C# でフォルダダイアログを開く
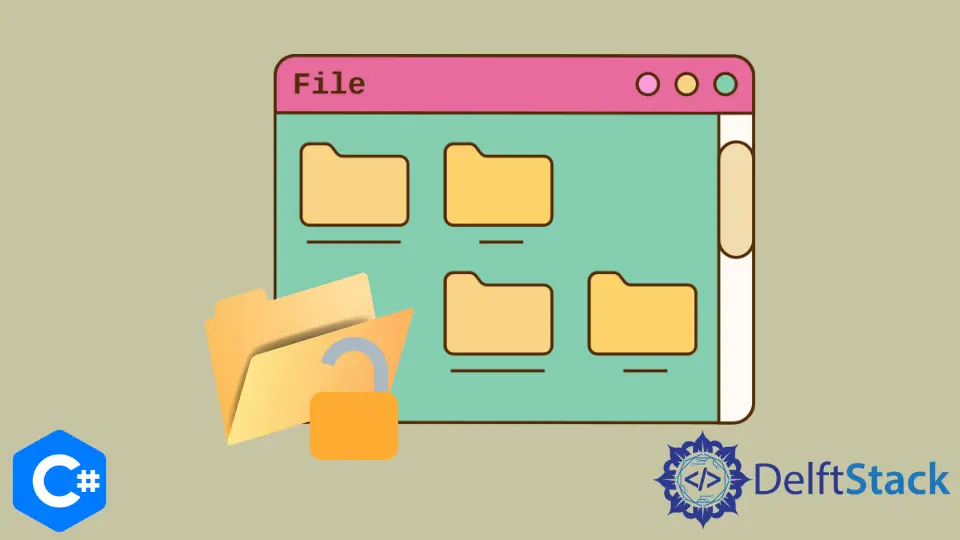
C# アプリケーションでは、ファイルの選択に関するユーザーとの対話は、基本的かつ必須のトピックです。このチュートリアルでは、C# でフォルダダイアログを開く 2つの異なる方法を学習します。
Winforms で C# プロジェクトを開発するときは、フォルダブラウザとファイルダイアログを開いてファイルとフォルダを選択することが重要です。
フォルダダイアログは WindowsAPI の一部であり、Windows プラットフォームの C# 開発者がアクセスできます。最初の方法は、OpenFileDialog
クラスを使用して、1つまたは複数のファイルを開くためのフォルダーダイアログを表示することです。
もう 1つの方法は、FolderBrowserDialog
コントロールを使用して、同じディレクトリからフォルダを選択するためのフォルダダイアログを表示することです。
C# の FolderBrowserDialog
クラスを使用してフォルダダイアログを開く
このクラスは、コンピューター上のフォルダーを参照および選択するためのダイアログフォルダーを開くのに役立ちます。フォルダ間を移動してフォルダを選択するための Windows エクスプローラのような機能があります。
FolderBrowserDialog
クラスには、他のクラスのような視覚的プロパティがないか、必要ありません。これは .Net
フレームワークの一部であり、C# アプリケーション用のフォルダーブラウザコンポーネントを提供します。
Visual Studio の次の C# プログラムは、ユーザーがフォルダーを選択して OK
を押すと、フォルダーダイアログを開き、何かを出力します。C# コードを実行する前に、VisualStudio で Form1.cs [Design]
と button1
ボタンを作成します。
using System;
using System.Data;
using System.Linq;
using System.Drawing;
using System.Collections.Generic;
using System.Text;
using System.ComponentModel;
using System.IO;
using System.Windows.Forms;
namespace openFolderDialog {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
// `button1` button click event to open folder dialog
private void button1_Click(object sender, EventArgs e) {
FolderBrowserDialog fbd = new FolderBrowserDialog();
if (fbd.ShowDialog() == System.Windows.Forms.DialogResult.OK)
// shows the path to the selected folder in the folder dialog
MessageBox.Show(fbd.SelectedPath);
}
}
}
FolderBrowserDialog
は C# でフォルダダイアログを開くための便利な方法ですが、多くの制限があり、コンポーネントをアプリケーションで実際に使用できない場合があります。
これは、WindowsExplorer よりもはるかに優れた強力な動作制御およびカスタマイズ機能を備えています。
C# の OpenFileDialog
クラスを使用してフォルダダイアログを開く
C# では、OpenFileDialog
コントロールが Windows Open File Dialog を起動し、同じディレクトリ内のファイルを選択できるようにするのが最も簡単です。Open File Dialog の主な目的は、C# でのファイルのアップロードやダウンロードなど、さまざまなプロセスで単一または複数のファイルを選択することです。
using System;
using System.Data;
using System.Linq;
using System.Drawing;
using System.Collections.Generic;
using System.Text;
using System.ComponentModel;
using System.IO;
using System.Windows.Forms;
namespace OpenFileDialogBox_CS {
// create `Form1.cs [Design]`in Visual Studio
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
// create a `btnSelect` button in Form1.cs [Design] and use its name in C# code
private void btnSelect_Click(object sender, EventArgs e) {
openFileDialog1.Multiselect = false;
if (openFileDialog1.ShowDialog() == DialogResult.OK) {
string fileName = Path.GetFileName(openFileDialog1.FileName);
string filePath = openFileDialog1.FileName;
MessageBox.Show(fileName + " - " + filePath);
}
}
// create a `btnSelectMultiple` button in Form1.cs [Design] and use its name in C# code
private void btnSelectMultiple_Click(object sender, EventArgs e) {
string message = "";
openFileDialog1.Multiselect = true;
if (openFileDialog1.ShowDialog() == DialogResult.OK) {
foreach (string file in openFileDialog1.FileNames) {
message += Path.GetFileName(file) + " - " + file + Environment.NewLine;
}
MessageBox.Show(message);
}
}
}
}
OpenFileDialog
では、Filter
プロパティを使用して特定の拡張子を持つファイルのみを表示できます。
openFileDialog1.Filter = "Image Files|*.GIF;*.BMP;*.JPEG;*.PNG;*.JPG|All files (*.*)|*.*";
次の C# コードを使用して、OpenFileDialog
クラスが特定のフォルダーでフォルダーダイアログを開始するようにします。
openFileDialog1.InitialDirectory = "c:\\temp";
C# でフォルダダイアログを開く方法はどちらもシンプルで、必要に応じてフォルダダイアログを開くための機能やその他の要素の膨大なライブラリをサポートしています。さらに、どちらの方法でも、フォルダを開くダイアログ要素とそのプロパティを完全にカスタマイズできます。
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub