How to Get the Value of ListBox Selected Item in C#
-
Use the
ListBox.SelectedValue
Property to Get the Value of the ListBox Selected Item inC#
-
Use the
ListBox.SelectedItem
Property to Get the Value of the ListBox Selected Item inC#
-
Use the
GetItemText()
Method to Get Value of the ListBox Selected Item inC#
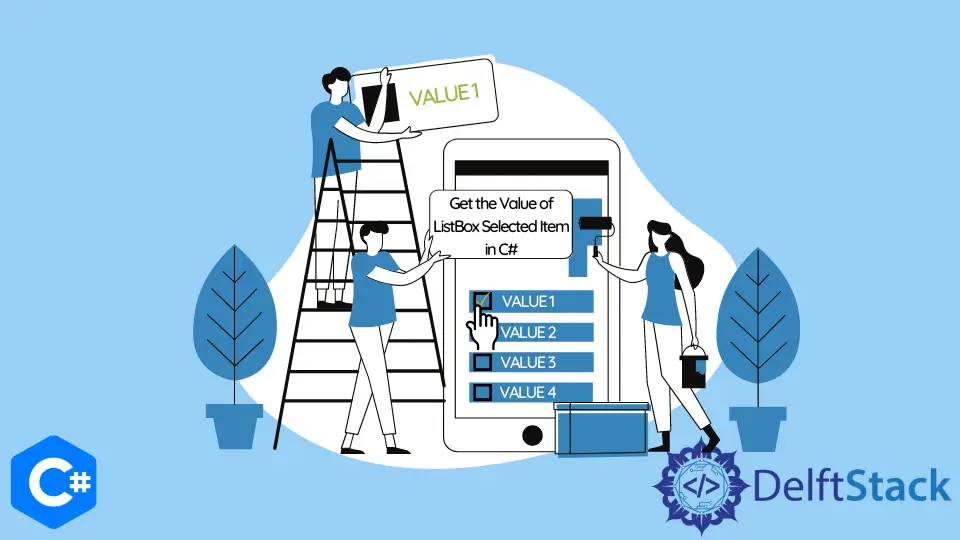
This article will teach you how to programmatically get the value of a ListBox control’s selected item. In C#, the ListBox control SelectionMode
property have two possible values Single
and Multiple
.
The Single
mode allows you to select a single item from the ListBox control. In general, there are multiple ways to get the value of the ListBox selected item in C#.
Use the ListBox.SelectedValue
Property to Get the Value of the ListBox Selected Item in C#
The SelectedValue
property is an alternative means of binding. It can get you the different values of a selected object or item in a ListBox.
The SelectedValuePath
property plays an important part in selecting a value of a ListBox. For example, if your ListBox is bound to a collection of category objects, each of which has the ID
, Name
, and Context
properties, the SelectedValuePath = Name
will cause the value of the selected Category’s Name
property to be returned in the SelectedValue
.
// create a `button1` button and a `listBox1` listbox in your C# project's `Form1`
using System;
using System.Windows.Forms;
namespace ListboxSelectedValue {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {
// add items and values to the `listBox1` listbox
listBox1.Items.Add(new ListItem { Name = "Item1", Value = 1 });
listBox1.Items.Add(new ListItem { Name = "Item2", Value = 2 });
listBox1.Items.Add(new ListItem { Name = "Item3", Value = 3 });
listBox1.Items.Add(new ListItem { Name = "Item4", Value = 4 });
}
// create a class to add values and text to the `listBox1` listbox
public class ListItem {
public string Name { get; set; }
public int Value { get; set; }
public override string ToString() {
return Name;
}
}
private void button1_Click(object sender, EventArgs e) {
// to get the value when a listBox1 item is selected
var value = ((ListItem)listBox1.SelectedItem).Value;
// to display the selected value
MessageBox.Show("The value of your selected item: " + value);
}
}
}
Output:
*select an item from the listbox item2*
*press button1*
The value of your selected item: 2
Use the ListBox.SelectedItem
Property to Get the Value of the ListBox Selected Item in C#
The ListBox.SelectedItem
property can get or set the value of a ListBox selected item. If you want to obtain the currently selected item’s index position and value in a ListBox, use the SelectedIndex
property.
In C#, the SelectedItem
property returns the entire object your ListBox is bound to or the items it contains. It only enables you to bind an entire object to the property that the ListBox is bound to, not the value of a single property on that object (such as an ID
property of a Category
object).
// create a `button1` button and a `listBox1` listbox with items
// use the following code in your C# form's `button1_Click` event
private void button1_Click(object sender, EventArgs e) {
// to retrive the object from listbox and store it into a string variable
string curItem = listBox1.SelectedItem.ToString();
// to show the value of selected item stored as a string
MessageBox.Show("Your selected item: " + curItem);
}
Output:
*select item3 from the listbox and press button1*
Your selected item: item3
Use the GetItemText()
Method to Get Value of the ListBox Selected Item in C#
The GetItemText
method in C# returns the textual representation of the selected item in a ListBox. The value returned by the GetItemText(Object)
is the value of the ToString
item’s method.
Create a Form1
in your C# project, which contains a listBox1
ListBox and a button1
button. Afterward, paste the following code in your button1_Click
event to retrieve the value of the selected item in a ListBox:
private void button1_Click(object sender, EventArgs e) {
// to get the value when a listBox1 item is selected
string text = listBox1.GetItemText(listBox1.SelectedItem);
// to display the selected value
MessageBox.Show("The value of your selected item: " + text);
}
Output:
*select item4 from the listbox and press button1*
The value of your selected item: item4
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub