.DirectoryServices
네임스페이스를 사용하여 Active Directory에서 사용자 정보 쿼리 -
C#에서
System.DirectoryServices.AccountManagement
네임스페이스를 사용하여 Active Directory에서 사용자 정보 쿼리 -
System.DirectoryServices
및System.DirectoryServices.AccountManagement
를 결합하여AccountManagement
라이브러리에 의해 노출되지 않는 속성에 액세스
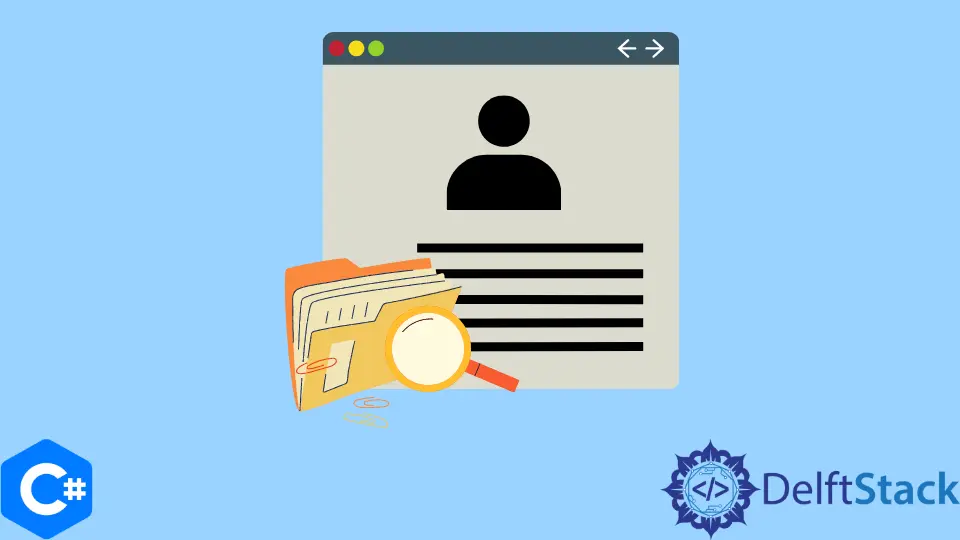
일부 명령줄 및 MSAD 도구는 상당히 제한적이고 사용하기 어렵기 때문에 Active Directory에서 사용자 정보를 검색하는 것은 어려울 수 있습니다. 반면에 C# 코드를 작성하는 것은 더 보람 있고 사용자 지정이 가능합니다.
C#에서 정보를 쿼리하는 AD 작업에는 두 가지 클래스 집합이 있습니다. 첫 번째는 System.DirectoryServices
네임스페이스를 사용하며 사용하기 더 쉽습니다.
그러나 System.DirectoryServices.AccountManagement
네임스페이스를 특징으로 하는 두 번째 항목만큼 다재다능하지는 않습니다.
C#에서 System.DirectoryServices
네임스페이스를 사용하여 Active Directory에서 사용자 정보 쿼리
‘System.DirectoryServices’는 Active Directory에서 사용자 정보에 액세스할 수 있는 기술적인 방법입니다. AD
개체의 속성(모두 일반 개체이므로)은 모든 사용자 정보를 포함하는 배열에 보관됩니다.
이 방법은 훨씬 더 비밀스럽고 세부 지향적입니다. 종종 Active Directory에서 사용자 정보를 쿼리하기 위해 개발자가 수동으로 설정해야 하는 UAC
코드가 필요합니다.
다음 실행 코드는 Active Directory를 사용하여 C#에서 사용자 정보를 쿼리하는 방법을 보여줍니다.
using System;
using System.Text;
using System.DirectoryServices;
namespace queryInfoAD {
class userActiveDirectory {
static void Main(string[] args) {
// Input the user name (password can also be required) to get a particular user info from
// Active Directory
Console.Write("User Name = ");
String searchUsername = Console.ReadLine();
try {
// create an LDAP connection object that will help you fetch the Active Directory user
DirectoryEntry userLdapConn = createDirectoryEntry();
// create `ObjSearch` as a search object which operates on an LDAP connection object to only
// find a single user's details
DirectorySearcher ObjSearch = new DirectorySearcher(userLdapConn);
ObjSearch.Filter = searchUsername;
// create result objects from the search object
SearchResult checkUser = ObjSearch.FindOne();
if (checkUser != null) {
ResultPropertyCollection fields = checkUser.Properties;
foreach (String ldapUser in fields.PropertyNames) {
foreach (Object InfoChart in fields[ldapUser])
Console.WriteLine(String.Format("{0,-20} : {1}", ldapUser, InfoChart.ToString()));
}
}
else {
Console.WriteLine("User does not exist!");
}
}
catch (Exception e) {
Console.WriteLine("Error!\n\n" + e.ToString());
}
}
static DirectoryEntry createDirectoryEntry() {
// create an LDAP connection with custom settings and return it
DirectoryEntry ldapConnection = new DirectoryEntry("Insert connection domain link...");
ldapConnection.Path =
"Insert the path to the Active Directory which contains user information";
ldapConnection.AuthenticationType = AuthenticationTypes.Secure;
return ldapConnection;
}
}
}
System.DirectoryServices
는 관리 코드에서 활성 디렉터리에 쉽게 액세스할 수 있도록 합니다. DirectorySearcher
의 search
객체는 공통 사용자 이름을 가진 단 한 명의 사용자로 검색 범위를 좁히는 데 도움이 됩니다.
흥미로운 정보에 대해 특정 사용자를 검색할 수 있는 dSearcher.Filter
메서드를 도입하여 LDAP 쿼리를 더 좁힐 수 있습니다. 또한 DirectoryEntry
및 DirectorySearcher
개체는 System.DirectoryServices
네임스페이스에 속하므로 폐기하는 것을 잊지 마십시오.
C#에서 System.DirectoryServices.AccountManagement
네임스페이스를 사용하여 Active Directory에서 사용자 정보 쿼리
주요 목적은 .NET을 통해 Active Directory를 훨씬 쉽게 관리하는 것입니다. 활성 디렉터리에서 사용자 정보를 검색하는 새로운 접근 방식이며 거의 모든 AD 작업을 수행할 수 있습니다.
이 방법을 통해 개발자는 user.DisplayName
및 user.VoiceTelephoneNumber
와 같은 명령을 사용하여 Active Directory에서 사용자 정의된 사용자 정보 집합을 쿼리할 수 있습니다.
using System;
using System.DirectoryServices;
using System.DirectoryServices.AccountManagement;
// add the reference in the `System.DirectoryServices.AccountManagement` library to your project
// before executing this code
namespace DSAM_examp {
class AccManagement {
static void Main(string[] args) {
try {
// enter Active Directory settings
PrincipalContext ActiveDirectoryUser =
new PrincipalContext(ContextType.Domain, "Insert the domain link here...");
// create search user and add criteria
Console.Write("Enter User Name: ");
UserPrincipal userName = new UserPrincipal(ActiveDirectoryUser);
userName.SamAccountName = Console.ReadLine();
PrincipalSearcher search = new PrincipalSearcher(userName);
foreach (UserPrincipal result in search.FindAll())
// the user information in AD contains the VoiceTelephoneNumber that is unique and helps
// find the target user
// result.VoiceTelephoneNumber != null is also valid
if (result.DisplayName != null) {
// to display the user name and telephone number from Active Directory. You can also
// retrieve more information using similar commands.
Console.WriteLine(result.DisplayName, result.VoiceTelephoneNumber);
}
search.Dispose();
}
catch (Exception error) {
Console.WriteLine("User not found! Error: " + error.Message);
}
}
}
}
사용자 정보를 쿼리하는 가장 간단하지만 가장 느린 방법인 System.DirectoryServices.AccountManagement
네임스페이스의 클래스로 UserPrincipal.FindByIdentity
를 사용할 수 있습니다.
SamAccountName
메서드 및 수동 사용자 이름 입력을 제거하여 Active Directory의 모든 사용자 정보를 쿼리할 수 있습니다.
System.DirectoryServices
및 System.DirectoryServices.AccountManagement
를 결합하여 AccountManagement
라이브러리에 의해 노출되지 않는 속성에 액세스
AccountManagement
라이브러리가 노출하지 않는 속성은 UserPrincipal
을 보다 일반적인 개체로 변환하여 액세스할 수 있습니다.
Active Directory의 UserPrincipal
에서 기본 개체를 검색할 때 두 DirectoryServices
네임스페이스가 중요한 역할을 합니다.
using System;
using System.DirectoryServices;
using System.DirectoryServices.AccountManagement;
namespace HybridMethod {
class getUserInfo {
static void Main(string[] args) {
try {
PrincipalContext userActiveDirectory =
new PrincipalContext(ContextType.Domain, "Insert your domain link here...");
// `UserPrincipal` class encapsulates principals that contain user information
UserPrincipal userName = new UserPrincipal(userActiveDirectory);
// retrieves the principal context that is essential to perform the query. Its context
// specifies the server/domain against which search operations are performed.
PrincipalSearcher searchObj = new PrincipalSearcher(userName);
foreach (UserPrincipal result in searchObj.FindAll()) {
if (result.DisplayName != null) {
// access the underlying object, e.g., user properties
DirectoryEntry lowerLdap = (DirectoryEntry)result.GetUnderlyingObject();
Console.WriteLine("{0,30} {1} {2}", result.DisplayName,
lowerLdap.Properties["postofficebox"][0].ToString());
}
}
// disposing the `PrincipalSearcher` object
searchObj.Dispose();
}
catch (Exception exp) {
Console.WriteLine(exp.Message);
}
}
}
}
이 자습서에서는 C#의 Active Directory에서 사용자 정보를 쿼리하는 세 가지 방법을 배웠습니다. 세 번째 하이브리드 방법을 사용하면 모호한 사용자 속성을 얻을 수도 있습니다.
이러한 방법은 가독성 및 간결함과 같은 몇 가지 이점을 제공하며 Active Directory에서 사용자 정보의 전체 또는 일부를 빠르고 쉽게 가져오는 데 매우 유용합니다.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub