在 C# 中打开文件夹对话框
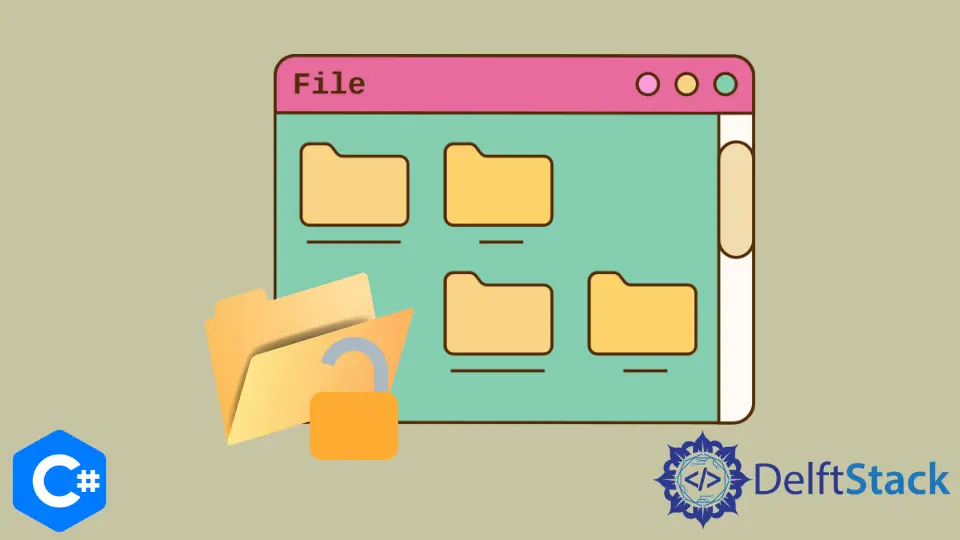
在 C# 应用程序中,在选择文件时与用户进行交互是一个基本且必修的话题。在本教程中,你将学习在 C# 中打开文件夹对话框的两种不同方法。
在 Winforms 中开发 C# 项目时,处理文件夹浏览器和打开文件对话框以选择文件和文件夹很重要。
文件夹对话框是 Windows API 的一部分,可供 Windows 平台上的 C# 开发人员访问。第一种方法是使用 OpenFileDialog
类来显示用于打开一个或多个文件的文件夹对话框。
另一种方法是使用 FolderBrowserDialog
控件显示一个文件夹对话框,用于从同一目录中选择文件夹。
使用 C# 中的 FolderBrowserDialog
类打开文件夹对话框
此类用于打开对话框文件夹以浏览和选择计算机上的文件夹。它具有类似 Windows 资源管理器的功能,可以浏览文件夹并选择文件夹。
FolderBrowserDialog
类没有或不需要像其他的视觉属性。它是 .Net
框架的一部分,并为你的 C# 应用程序提供文件夹浏览器组件。
Visual Studio 中的以下 C# 程序将打开一个文件夹对话框并在用户选择一个文件夹并按下 OK
时输出一些内容。在执行 C# 代码之前,在 Visual Studio 中创建一个 Form1.cs [Design]
和一个 button1
按钮。
using System;
using System.Data;
using System.Linq;
using System.Drawing;
using System.Collections.Generic;
using System.Text;
using System.ComponentModel;
using System.IO;
using System.Windows.Forms;
namespace openFolderDialog {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
// `button1` button click event to open folder dialog
private void button1_Click(object sender, EventArgs e) {
FolderBrowserDialog fbd = new FolderBrowserDialog();
if (fbd.ShowDialog() == System.Windows.Forms.DialogResult.OK)
// shows the path to the selected folder in the folder dialog
MessageBox.Show(fbd.SelectedPath);
}
}
}
虽然 FolderBrowserDialog
是一种在 C# 中打开文件夹对话框的便捷方式,但它存在许多限制,有些限制了该组件在应用程序中的实际使用。
它具有强大的行为控制和自定义功能,远远领先于 Windows 资源管理器。
使用 C# 中的 OpenFileDialog
类打开文件夹对话框
在 C# 中,OpenFileDialog
控件是最容易启动 Windows 打开文件对话框并让它们选择同一目录中的文件的方法。打开文件对话框的主要目的是为不同的过程选择单个或多个文件,例如在 C# 中上传和下载文件。
using System;
using System.Data;
using System.Linq;
using System.Drawing;
using System.Collections.Generic;
using System.Text;
using System.ComponentModel;
using System.IO;
using System.Windows.Forms;
namespace OpenFileDialogBox_CS {
// create `Form1.cs [Design]`in Visual Studio
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
// create a `btnSelect` button in Form1.cs [Design] and use its name in C# code
private void btnSelect_Click(object sender, EventArgs e) {
openFileDialog1.Multiselect = false;
if (openFileDialog1.ShowDialog() == DialogResult.OK) {
string fileName = Path.GetFileName(openFileDialog1.FileName);
string filePath = openFileDialog1.FileName;
MessageBox.Show(fileName + " - " + filePath);
}
}
// create a `btnSelectMultiple` button in Form1.cs [Design] and use its name in C# code
private void btnSelectMultiple_Click(object sender, EventArgs e) {
string message = "";
openFileDialog1.Multiselect = true;
if (openFileDialog1.ShowDialog() == DialogResult.OK) {
foreach (string file in openFileDialog1.FileNames) {
message += Path.GetFileName(file) + " - " + file + Environment.NewLine;
}
MessageBox.Show(message);
}
}
}
}
使用 OpenFileDialog
,可以使用其 Filter
属性仅显示具有特定扩展名的文件。
openFileDialog1.Filter = "Image Files|*.GIF;*.BMP;*.JPEG;*.PNG;*.JPG|All files (*.*)|*.*";
使用以下 C# 代码使 OpenFileDialog
类在特定文件夹中启动文件夹对话框。
openFileDialog1.InitialDirectory = "c:\\temp";
在 C# 中打开文件夹对话框的两种方法都很简单,并且支持大量功能库和其他元素,可以根据你的需要自定义打开文件夹对话框。此外,这两种方法都使你能够完全自定义打开的文件夹对话框元素及其属性。
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub