How to Open Folder Dialog in C#
-
Use the
FolderBrowserDialog
Class in C# to Open a Folder Dialog -
Use the
OpenFileDialog
Class in C# to Open a Folder Dialog
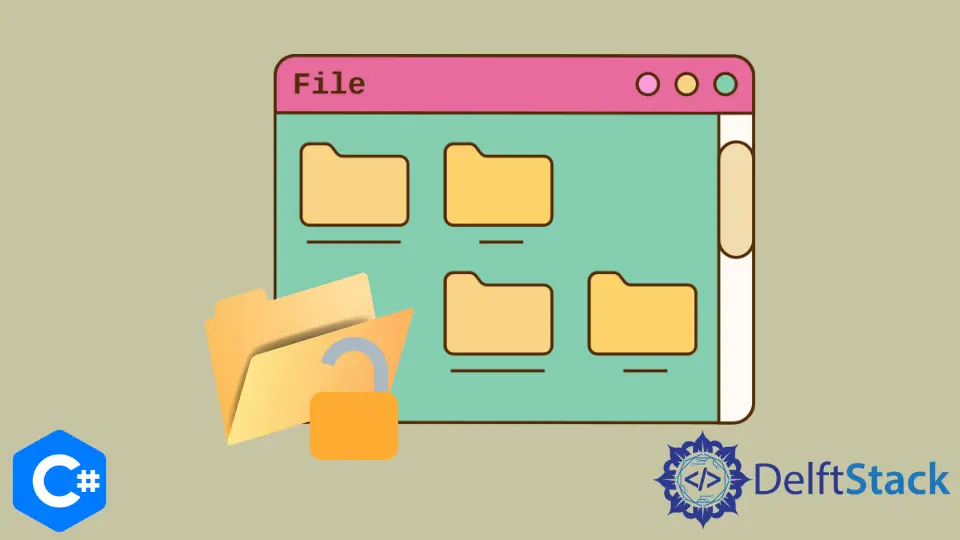
In C# applications, interaction with users on selecting files is a fundamental and compulsory topic. In this tutorial, you will learn the two different ways to open a folder dialog in C#.
It’s important to deal with Folder Browser and Open File Dialogs to select files and folders when developing C# projects in Winforms.
The folder dialogs are a part of the Windows API and are accessible to C# developers on the Windows platform. The first way is to use the OpenFileDialog
class to display a folder dialog for opening one or several files.
The other method is to use the FolderBrowserDialog
control to display a folder dialog for selecting folders from the same directory.
Use the FolderBrowserDialog
Class in C# to Open a Folder Dialog
This class serves to open a dialog folder to browse and select a folder on a computer. It has Windows Explorer-like features to navigate through folders and select a folder.
The FolderBrowserDialog
class does not have or need visual properties like others. It is a part of the .Net
framework and provides a folder browser component for your C# applications.
The following C# program in Visual Studio will open a folder dialog and output something when the user selects a folder and presses OK
. Create a Form1.cs [Design]
in Visual Studio and a button1
button before executing the C# code.
using System;
using System.Data;
using System.Linq;
using System.Drawing;
using System.Collections.Generic;
using System.Text;
using System.ComponentModel;
using System.IO;
using System.Windows.Forms;
namespace openFolderDialog {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
// `button1` button click event to open folder dialog
private void button1_Click(object sender, EventArgs e) {
FolderBrowserDialog fbd = new FolderBrowserDialog();
if (fbd.ShowDialog() == System.Windows.Forms.DialogResult.OK)
// shows the path to the selected folder in the folder dialog
MessageBox.Show(fbd.SelectedPath);
}
}
}
While FolderBrowserDialog
is a handy way to open folder dialog in C#, it suffers from many limitations, some preventing the component from being of practical use in applications.
It features powerful behavior control and customization functionality miles ahead of Windows Explorer.
Use the OpenFileDialog
Class in C# to Open a Folder Dialog
In C#, OpenFileDialog
control is the easiest to launch Windows Open File Dialog and let them select files in the same directory. The primary purpose of Open File Dialog is to select single or multiple files for different processes like uploading and downloading files in C#.
using System;
using System.Data;
using System.Linq;
using System.Drawing;
using System.Collections.Generic;
using System.Text;
using System.ComponentModel;
using System.IO;
using System.Windows.Forms;
namespace OpenFileDialogBox_CS {
// create `Form1.cs [Design]`in Visual Studio
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
// create a `btnSelect` button in Form1.cs [Design] and use its name in C# code
private void btnSelect_Click(object sender, EventArgs e) {
openFileDialog1.Multiselect = false;
if (openFileDialog1.ShowDialog() == DialogResult.OK) {
string fileName = Path.GetFileName(openFileDialog1.FileName);
string filePath = openFileDialog1.FileName;
MessageBox.Show(fileName + " - " + filePath);
}
}
// create a `btnSelectMultiple` button in Form1.cs [Design] and use its name in C# code
private void btnSelectMultiple_Click(object sender, EventArgs e) {
string message = "";
openFileDialog1.Multiselect = true;
if (openFileDialog1.ShowDialog() == DialogResult.OK) {
foreach (string file in openFileDialog1.FileNames) {
message += Path.GetFileName(file) + " - " + file + Environment.NewLine;
}
MessageBox.Show(message);
}
}
}
}
With OpenFileDialog
, it is possible to show only files with specific extensions using its Filter
property.
openFileDialog1.Filter = "Image Files|*.GIF;*.BMP;*.JPEG;*.PNG;*.JPG|All files (*.*)|*.*";
Use the following C# code to make the OpenFileDialog
class start a folder dialog in a specific folder.
openFileDialog1.InitialDirectory = "c:\\temp";
Both methods to open folder dialog in C# are simple and support a vast library of features and other elements to customize the open folder dialog according to your needs. Furthermore, both methods enable you to fully customize the open folder dialog elements and their properties.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub